
Breathalyzer running on the MBED 1768 Platform; EE4427 Group 3 Spring 2018
Dependencies: 4DGL-uLCD-SE mbed
main.cpp
00001 //Written by Todd Clark 00002 #include "mbed.h" 00003 #include "lcdOut.h" 00004 #include "sensor.h" 00005 #include "light.h" 00006 #include "convertToBAC.h" 00007 #include "switch.h" 00008 00009 BusOut onboardLED {LED1,LED2,LED3,LED4}; 00010 InterruptIn button(p18); 00011 Timer debounce; 00012 Ticker reset; 00013 00014 bool overLimit; 00015 bool lightsOn = false; 00016 00017 bool isOverLimit(float out, bool isUtahMode){ 00018 float legalLimitBAC = (isUtahMode) ? 0.05 : 0.08; 00019 return (out < legalLimitBAC) ? false : true; 00020 } 00021 00022 void resetLights(){ 00023 lightsOn = false; 00024 turnOff(); 00025 resetDisplay(); 00026 onboardLED = 0x0; 00027 } 00028 00029 void runTest(){ 00030 reset.attach(&resetLights, 30); 00031 bool isUtahMode = getUtahMode(); 00032 float input = takeReading(); 00033 float out = getBAC(input); 00034 overLimit = isOverLimit(out, isUtahMode); 00035 displayOut(out, isUtahMode); 00036 } 00037 00038 void toggle(){ 00039 if (debounce.read_ms()>200){ 00040 runTest(); 00041 lightsOn = true; 00042 debounce.reset(); 00043 } 00044 } 00045 00046 void initialize(){ 00047 debounce.start(); 00048 button.rise(&toggle); 00049 } 00050 00051 int main(){ 00052 float delay = 0.1; 00053 displayOut(-1.0, false); 00054 while(1){ 00055 initialize(); 00056 if(overLimit && lightsOn){ 00057 changeBlue(); 00058 onboardLED = 0x1; 00059 wait(delay); 00060 changeRed(); 00061 onboardLED = 0x2; 00062 wait(delay); 00063 changeBlue(); 00064 onboardLED = 0x4; 00065 wait(delay); 00066 changeRed(); 00067 onboardLED = 0x8; 00068 } 00069 else if(lightsOn){ 00070 changeGreen(); 00071 onboardLED = 0x0; 00072 } 00073 else{ 00074 turnOff(); 00075 onboardLED = 0x0; 00076 } 00077 wait(delay); 00078 } 00079 } 00080 00081
Generated on Wed Jul 20 2022 20:14:45 by
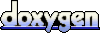