KeyboardManager: a class to manage the polling of a switch-matrix keyboard
Embed:
(wiki syntax)
Show/hide line numbers
LongKeyPressMonitor.h
00001 #ifndef LONG_KEY_PRESS_MONITOR_H_ 00002 #define LONG_KEY_PRESS_MONITOR_H_ 00003 00004 #include <kbd_mgr/KeyPressEventServer.h> 00005 #include <set> 00006 00007 #include "mbed.h" 00008 00009 namespace kbd_mgr { 00010 00011 /** 00012 * @brief A key press event handler that detects long key presses and report them as specified. 00013 * This class offers two specific reactions for long key presses. Either a specific long key press is reported 00014 * or the key press event is auto repeated. The timing for both kind of reactions can be specified independently. 00015 * The monitor reacts on key code, not on mapped key char. If a mapped key char is present in the event, it is retained 00016 * in the generated key press events. 00017 */ 00018 class LongKeyPressMonitor : public KeyPressEventServer, public KeyPressEventHandler { 00019 public: 00020 LongKeyPressMonitor() : 00021 repeatKeys_(), repeatInitTime_(0), repeatDelay_(0), longPressKeys_(), longPressTime_(0), 00022 state(Idle), keyDownCount(0), timer() 00023 { } 00024 00025 class AutoRepeatSetupProxy { 00026 public: 00027 AutoRepeatSetupProxy(LongKeyPressMonitor *monitor) : monitor_(monitor) { } 00028 AutoRepeatSetupProxy& operator()(int key) { this->monitor_->addAutoRepeatKey(key, key); return *this; } 00029 AutoRepeatSetupProxy& operator()(int firstKey, int lastKey) { this->monitor_->addAutoRepeatKey(firstKey, lastKey); return *this; } 00030 00031 private: 00032 LongKeyPressMonitor *monitor_; 00033 }; 00034 00035 friend class AutoRepeatSetupProxy; 00036 00037 /** 00038 * @brief Sets up auto-repeat keys. 00039 * This method takes the timing parameters. It returns a special class that allows specifying the keys 00040 * between brackets. Eg: 00041 * - monitor.autoRepeat(0.3, 0.1)(1)(2)(4,8) 00042 * Sets up auto repeat after 300ms, every 100ms for keys 1, 2 and 4 to 8. 00043 */ 00044 AutoRepeatSetupProxy autoRepeat(float initTime, float delay); 00045 00046 00047 class LongPressSetupProxy { 00048 public: 00049 LongPressSetupProxy(LongKeyPressMonitor *monitor) : monitor_(monitor) { } 00050 LongPressSetupProxy& operator()(int key) { this->monitor_->addLongPressKey(key, key); return *this; } 00051 LongPressSetupProxy& operator()(int firstKey, int lastKey) { this->monitor_->addLongPressKey(firstKey, lastKey); return *this; } 00052 00053 private: 00054 LongKeyPressMonitor *monitor_; 00055 }; 00056 00057 friend class LongPressSetupProxy; 00058 00059 /** 00060 * @brief Sets up long key press keys. 00061 * This method takes the timing parameters. It returns a special class that allows specifying the keys 00062 * between brackets. Eg: 00063 * - monitor.longKeyPress(0.5)(3)(12,14) 00064 * Sets up report of long key press after 500ms for keys 3 and 12 to 14. 00065 */ 00066 LongPressSetupProxy longKeyPress(float longPressTime); 00067 00068 /** 00069 * @brief KeyPressEventHandler interface 00070 */ 00071 virtual void handleKeyPress(const KeyEvent &keypress); 00072 00073 private: 00074 void addAutoRepeatKey(int firstKey, int lastKey); 00075 bool isAutoRepeatKey(int key) const { return this->repeatKeys_.find(key) != this->repeatKeys_.end(); } 00076 void addLongPressKey(int firstKey, int lastKey); 00077 bool isLongPressKey(int key) const { return this->longPressKeys_.find(key) != this->longPressKeys_.end(); } 00078 00079 void handleKeyDown(const KeyEvent &keypress); 00080 void handleFirstKeyDown(const KeyEvent &keypress); 00081 void handleOtherKeyDown(const KeyEvent &keypress); 00082 void handleKeyUp(const KeyEvent &keypress); 00083 void handleLastKeyUp(const KeyEvent &keypress); 00084 00085 void handleTimer(); 00086 void handleRepeatTimer(); 00087 void handleLongPressTimer(); 00088 00089 typedef std::set<int> KeySet; 00090 00091 KeySet repeatKeys_; 00092 float repeatInitTime_; 00093 float repeatDelay_; 00094 00095 KeySet longPressKeys_; 00096 float longPressTime_; 00097 00098 enum State { 00099 Idle, 00100 RepeatInitWait, 00101 Repeating, 00102 LongPressWait, 00103 LongPressReported, 00104 Invalid 00105 }; 00106 00107 State state; 00108 KeyEvent keypress; 00109 int keyDownCount; 00110 Timeout timer; 00111 }; 00112 00113 } // kbd_mgr 00114 00115 #endif // LONG_KEY_PRESS_MONITOR_H_
Generated on Thu Jul 14 2022 19:25:04 by
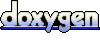