
a
Dependencies: BSP_DISCO_F429ZI LCD_DISCO_F429ZI TS_DISCO_F429ZI mbed
Led_Lcd.cpp
00001 #include "mbed.h" 00002 #include "Led_Lcd.h" 00003 00004 LCD_DISCO_F429ZI lcd; 00005 00006 void LedLcd::ButtonNoPushed(uint16_t x_pos,uint16_t y_pos, uint16_t width, uint16_t height){ 00007 lcd.SetTextColor(LCD_COLOR_GREEN); 00008 lcd.DrawRect(x_pos,y_pos,width,height); 00009 lcd.SetTextColor(LCD_COLOR_BLUE); 00010 lcd.FillRect(x_pos+1,y_pos+1,width-1,height-1); 00011 } 00012 void LedLcd::ButtonPushed(uint16_t x_pos,uint16_t y_pos, uint16_t width, uint16_t height) { 00013 lcd.SetTextColor(LCD_COLOR_GREEN); 00014 lcd.DrawRect(x_pos,y_pos,width,height); 00015 lcd.SetTextColor(LCD_COLOR_GREEN); 00016 lcd.FillRect(x_pos+1,y_pos+1,width-1,height-1); 00017 } 00018 00019 void LedLcd::SetString(uint8_t x_pos,uint8_t y_pos,uint8_t *pText, Text_AlignModeTypdef mode) { 00020 lcd.SetFont(&Font24); 00021 lcd.SetTextColor(LCD_COLOR_WHITE); 00022 lcd.SetBackColor(LCD_COLOR_RED); 00023 lcd.DisplayStringAt(x_pos, y_pos,pText, mode); 00024 } 00025 00026 LedLcd::LedLcd() { 00027 lcd.Clear(LCD_COLOR_BLACK); 00028 } 00029 00030 void LedLcd::On(uint8_t ButtonNum) { 00031 00032 ButtonNoPushed(0,0,80,80); 00033 ButtonNoPushed(0,79,80,80); 00034 ButtonNoPushed(0,159,80,80); 00035 ButtonNoPushed(0,239,80,80); 00036 00037 if(ButtonNum==0) { 00038 ButtonPushed(0,0,80,80); 00039 } 00040 else if(ButtonNum==1) { 00041 ButtonPushed(0,79,80,80); 00042 } 00043 else if(ButtonNum==2) { 00044 ButtonPushed(0,159,80,80); 00045 } 00046 else if(ButtonNum==3) { 00047 ButtonPushed(0,239,80,80); 00048 } 00049 else if(ButtonNum==4) { 00050 } 00051 00052 SetString(0,0,(uint8_t *)"0",LEFT_MODE); 00053 SetString(0,79,(uint8_t *)"1",LEFT_MODE); 00054 SetString(0,159,(uint8_t *)"2",LEFT_MODE); 00055 SetString(0,239,(uint8_t *)"3",LEFT_MODE); 00056 } 00057
Generated on Sun Jul 24 2022 03:54:20 by
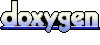