
A DTMF sequence editor and player for HAM radio equipment command & control.
Embed:
(wiki syntax)
Show/hide line numbers
mbed_display_manager.cpp
00001 #include "mbed_display_manager.hpp" 00002 #include <iostream> 00003 00004 using namespace ext_text_lcd; 00005 00006 MbedDisplayManager::MbedDisplayManager() : 00007 lcd(p28, p27, Port2, 0, TextLCD::LCD16x2), pos(0) 00008 { 00009 std::cout << "Init Display" << "\r" << std::endl; 00010 } 00011 00012 void MbedDisplayManager::moveTo(std::size_t pos) 00013 { 00014 std::cout << "Display moveTo " << pos << "\r" << std::endl; 00015 this->pos = pos; 00016 updateCursor(); 00017 } 00018 00019 void MbedDisplayManager::writeStatus(const std::string &text) 00020 { 00021 std::cout << "Display write status '" << text << "'\r" << std::endl; 00022 writeAt(0, text); 00023 } 00024 00025 void MbedDisplayManager::writeText(const std::string &text) 00026 { 00027 std::cout << "Display write text '" << text << "'\r" << std::endl; 00028 writeAt(1, text); 00029 } 00030 00031 void MbedDisplayManager::writeAt(std::size_t row, const std::string &text) 00032 { 00033 std::size_t len = text.size(); 00034 std::string str = (text + std::string(20 - len, ' ')); 00035 lcd.locate(0, row); 00036 lcd.printf(str.c_str()); 00037 updateCursor(); 00038 } 00039 00040 void MbedDisplayManager::updateCursor() 00041 { 00042 lcd.locate(pos, 1); 00043 } 00044 00045 void MbedDisplayManager:: showCursor() 00046 { 00047 std::cout << "Display showCursor" << "\r" << std::endl; 00048 lcd.setDisplayControl(TextLCD::DisplayOn, TextLCD::CursorOn, TextLCD::BlinkingCursor); 00049 } 00050 00051 void MbedDisplayManager:: hideCursor() 00052 { 00053 std::cout << "Display hideCursor" << "\r" << std::endl; 00054 lcd.setDisplayControl(TextLCD::DisplayOn, TextLCD::CursorOff); 00055 } 00056 00057 void MbedDisplayManager:: clear() 00058 { 00059 std::cout << "Display clear" << "\r" << std::endl; 00060 lcd.cls(); 00061 }
Generated on Wed Jul 13 2022 16:23:43 by
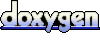