KeyboardManager: a class to manage the polling of a switch-matrix keyboard
Embed:
(wiki syntax)
Show/hide line numbers
KeyPressEventHandler.h
00001 #ifndef KEY_PRESS_EVENT_HANDLER_H_ 00002 #define KEY_PRESS_EVENT_HANDLER_H_ 00003 00004 namespace kbd_mgr { 00005 00006 struct KeyEvent { 00007 enum KeyId { 00008 NoKey = -1 00009 }; 00010 00011 enum EventType { 00012 NoEvent, KeyDown, KeyPress, RepeatedKeyPress, LongKeyPress, KeyUp 00013 }; 00014 00015 int keyCode; 00016 char keyChar; 00017 EventType event; 00018 00019 KeyEvent() : keyCode(NoKey), keyChar(0), event(NoEvent) { } 00020 00021 /** 00022 * @brief Creates a raw key event (no char). 00023 */ 00024 KeyEvent(int key, EventType event) : keyCode(key), keyChar(0), event(event) { } 00025 00026 /** 00027 * @brief Converts a raw key event into a mapped key. 00028 */ 00029 KeyEvent(const KeyEvent &raw, char ch) : keyCode(raw.keyCode), keyChar(ch), event(raw.event) { } 00030 00031 /** 00032 * @brief Creates a key event with a different event code. 00033 */ 00034 KeyEvent(const KeyEvent &other, EventType event) : keyCode(other.keyCode), keyChar(other.keyChar), event(event) { } 00035 }; 00036 00037 /** 00038 * @brief Interface used to report key presses and releases. 00039 */ 00040 class KeyPressEventHandler { 00041 public: 00042 virtual void handleKeyPress(const KeyEvent &keypress) = 0; 00043 virtual ~KeyPressEventHandler() { } 00044 }; 00045 00046 template <class T> 00047 class MemberKeyPressEventHandler : public KeyPressEventHandler { 00048 public: 00049 typedef void (T::*MemberFunction)(const KeyEvent &keypress); 00050 00051 MemberKeyPressEventHandler(T *obj, MemberFunction fn) : 00052 object(obj), func(fn) 00053 { } 00054 00055 virtual void handleKeyPress(const KeyEvent &keypress) { 00056 (object->*func)(keypress); 00057 } 00058 00059 private: 00060 T *object; 00061 MemberFunction func; 00062 }; 00063 00064 class FunctionKeyPressEventHandler : public KeyPressEventHandler { 00065 public: 00066 typedef void (*HandlerFunction)(const KeyEvent &keypress); 00067 00068 FunctionKeyPressEventHandler(HandlerFunction fn) : 00069 func(fn) 00070 { } 00071 00072 virtual void handleKeyPress(const KeyEvent &keypress) { 00073 func(keypress); 00074 } 00075 00076 private: 00077 HandlerFunction func; 00078 }; 00079 00080 } // kbd_mgr 00081 00082 #endif // KEY_PRESS_EVENT_HANDLER_H_
Generated on Thu Jul 14 2022 19:25:04 by
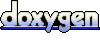