KeyboardManager: a class to manage the polling of a switch-matrix keyboard
Embed:
(wiki syntax)
Show/hide line numbers
KeyboardStateHandler.h
00001 #ifndef KEYBOARD_STATE_HANDLER_H_ 00002 #define KEYBOARD_STATE_HANDLER_H_ 00003 00004 #include "kbd_mgr/KeyboardState.h" 00005 00006 namespace kbd_mgr { 00007 00008 /** 00009 * @brief Interface used to report a keyboard state. 00010 */ 00011 class KeyboardStateHandler { 00012 public: 00013 virtual void handleState(const KeyboardState &newState) = 0; 00014 virtual ~KeyboardStateHandler() { } 00015 }; 00016 00017 template <class T> 00018 class MemberKeyboardStateHandler : public KeyboardStateHandler { 00019 public: 00020 typedef void (T::*MemberFunction)(const KeyboardState &); 00021 00022 MemberKeyboardStateHandler(T *obj, MemberFunction fn) : 00023 object(obj), func(fn) 00024 { } 00025 00026 virtual void handleState(const KeyboardState &newState) { 00027 (object->*func)(newState); 00028 } 00029 00030 private: 00031 T *object; 00032 MemberFunction func; 00033 }; 00034 00035 class FunctionKeyboardStateHandler : public KeyboardStateHandler { 00036 public: 00037 typedef void (*HandlerFunction)(const KeyboardState &); 00038 00039 FunctionKeyboardStateHandler(HandlerFunction fn) : 00040 func(fn) 00041 { } 00042 00043 virtual void handleState(const KeyboardState &newState) { 00044 func(newState); 00045 } 00046 00047 private: 00048 HandlerFunction func; 00049 }; 00050 00051 } // kbd_mgr 00052 00053 #endif // KEYBOARD_STATE_HANDLER_H_
Generated on Thu Jul 14 2022 19:25:04 by
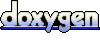