LCD driver
Dependents: ecu_reader ecu_reader ecu_simulator regulator_napona
TextLCD.h
00001 /* mbed TextLCD Library 00002 * Copyright (c) 2007-2009 sford 00003 * Released under the MIT License: http://mbed.org/license/mit 00004 */ 00005 00006 #ifndef MBED_TEXTLCD_H 00007 #define MBED_TEXTLCD_H 00008 00009 #include "Stream.h" 00010 #include "DigitalOut.h" 00011 #include "BusOut.h" 00012 00013 namespace mbed { 00014 00015 /* Class: TextLCD 00016 * A 16x2 Text LCD controller 00017 * 00018 * Allows you to print to a Text LCD screen, and locate/cls. Could be 00019 * turned in to a more generic libray. 00020 * 00021 * If you are connecting multiple displays, you can connect them all in 00022 * parallel except for the enable (e) pin, which must be unique for each 00023 * display. 00024 * 00025 * Example: 00026 * > #include "mbed.h" 00027 * > #include "TextLCD.h" 00028 * > 00029 * > TextLCD lcd(p24, p25, p26, p27, p28, p29, p30); // rs, rw, e, d0, d1, d2, d3 00030 * > 00031 * > int main() { 00032 * > lcd.printf("Hello World!"); 00033 * > } 00034 */ 00035 class TextLCD : public Stream { 00036 00037 public: 00038 /* Constructor: TextLCD 00039 * Create a TextLCD object, connected to the specified pins 00040 * 00041 * All signals must be connected to DigitalIn compatible pins. 00042 * 00043 * Variables: 00044 * rs - Used to specify data or command 00045 * rw - Used to determine read or write 00046 * e - enable 00047 * d0..d3 - The data lines 00048 */ 00049 TextLCD(PinName rs, PinName rw, PinName e, PinName d0, PinName d1, 00050 PinName d2, PinName d3, int columns = 16, int rows = 2); 00051 00052 #if 0 // Inhereted from Stream, for documentation only 00053 /* Function: putc 00054 * Write a character 00055 * 00056 * Variables: 00057 * c - The character to write to the serial port 00058 */ 00059 int putc(int c); 00060 00061 /* Function: printf 00062 * Write a formated string 00063 * 00064 * Variables: 00065 * format - A printf-style format string, followed by the 00066 * variables to use in formating the string. 00067 */ 00068 int printf(const char* format, ...); 00069 #endif 00070 00071 /* Function: locate 00072 * Locate to a certian position 00073 * 00074 * Variables: 00075 * column - the column to locate to, from 0..15 00076 * row - the row to locate to, from 0..1 00077 */ 00078 virtual void locate(int column, int row); 00079 00080 /* Function: cls 00081 * Clear the screen, and locate to 0,0 00082 */ 00083 virtual void cls(); 00084 00085 virtual void reset(); 00086 00087 protected: 00088 00089 void clock(); 00090 void writeData(int data); 00091 void writeCommand(int command); 00092 void writeByte(int value); 00093 void writeNibble(int value); 00094 virtual int _putc(int c); 00095 virtual int _getc(); 00096 virtual void newline(); 00097 00098 int _row; 00099 int _column; 00100 DigitalOut _rw, _rs, _e; 00101 BusOut _d; 00102 int _columns; 00103 int _rows; 00104 00105 }; 00106 00107 } 00108 00109 #endif
Generated on Tue Jul 12 2022 13:01:43 by
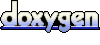