CAN-Bus ECU simulator. Only part of the SAE J1979 are implemented. Uses CAN-Bus demo board as hardware platform. http://skpang.co.uk/catalog/canbus-ecu-simulator-with-lpc1768-module-p-1400.html Useful for testing diagnostic tools.
Fork of ecu_reader by
main.cpp
00001 /* 00002 00003 mbed Can-Bus ECU simulator 00004 00005 v1.0 December 2014 00006 00007 ******************************************************************************** 00008 00009 WARNING: Use at your own risk, sadly this software comes with no guarantees. 00010 This software is provided 'free' and in good faith, but the author does not 00011 accept liability for any damage arising from its use. 00012 00013 ******************************************************************************** 00014 00015 */ 00016 00017 #include "mbed.h" 00018 #include "ecu_simulator.h" 00019 #include "globals.h" 00020 #include "TextLCD.h" 00021 00022 TextLCD lcd(p18, p19, p20, p17, p16, p15, p14); // rs, rw, e, d0, d1, d2, d3 00023 00024 DigitalIn click(p21); // Joystick inputs 00025 DigitalIn right(p22); 00026 DigitalIn down(p23); 00027 DigitalIn left(p24); 00028 DigitalIn up(p25); 00029 00030 ecu_sim sim(CANSPEED_500); //Create object and set CAN speed 00031 ecu_t ecu; 00032 00033 void update_menu(void); 00034 void update_param(unsigned char dir); 00035 00036 // Menu defines 00037 #define L0_MAIN_MENU 0 00038 #define L0_RPM 1 00039 #define L0_THROTTLE 2 00040 #define L0_SPEED 3 00041 #define L0_COOLANT 4 00042 #define L0_MAF 5 00043 #define L0_O2 6 00044 #define L0_DTC 7 00045 00046 #define INC 0 00047 #define DEC 1 00048 00049 #define CAN250 0 00050 #define CAN500 1 00051 unsigned char menu_state; 00052 unsigned char canspeed; 00053 00054 int main() 00055 { 00056 pc.baud(115200); 00057 00058 //Enable Pullup 00059 click.mode(PullUp); 00060 right.mode(PullUp); 00061 down.mode(PullUp); 00062 left.mode(PullUp); 00063 up.mode(PullUp); 00064 00065 printf("\n\nECU Simulator v1.0 \n"); 00066 00067 led1 = 1; 00068 wait(0.1); 00069 led2 = 1; 00070 wait(0.1); 00071 led3 = 1; 00072 wait(0.1); 00073 led4 = 1; 00074 wait(0.2); 00075 led1 = 0; led2 = 0; led3 = 0; led4 = 0; 00076 00077 lcd.cls(); 00078 lcd.locate(0,0); // Set LCD cursor position 00079 lcd.printf("ECU Simulator v1.0"); 00080 lcd.locate(0,1); 00081 lcd.printf("www.skpang.co.uk"); 00082 00083 wait(1); 00084 lcd.cls(); 00085 00086 lcd.printf("<- Params ->"); 00087 lcd.locate(0,1); 00088 lcd.printf("500kb/s"); 00089 00090 canspeed = CAN500; 00091 menu_state = L0_MAIN_MENU; 00092 ecu.dtc = false; 00093 00094 wait(0.2); 00095 led1 = 1; 00096 00097 while(1) { // Main CAN loop 00098 00099 sim.request(); 00100 00101 if(!down){ 00102 update_param(DEC); 00103 } 00104 00105 if(!up){ 00106 update_param(INC); 00107 } 00108 00109 if(!left){ 00110 if(menu_state != L0_MAIN_MENU) menu_state--; 00111 update_menu(); 00112 } 00113 00114 if(!right){ 00115 if(menu_state != L0_DTC) menu_state++; 00116 update_menu(); 00117 } 00118 00119 } 00120 } 00121 void update_param(unsigned char dir) 00122 { 00123 char buffer[20]; 00124 lcd.locate(0,1); 00125 switch(menu_state) 00126 { 00127 case L0_MAIN_MENU: 00128 if(dir == INC){ 00129 sim.canspeed(CANSPEED_500); 00130 lcd.printf("500kb/s"); 00131 canspeed = CAN500; 00132 }else { 00133 sim.canspeed(CANSPEED_250); 00134 lcd.printf("250kb/s"); 00135 canspeed = CAN250; 00136 } 00137 00138 break; 00139 00140 case L0_RPM: 00141 if(dir == INC){ 00142 ecu.engine_rpm = ecu.engine_rpm +10; 00143 } else ecu.engine_rpm = ecu.engine_rpm - 10; 00144 00145 sprintf(buffer,"%d RPM ", (int)((((ecu.engine_rpm & 0xff00) >> 8) * 256) + (ecu.engine_rpm & 0x00ff)) / 4); 00146 lcd.printf(buffer); 00147 break; 00148 00149 case L0_THROTTLE: 00150 if(dir == INC){ 00151 ecu.throttle_position++; 00152 } else ecu.throttle_position--; 00153 00154 sprintf(buffer,"%d %% ", (int)ecu.throttle_position); 00155 lcd.printf(buffer); 00156 break; 00157 00158 case L0_SPEED: 00159 if(dir == INC){ 00160 ecu.vehicle_speed++; 00161 } else ecu.vehicle_speed--; 00162 00163 sprintf(buffer,"%d km/h ",(int) ecu.vehicle_speed); 00164 lcd.printf(buffer); 00165 break; 00166 00167 case L0_COOLANT: 00168 if(dir == INC){ 00169 ecu.coolant_temp++; 00170 } else ecu.coolant_temp--; 00171 00172 sprintf(buffer,"%d C ",(int) ecu.coolant_temp - 40); 00173 lcd.printf(buffer); 00174 break; 00175 00176 case L0_MAF: 00177 if(dir == INC){ 00178 ecu.maf_airflow = ecu.maf_airflow +10; 00179 } else ecu.maf_airflow = ecu.maf_airflow - 10; 00180 00181 sprintf(buffer,"%d g/s ", (int)((((ecu.maf_airflow & 0xff00) >> 8) * 256) + (ecu.maf_airflow & 0x00ff)) / 100); 00182 lcd.printf(buffer); 00183 break; 00184 00185 case L0_O2: 00186 if(dir == INC){ 00187 ecu.o2_voltage = ecu.o2_voltage +10; 00188 } else ecu.o2_voltage = ecu.o2_voltage - 10; 00189 00190 sprintf(buffer,"%d ", (int)ecu.o2_voltage); 00191 lcd.printf(buffer); 00192 break; 00193 00194 case L0_DTC: 00195 if(dir == INC){ 00196 ecu.dtc = true; 00197 led4 = 1; 00198 } else { 00199 ecu.dtc = false; 00200 led4 = 0; 00201 } 00202 00203 sprintf(buffer,"%d ", (int)ecu.dtc); 00204 lcd.printf(buffer); 00205 break; 00206 00207 } 00208 00209 wait(0.1); //Delay for auto repeat 00210 00211 00212 } 00213 void update_menu(void) 00214 { 00215 char buffer[20]; 00216 lcd.cls(); 00217 lcd.locate(0,0); 00218 00219 switch(menu_state) 00220 { 00221 case L0_MAIN_MENU: 00222 lcd.printf("CAN speed"); 00223 lcd.locate(0,1); 00224 if(canspeed == CAN500) lcd.printf("500kb/s"); 00225 else lcd.printf("250kb/s"); 00226 break; 00227 00228 case L0_RPM: 00229 lcd.printf("Engine RPM"); 00230 lcd.locate(0,1); 00231 sprintf(buffer,"%d RPM ", (int) ((((ecu.engine_rpm & 0xff00) >> 8) * 256) + (ecu.engine_rpm & 0x00ff)) / 4); 00232 lcd.printf(buffer); 00233 break; 00234 00235 case L0_THROTTLE: 00236 lcd.printf("Throttle pos"); 00237 lcd.locate(0,1); 00238 sprintf(buffer,"%d % ", ecu.throttle_position); 00239 lcd.printf(buffer); 00240 break; 00241 00242 case L0_SPEED: 00243 lcd.printf("Vehicle speed"); 00244 lcd.locate(0,1); 00245 sprintf(buffer,"%d kph ", ecu.vehicle_speed); 00246 lcd.printf(buffer); 00247 break; 00248 00249 case L0_COOLANT: 00250 lcd.printf("Coolant temp"); 00251 lcd.locate(0,1); 00252 sprintf(buffer,"%d C ",(int) ecu.coolant_temp - 40); 00253 lcd.printf(buffer); 00254 break; 00255 00256 case L0_MAF: 00257 lcd.printf("MAF air flow "); 00258 lcd.locate(0,1); 00259 sprintf(buffer,"%d g/s ", ecu.maf_airflow); 00260 lcd.printf(buffer); 00261 break; 00262 00263 case L0_O2: 00264 lcd.printf("Oxygen sensor v."); 00265 lcd.locate(0,1); 00266 sprintf(buffer,"%d ", ecu.o2_voltage); 00267 lcd.printf(buffer); 00268 break; 00269 00270 case L0_DTC: 00271 lcd.printf("DTC"); 00272 lcd.locate(0,1); 00273 sprintf(buffer,"%d ", ecu.dtc); 00274 lcd.printf(buffer); 00275 break; 00276 00277 } 00278 00279 while(!left); //Wait for key to be released 00280 while(!right); 00281 wait(0.05); 00282 00283 } 00284 00285 00286
Generated on Wed Jul 13 2022 23:36:49 by
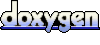