
Dependencies: FatFileSystem mbed GPS TextLCD
main.cpp
00001 /* 00002 00003 mbed Can-Bus demo 00004 00005 This program is to demonstrate the CAN-bus capability of the mbed module. 00006 00007 http://www.skpang.co.uk/catalog/product_info.php?products_id=741 00008 00009 v1.0 July 2010 00010 00011 ******************************************************************************** 00012 00013 WARNING: Use at your own risk, sadly this software comes with no guarantees. 00014 This software is provided 'free' and in good faith, but the author does not 00015 accept liability for any damage arising from its use. 00016 00017 ******************************************************************************** 00018 00019 00020 */ 00021 00022 #include "mbed.h" 00023 #include "ecu_reader.h" 00024 #include "globals.h" 00025 #include "TextLCD.h" 00026 #include "GPS.h" 00027 #include "SDFileSystem.h" 00028 00029 GPS gps(p28, p27); 00030 TextLCD lcd(p18, p19, p20, p17, p16, p15, p14); // rs, rw, e, d0, d1, d2, d3 00031 SDFileSystem sd(p5, p6, p7, p13, "sd"); 00032 00033 DigitalIn click(p21); // Joystick inputs 00034 DigitalIn right(p22); 00035 DigitalIn down(p23); 00036 DigitalIn left(p24); 00037 DigitalIn up(p25); 00038 Serial pc(USBTX, USBRX); 00039 00040 00041 ecu_reader obdii(CANSPEED_500); //Create object and set CAN speed 00042 void gps_demo(void); 00043 void sd_demo(void); 00044 00045 int main() { 00046 pc.baud(115200); 00047 char buffer[20]; 00048 00049 //Enable Pullup 00050 click.mode(PullUp); 00051 right.mode(PullUp); 00052 down.mode(PullUp); 00053 left.mode(PullUp); 00054 up.mode(PullUp); 00055 00056 printf("ECU Reader \n"); 00057 lcd.locate(0,0); // Set LCD cursor position 00058 lcd.printf("CAN-Bus demo"); 00059 00060 lcd.locate(0,1); 00061 lcd.printf("www.skpang.co.uk"); 00062 00063 pc.printf("\n\rCAN-bus demo..."); 00064 00065 wait(3); 00066 lcd.cls(); 00067 lcd.printf("Use joystick"); 00068 00069 lcd.locate(0,1); 00070 lcd.printf("U-CAN:D-GPS:L-SD"); 00071 00072 pc.printf("\nU-CAN:D-GPS:L-SD"); 00073 00074 while(1) // Wait until option is selected by the joystick 00075 { 00076 00077 if(down == 0) gps_demo(); 00078 if(left == 0) sd_demo(); 00079 00080 if(up == 0) break; 00081 00082 } 00083 lcd.cls(); 00084 00085 while(1) { // Main CAN loop 00086 led2 = 1; 00087 wait(0.1); 00088 led2 = 0; 00089 wait(0.1); 00090 00091 if(obdii.request(ENGINE_RPM,buffer) == 1) // Get engine rpm and display on LCD 00092 { 00093 lcd.locate(0,0); 00094 lcd.printf(buffer); 00095 pc.printf(buffer); 00096 } 00097 00098 if(obdii.request(ENGINE_COOLANT_TEMP,buffer) == 1) 00099 { 00100 lcd.locate(9,0); 00101 lcd.printf(buffer); 00102 } 00103 00104 if(obdii.request(VEHICLE_SPEED,buffer) == 1) 00105 { 00106 lcd.locate(0,1); 00107 lcd.printf(buffer); 00108 } 00109 00110 if(obdii.request(THROTTLE,buffer) ==1 ) 00111 { 00112 lcd.locate(9,1); 00113 lcd.printf(buffer); 00114 } 00115 00116 } 00117 } 00118 00119 void gps_demo(void) 00120 { 00121 lcd.cls(); 00122 lcd.printf("GPS demo"); 00123 lcd.locate(0,1); 00124 lcd.printf("Waiting for lock"); 00125 00126 wait(3); 00127 lcd.cls(); 00128 00129 while(1) 00130 { 00131 if(gps.sample()) { 00132 lcd.cls(); 00133 lcd.printf("Long:%f", gps.longitude); 00134 lcd.locate(0,1); 00135 lcd.printf("Lat:%f", gps.latitude); 00136 pc.printf("I'm at %f, %f\n", gps.longitude, gps.latitude); 00137 } else { 00138 pc.printf("Oh Dear! No lock :(\n"); 00139 lcd.cls(); 00140 lcd.printf("Waiting for lock"); 00141 00142 } 00143 } 00144 00145 } 00146 00147 void sd_demo(void) 00148 { 00149 lcd.cls(); 00150 printf("\nSD demo"); 00151 lcd.printf("SD demo"); 00152 wait(2); 00153 lcd.cls(); 00154 00155 FILE *fp = fopen("/sd/sdtest2.txt", "w"); 00156 if(fp == NULL) { 00157 lcd.cls(); 00158 lcd.printf("Could not open file for write\n"); 00159 pc.printf("\nCould not open file for write"); 00160 } 00161 fprintf(fp, "Hello fun SD Card World! testing 1234"); 00162 fclose(fp); 00163 lcd.locate(0,1); 00164 lcd.printf("Writtern to SD card"); 00165 pc.printf("\nWrittern to SD card"); 00166 00167 while(1) 00168 { 00169 led2 = 1; 00170 wait(0.1); 00171 led2 = 0; 00172 wait(0.1); 00173 00174 } 00175 00176 }
Generated on Fri Jul 15 2022 13:02:58 by
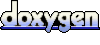