Mbed OS 5
Fork of PinDetect by
Embed:
(wiki syntax)
Show/hide line numbers
PinDetect.h
00001 /* 00002 Copyright (c) 2010 Andy Kirkham 00003 00004 Permission is hereby granted, free of charge, to any person obtaining a copy 00005 of this software and associated documentation files (the "Software"), to deal 00006 in the Software without restriction, including without limitation the rights 00007 to use, copy, modify, merge, publish, distribute, sublicense, and/or sell 00008 copies of the Software, and to permit persons to whom the Software is 00009 furnished to do so, subject to the following conditions: 00010 00011 The above copyright notice and this permission notice shall be included in 00012 all copies or substantial portions of the Software. 00013 00014 THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR 00015 IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, 00016 FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE 00017 AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER 00018 LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, 00019 OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN 00020 THE SOFTWARE. 00021 */ 00022 00023 #ifndef AJK_PIN_DETECT_H 00024 #define AJK_PIN_DETECT_H 00025 00026 #ifndef MBED_H 00027 #include "mbed.h" 00028 #endif 00029 00030 #ifndef PINDETECT_PIN_ASSERTED 00031 #define PINDETECT_PIN_ASSERTED 1 00032 #endif 00033 00034 #ifndef PINDETECT_SAMPLE_PERIOD 00035 #define PINDETECT_SAMPLE_PERIOD 20000 00036 #endif 00037 00038 #ifndef PINDETECT_ASSERT_COUNT 00039 #define PINDETECT_ASSERT_COUNT 1 00040 #endif 00041 00042 #ifndef PINDETECT_HOLD_COUNT 00043 #define PINDETECT_HOLD_COUNT 50 00044 #endif 00045 00046 namespace AjK { 00047 00048 class PinDetect { 00049 public: 00050 friend class Ticker; 00051 00052 00053 /** PinDetect constructor 00054 * 00055 * By default the PinMode is set to PullDown. 00056 * 00057 * @see http://mbed.org/handbook/DigitalIn 00058 * @param p PinName is a valid pin that supports DigitalIn 00059 */ 00060 PinDetect(PinName p); 00061 00062 /** PinDetect constructor 00063 * 00064 * @see http://mbed.org/handbook/DigitalIn 00065 * @param PinName p is a valid pin that supports DigitalIn 00066 * @param PinMode m The mode the DigitalIn should use. 00067 */ 00068 PinDetect(PinName p, PinMode m); 00069 00070 /** PinDetect destructor 00071 */ 00072 virtual ~PinDetect(void); 00073 00074 /** Set the sampling time in microseconds. 00075 * 00076 * @param int The time between pin samples in microseconds. 00077 */ 00078 void setSampleFrequency(int i = PINDETECT_SAMPLE_PERIOD); 00079 00080 /** Set the value used as assert. 00081 * 00082 * Defaults to 1 (ie if pin == 1 then pin asserted). 00083 * 00084 * @param int New assert value (1 or 0) 00085 */ 00086 void setAssertValue(int i = PINDETECT_PIN_ASSERTED); 00087 00088 /** Set the number of continuous samples until assert assumed. 00089 * 00090 * Defaults to 1 (1 * sample frequency). 00091 * 00092 * @param int The number of continuous samples until assert assumed. 00093 */ 00094 void setSamplesTillAssert(int i); 00095 00096 /** Set the number of continuous samples until held assumed. 00097 * 00098 * Defaults to 50 * sample frequency. 00099 * 00100 * @param int The number of continuous samples until held assumed. 00101 */ 00102 void setSamplesTillHeld(int i); 00103 00104 /** Set the pin mode. 00105 * 00106 * @see http://mbed.org/projects/libraries/api/mbed/trunk/DigitalInOut#DigitalInOut.mode 00107 * @param PinMode m The mode to pass on to the DigitalIn 00108 */ 00109 void mode(PinMode m); 00110 00111 void attach_asserted(Callback<void()> function); 00112 00113 void attach_deasserted(Callback<void()> function); 00114 00115 void attach_asserted_held(Callback<void()> function); 00116 00117 void attach_deasserted_held(Callback<void()> function); 00118 00119 /** operator int() 00120 * 00121 * Read the value of the pin being sampled. 00122 */ 00123 operator int() { 00124 return _in->read(); 00125 } 00126 00127 protected: 00128 DigitalIn *_in; 00129 Ticker *_ticker; 00130 int _prevState; 00131 int _currentStateCounter; 00132 int _sampleTime; 00133 int _assertValue; 00134 int _samplesTillAssertReload; 00135 int _samplesTillAssert; 00136 int _samplesTillHeldReload; 00137 int _samplesTillHeld; 00138 Callback<void()> _callbackAsserted; 00139 Callback<void()> _callbackDeasserted; 00140 Callback<void()> _callbackAssertedHeld; 00141 Callback<void()> _callbackDeassertedHeld; 00142 00143 /** The Ticker periodic callback function 00144 */ 00145 void isr(void); 00146 00147 /** initialise class 00148 * 00149 * @param PinName p is a valid pin that supports DigitalIn 00150 * @param PinMode m The mode the DigitalIn should use. 00151 */ 00152 void init(PinName p, PinMode m); 00153 }; 00154 00155 }; // namespace AjK ends. 00156 00157 using namespace AjK; 00158 00159 #endif
Generated on Wed Jul 13 2022 16:26:39 by
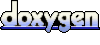