KeyboardManager: a class to manage the polling of a switch-matrix keyboard
Embed:
(wiki syntax)
Show/hide line numbers
KeyboardMonitor.h
00001 #ifndef KEYBOARD_MONITOR_H_ 00002 #define KEYBOARD_MONITOR_H_ 00003 00004 #include "kbd_mgr/KeyboardStateEventServer.h" 00005 #include "kbd_mgr/KeyboardState.h" 00006 00007 #include "mbed.h" 00008 #include <vector> 00009 00010 namespace kbd_mgr { 00011 00012 /** 00013 * @brief A class that polls the state of a switch-matrix keyboard. 00014 * For efficiency reasons, this class uses contiguous bits in a GPIO ports for reading the state of the keys in a row. 00015 * Key rows are activated one by one using a set of digital outputs. 00016 * When a full keyboard scan has been done, it is provided to the registered handler. 00017 */ 00018 class KeyboardMonitor : public KeyboardStateEventServer { 00019 public: 00020 typedef std::vector<PinName> OutPinsSet; 00021 00022 /** 00023 * @param inPort Port to be used for reading the key state. 00024 * @param numKeysPerRow Number of keys in a row (N) 00025 * @param inLowestBit Index of the lowest bit of inPort (using inLowestBit to inLowestBit+N). 00026 * @param outPins Pins to be used for powering each key row. 00027 */ 00028 KeyboardMonitor (PortName inPort, std::size_t numKeysPerRow, std::size_t inLowestBit, 00029 const OutPinsSet &outPins); 00030 00031 ~KeyboardMonitor(); 00032 00033 /** 00034 * @brief Starts the polling of the keyboard state. 00035 */ 00036 void start(float pollingPeriod = 0.001); 00037 00038 /** 00039 * @brief Disables the polling of the keyboard state. 00040 */ 00041 void stop(); 00042 00043 private: 00044 void timerHandler(); 00045 00046 PortIn in; 00047 int inBitShift; 00048 OutPinsSet outPins; 00049 00050 Ticker ticker; 00051 std::size_t scanRow; /*!< next key row to be scanned */ 00052 KeyboardState currentState; /*!< currently being built keyboard state */ 00053 }; 00054 00055 } // kbd_mgr 00056 00057 #endif // KEYBOARD_MONITOR_H_
Generated on Thu Jul 14 2022 19:25:04 by
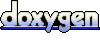