KeyboardManager: a class to manage the polling of a switch-matrix keyboard
Embed:
(wiki syntax)
Show/hide line numbers
KeyboardEventServer.h
00001 #ifndef KEYBOARD_EVENT_SERVER_H_ 00002 #define KEYBOARD_EVENT_SERVER_H_ 00003 00004 #include <cstdlib> 00005 00006 namespace kbd_mgr { 00007 00008 template <class HandlerClass> 00009 class KeyboardEventServer { 00010 protected: 00011 KeyboardEventServer() : handler_(NULL), ownHandler_(false) { } 00012 00013 ~KeyboardEventServer() 00014 { 00015 clearHandler(); 00016 } 00017 00018 void setHandler(HandlerClass *h, bool owner = true) { 00019 this->handler_ = h; 00020 this->ownHandler_ = owner; 00021 } 00022 00023 void clearHandler() { 00024 if (this->ownHandler_) { 00025 delete this->handler_; 00026 } 00027 this->handler_ = NULL; 00028 this->ownHandler_ = false; 00029 } 00030 00031 HandlerClass *handler() const { return this->handler_; } 00032 00033 bool hasHandler() const { return this->handler_ != NULL; } 00034 00035 public: 00036 void attach(HandlerClass *handler) { 00037 setHandler(handler, false); 00038 } 00039 void attach(HandlerClass &handler) { 00040 setHandler(&handler, false); 00041 } 00042 00043 void detach() { 00044 clearHandler(); 00045 } 00046 00047 private: 00048 HandlerClass *handler_; 00049 bool ownHandler_; 00050 }; 00051 00052 } // kbd_mgr 00053 00054 #endif // KEYBOARD_EVENT_SERVER_H_
Generated on Thu Jul 14 2022 19:25:04 by
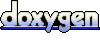