Changed MCP3221 I2C read from two 1-byte reads to one 2-byte read
Dependents: DISCO-F746NG_LCD_TS_ADC
Fork of MCP3221 by
MCP3221.cpp
00001 00002 #include "MCP3221.h" 00003 00004 //Create instance 00005 MCP3221::MCP3221(PinName sda, PinName scl, float supplyVoltage) : i2c(sda, scl), _supplyVoltage(supplyVoltage) 00006 { 00007 } 00008 00009 //destroy instance 00010 MCP3221::~MCP3221() 00011 { 00012 } 00013 00014 float MCP3221::read() 00015 { 00016 00017 //You cannot write to an MCP3221, it has no writable registers. 00018 //MCP3221 also requires an ACKnowledge between each byte sent, before it will send the next byte. So we need to be a bit manual with how we talk to it. 00019 //It also needs an (NOT) ACKnowledge after the second byte or it will keep sending bytes (continuous sampling) 00020 // 00021 //From the datasheet. 00022 // 00023 //I2C.START 00024 //Send 8 bit device/ part address to open conversation. (See .h file for part explanation) 00025 //read a byte (with ACK) 00026 //read a byte (with NAK) 00027 //I2C.STOP 00028 00029 00030 // char data[2]; 00031 /* 00032 i2c.start(); 00033 int acknowledged = i2c.write(MCP3221_CONVERSE); //send a byte to start the conversation. It should be acknowledged. 00034 _data[0] = i2c.read(1); //read a byte. acknowledge when we have it. 00035 _data[1] = i2c.read(0); //read the second byte. (n)acknowledge when we have it to stop the flow. 00036 i2c.stop(); 00037 */ 00038 // PA20170202 in the DISCO-F746NG the above does not return the LS byte, however this works 00039 i2c.read(MCP3221_CONVERSE, _data, 2); 00040 //convert to 12 bit. 00041 short res; 00042 int _12_bit_var; // 2 bytes 00043 char _4_bit_MSnibble = _data[0]; // 1 byte, example 0000 1000 00044 char _8_bit_LSByte = _data[1]; // 1 byte, example 1111 0000 00045 00046 _12_bit_var = ((0x0F & _4_bit_MSnibble) << 8) | _8_bit_LSByte; //example 100011110000 00047 res=_12_bit_var; 00048 00049 return (_supplyVoltage/4096) * res; 00050 00051 } 00052
Generated on Thu Jul 14 2022 01:04:56 by
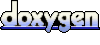