KeyboardManager: a class to manage the polling of a switch-matrix keyboard
Embed:
(wiki syntax)
Show/hide line numbers
KeyPressEventServer.h
00001 #ifndef KEYPRESS_EVENT_SERVER_H_ 00002 #define KEYPRESS_EVENT_SERVER_H_ 00003 00004 #include "kbd_mgr/KeyboardEventServer.h" 00005 #include "kbd_mgr/KeyPressEventHandler.h" 00006 00007 namespace kbd_mgr { 00008 00009 /** 00010 * @brief A base class for monitors that report keypresses. 00011 */ 00012 class KeyPressEventServer : public KeyboardEventServer<KeyPressEventHandler> { 00013 public: 00014 /** 00015 * @brief Attaches the monitor to a function. 00016 * @param fn Event handler called to report keyboard state change. 00017 */ 00018 void attach(FunctionKeyPressEventHandler::HandlerFunction fn) { 00019 setHandler(new FunctionKeyPressEventHandler(fn)); 00020 } 00021 00022 /** 00023 * @brief Attaches the monitor to a method of an object. 00024 * @param obj Event handler object 00025 * @param fn Event handler method called to report keyboard state after each complete scan. 00026 */ 00027 template <class T> 00028 void attach(T *obj, typename MemberKeyPressEventHandler<T>::MemberFunction fn) { 00029 setHandler(new MemberKeyPressEventHandler<T>(obj, fn)); 00030 } 00031 00032 using KeyboardEventServer<KeyPressEventHandler>::attach; 00033 00034 void invokeHandler(const KeyEvent &keypress) { 00035 if (this->hasHandler()) { 00036 this->handler()->handleKeyPress(keypress); 00037 } 00038 } 00039 }; 00040 00041 } // kbd_mgr 00042 00043 #endif // KEYPRESS_EVENT_SERVER_H_
Generated on Thu Jul 14 2022 19:25:04 by
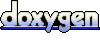