EEPROM emulation using Flash for STM32F103
Embed:
(wiki syntax)
Show/hide line numbers
eeprom_flash.cpp
00001 #include "mbed.h" 00002 #include "eeprom_flash.h" 00003 00004 /* 00005 * Debug option 00006 */ 00007 #if 0 00008 //Enable debug 00009 #include <cstdio> 00010 #define DBG(x, ...) std::printf("[eeprom: DBG]"x"\r\n", ##__VA_ARGS__); 00011 #define WARN(x, ...) std::printf("[eeprom: WARN]"x"\r\n", ##__VA_ARGS__); 00012 #define ERR(x, ...) std::printf("[eeprom: ERR]"x"\r\n", ##__VA_ARGS__); 00013 #else 00014 //Disable debug 00015 #define DBG(x, ...) 00016 #define WARN(x, ...) 00017 #define ERR(x, ...) 00018 #endif 00019 00020 /* 00021 * Must call this first to enable writing 00022 */ 00023 void enableEEPROMWriting() { 00024 HAL_StatusTypeDef status = HAL_FLASH_Unlock(); 00025 FLASH_PageErase(EEPROM_START_ADDRESS); // required to re-write 00026 CLEAR_BIT(FLASH->CR, FLASH_CR_PER); // Bug fix: bit PER has been set in Flash_PageErase(), must clear it here 00027 } 00028 00029 void disableEEPROMWriting() { 00030 HAL_FLASH_Lock(); 00031 } 00032 00033 /* 00034 * Writing functions 00035 * Must call enableEEPROMWriting() first 00036 */ 00037 HAL_StatusTypeDef writeEEPROMHalfWord(uint32_t address, uint16_t data) { 00038 HAL_StatusTypeDef status; 00039 address = address + EEPROM_START_ADDRESS; 00040 00041 status = HAL_FLASH_Program(FLASH_TYPEPROGRAM_HALFWORD, address, data); 00042 00043 return status; 00044 } 00045 00046 HAL_StatusTypeDef writeEEPROMWord(uint32_t address, uint32_t data) { 00047 HAL_StatusTypeDef status; 00048 address = address + EEPROM_START_ADDRESS; 00049 00050 status = HAL_FLASH_Program(FLASH_TYPEPROGRAM_WORD, address, data); 00051 00052 return status; 00053 } 00054 00055 /* 00056 * Reading functions 00057 */ 00058 uint16_t readEEPROMHalfWord(uint32_t address) { 00059 uint16_t val = 0; 00060 address = address + EEPROM_START_ADDRESS; 00061 val = *(__IO uint16_t*)address; 00062 00063 return val; 00064 } 00065 00066 uint32_t readEEPROMWord(uint32_t address) { 00067 uint32_t val = 0; 00068 address = address + EEPROM_START_ADDRESS; 00069 val = *(__IO uint32_t*)address; 00070 00071 return val; 00072 }
Generated on Fri Jul 15 2022 12:59:49 by
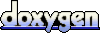