SPKT
Dependencies: SDFileSystem_Warning_Fixed
Dependents: DISCO-F746_WAV_PLAYER WAV
SD_WavReader.hpp
00001 00002 //-------------------------------------------------------------- 00003 00004 #ifndef SD_WAV_READER_HPP 00005 #define SD_WAV_READER_HPP 00006 00007 #include "stm32746g_discovery_audio.h" 00008 #include "SDFileSystem.h" 00009 #include "BlinkLabel.hpp" 00010 #include "Array.hpp" 00011 #include <string> 00012 00013 namespace Mikami 00014 { 00015 class SD_WavReader 00016 { 00017 public: 00018 SD_WavReader(int32_t bufferSize); 00019 virtual ~SD_WavReader(); 00020 00021 void Open(const string fileName); 00022 00023 void Close() { fclose(fp_); } 00024 00025 // Đọc tiêu đề của tệp 00026 bool IsWavFile(); 00027 00028 // Nhận dữ liệu âm thanh nổi từ tệp 00029 void ReadStereo(Array<int16_t>& dataL, Array<int16_t>& dataR); 00030 00031 // Chuyển đổi dữ liệu từ tệp thành đơn âm 00032 void ReadAndToMono(Array<int16_t>& data); 00033 00034 // Lấy kích thước dữ liệu (số điểm lấy mẫu) 00035 int32_t GetSize(); 00036 00037 private: 00038 const string STR_; 00039 00040 struct WaveFormatEx 00041 { 00042 uint16_t wFormatTag; // 1: PCM 00043 uint16_t nChannels; // 1: 1: đơn âm, 2: âm thanh nổi 00044 uint32_t nSamplesPerSec; // Tần số lấy mẫu (Hz) 00045 uint32_t nAvgBytesPerSec; // Tốc độ truyền (byte / s) 00046 uint16_t nBlockAlign; // 4: Trong trường hợp âm thanh nổi 16 bit 00047 uint16_t wBitsPerSample; // Số bit dữ liệu, 8 hoặc 16 00048 uint16_t cbSize; // Không được sử dụng cho PCM 00049 }; 00050 00051 SDFileSystem *sd_; 00052 FILE *fp_; 00053 00054 bool ok_; 00055 int32_t size_; // Kích thước dữ liệu (số điểm lấy mẫu) 00056 Array<int16_t> buffer; // Khu vực làm việc 00057 00058 void ErrorMsg(char msg[]) 00059 { BlinkLabel errLabel(240, 100, msg, Label::CENTER); } 00060 // Không sử dụng hàm tạo sao chép và toán tử gán đối tượng 00061 SD_WavReader(const SD_WavReader&); 00062 SD_WavReader& operator=(const SD_WavReader&); 00063 }; 00064 } 00065 #endif // SD_BINARY_READER_HPP
Generated on Thu Jul 21 2022 07:35:28 by
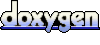