
HTTP Server upon new mbed Ethernet Interface. Based on original code by Henry Leinen.
Dependencies: EthernetInterface mbed-rtos mbed
Fork of HTTP_server by
HTTPServer.h
00001 /* 00002 Copyright (c) 2013 Pablo Gindel (palmer@pablogindel.com) 00003 Based on original code by Henry Leinen (henry[dot]leinen [at] online [dot] de) 00004 00005 Permission is hereby granted, free of charge, to any person obtaining a copy 00006 of this software and associated documentation files (the "Software"), to deal 00007 in the Software without restriction, including without limitation the rights 00008 to use, copy, modify, merge, publish, distribute, sublicense, and/or sell 00009 copies of the Software, and to permit persons to whom the Software is 00010 furnished to do so, subject to the following conditions: 00011 00012 The above copyright notice and this permission notice shall be included in 00013 all copies or substantial portions of the Software. 00014 00015 THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR 00016 IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, 00017 FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE 00018 AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER 00019 LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, 00020 OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN 00021 THE SOFTWARE. 00022 */ 00023 00024 00025 #ifndef __HTTPSERVER_H__ 00026 #define __HTTPSERVER_H__ 00027 00028 #include "mbed.h" 00029 #include "EthernetInterface.h" 00030 #include <vector> 00031 #include <string> 00032 #include <map> 00033 00034 #define BUFFER_SIZE 256 // all-purpose buffer 00035 #define TIMEOUT 500 00036 #define OK 0 00037 #define ERROR -1 00038 #define EMPTY -2 00039 #define MIN_LONG 3 00040 #define CHUNK_SIZE 256 00041 00042 #define DEBUG 2 00043 #include "debug.h" 00044 00045 enum RequestType { 00046 HTTP_RT_GET, /*!< GET request */ 00047 HTTP_RT_POST, /*!< POST request */ 00048 }; 00049 00050 /** HTTPMessage contains all the details of the request received by external HTTP client. */ 00051 struct HTTPMsg { 00052 // Specifies the request type received 00053 RequestType request; 00054 // The uri associated with the request. 00055 std::string uri; 00056 // Contains the HTTP/1.1 or HTTP/1.0 version requested by client. 00057 std::string version; 00058 // Map of headers provided by the client in the form <HeaderName>:<HeaderValue> 00059 std::map<std::string, std::string> headers; 00060 // Map of arguments that came with the uri string 00061 std::map<std::string, std::string> uri_args; 00062 // length of the body data section 00063 int body_length; 00064 }; 00065 00066 struct RequestConfig { 00067 const char* request_string; 00068 RequestType request_type; 00069 }; 00070 00071 class HTTPServer { 00072 00073 private: 00074 TCPSocketServer socketServer; 00075 TCPSocketConnection *cliente; 00076 HTTPMsg *msg; 00077 std::string path; 00078 char buffer [BUFFER_SIZE]; 00079 int pollConnection (); 00080 int receiveLine (); 00081 int parseRequest (); 00082 int parseHeader (); 00083 int parseUriArgs (char *uri_buffer); 00084 void handleRequest (); 00085 int handleGetRequest(); 00086 int handlePostRequest(); 00087 void startResponse (int returnCode, int nLen); 00088 void handleError (int errorCode); 00089 00090 int tcpsend (const char* text, int param) { 00091 sprintf (buffer, text, param); 00092 return tcpsend (buffer, strlen (buffer)); 00093 } 00094 00095 int tcpsend (const char* text) { 00096 return tcpsend ((char*)text, strlen (text)); 00097 } 00098 00099 /*int tcpsend (char* buf, int len) { 00100 int sent = 0; 00101 while (sent<len) { 00102 sent += cliente->send (buf+sent, len-sent); 00103 if (sent == ERROR) { 00104 WARN("Unsent bytes left !"); 00105 return ERROR; 00106 } 00107 } 00108 return OK; 00109 }*/ 00110 00111 int tcpsend (char* buf, int len) { 00112 if (cliente->send_all (buf, len) != len) { 00113 WARN("Unsent bytes left !"); 00114 return ERROR; 00115 } 00116 return OK; 00117 } 00118 00119 public: 00120 /** Constructor for HTTPServer objects. */ 00121 HTTPServer (int port, const char* _path); 00122 00123 /** Destructor for HTTPServer objects. */ 00124 ~HTTPServer(); 00125 00126 int poll(); 00127 }; 00128 00129 00130 void url_decode (char *str); 00131 00132 00133 00134 #endif //__HTTPSERVER_H__
Generated on Thu Jul 14 2022 22:45:21 by
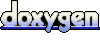