KeyboardManager: a class to manage the polling of a switch-matrix keyboard
Embed:
(wiki syntax)
Show/hide line numbers
LongKeyPressMonitor.cpp
00001 #include <kbd_mgr/LongKeyPressMonitor.h> 00002 00003 namespace kbd_mgr { 00004 00005 LongKeyPressMonitor::AutoRepeatSetupProxy LongKeyPressMonitor::autoRepeat(float initTime, float delay) 00006 { 00007 this->repeatInitTime_ = initTime; 00008 this->repeatDelay_ = delay; 00009 00010 return AutoRepeatSetupProxy(this); 00011 } 00012 00013 void LongKeyPressMonitor::addAutoRepeatKey(int firstKey, int lastKey) 00014 { 00015 for(int key = firstKey; key <= lastKey; ++key) { 00016 this->repeatKeys_.insert(key); 00017 this->longPressKeys_.erase(key); 00018 } 00019 } 00020 00021 LongKeyPressMonitor::LongPressSetupProxy LongKeyPressMonitor::longKeyPress(float longPressTime) 00022 { 00023 this->longPressTime_ = longPressTime; 00024 return LongPressSetupProxy(this); 00025 } 00026 00027 void LongKeyPressMonitor::addLongPressKey(int firstKey, int lastKey) 00028 { 00029 for(int key = firstKey; key <= lastKey; ++key) { 00030 this->longPressKeys_.insert(key); 00031 this->repeatKeys_.erase(key); 00032 } 00033 } 00034 00035 void LongKeyPressMonitor::handleKeyPress(const KeyEvent &keypress) 00036 { 00037 if (keypress.event == KeyEvent::KeyDown) { 00038 invokeHandler(keypress); 00039 handleKeyDown(keypress); 00040 } 00041 else if (keypress.event == KeyEvent::KeyUp) { 00042 handleKeyUp(keypress); 00043 invokeHandler(keypress); 00044 } 00045 else { 00046 invokeHandler(keypress); 00047 } 00048 } 00049 00050 void LongKeyPressMonitor::handleKeyDown(const KeyEvent &keypress) 00051 { 00052 this->keyDownCount++; 00053 if (this->keyDownCount == 1) { 00054 handleFirstKeyDown(keypress); 00055 } 00056 else { 00057 handleOtherKeyDown(keypress); 00058 } 00059 } 00060 00061 void LongKeyPressMonitor::handleFirstKeyDown(const KeyEvent &keypress) 00062 { 00063 this->keypress = keypress; 00064 00065 if (isAutoRepeatKey(keypress.keyCode)) { 00066 this->state = RepeatInitWait; 00067 this->timer.attach(this, &LongKeyPressMonitor::handleTimer, this->repeatInitTime_); 00068 } 00069 else if (isLongPressKey(keypress.keyCode)) { 00070 this->state = LongPressWait; 00071 this->timer.attach(this, &LongKeyPressMonitor::handleTimer, this->longPressTime_); 00072 } 00073 } 00074 00075 void LongKeyPressMonitor::handleOtherKeyDown(const KeyEvent &keypress) 00076 { 00077 this->state = Invalid; 00078 this->timer.detach(); 00079 } 00080 00081 void LongKeyPressMonitor::handleKeyUp(const KeyEvent &keypress) 00082 { 00083 this->keyDownCount--; 00084 00085 if (this->state == Invalid || this->state == RepeatInitWait || this->state == LongPressWait) { 00086 KeyEvent pressed(keypress, KeyEvent::KeyPress); 00087 invokeHandler(pressed); 00088 } 00089 00090 if (this->keyDownCount == 0) { 00091 handleLastKeyUp(keypress); 00092 } 00093 } 00094 00095 void LongKeyPressMonitor::handleLastKeyUp(const KeyEvent &keypress) 00096 { 00097 this->state = Idle; 00098 } 00099 00100 void LongKeyPressMonitor::handleTimer() 00101 { 00102 switch(this->state) { 00103 case RepeatInitWait: 00104 case Repeating: 00105 handleRepeatTimer(); 00106 break; 00107 case LongPressWait: 00108 handleLongPressTimer(); 00109 break; 00110 default: 00111 break; 00112 } 00113 } 00114 00115 void LongKeyPressMonitor::handleRepeatTimer() 00116 { 00117 KeyEvent repeated(this->keypress, 00118 (this->state == RepeatInitWait ? KeyEvent::KeyPress : KeyEvent::RepeatedKeyPress)); 00119 invokeHandler(repeated); 00120 00121 this->state = Repeating; 00122 this->timer.attach(this, &LongKeyPressMonitor::handleTimer, this->repeatDelay_); 00123 } 00124 00125 void LongKeyPressMonitor::handleLongPressTimer() 00126 { 00127 KeyEvent longPressed(keypress, KeyEvent::LongKeyPress); 00128 invokeHandler(longPressed); 00129 this->state = LongPressReported; 00130 } 00131 00132 } // kbd_mgr
Generated on Thu Jul 14 2022 19:25:04 by
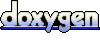