Driver for a CD22M3494 cross point switcher
Embed:
(wiki syntax)
Show/hide line numbers
CD22M3494.h
00001 #ifndef CD22M3494_H 00002 #define CD22M3494_H 00003 00004 #include <mbed.h> 00005 #include <map> 00006 #include <string> 00007 00008 #define LOG_LEVEL_INFO 00009 #include "logger.h" 00010 00011 /* 00012 X ADDRESS 00013 AX3 AX2 AX1 AX0 X SWITCH 00014 0 0 0 0 X0 00015 0 0 0 1 X1 00016 0 0 1 0 X2 00017 0 0 1 1 X3 00018 0 1 0 0 X4 00019 0 1 0 1 X5 00020 0 1 1 0 X12 00021 0 1 1 1 X13 00022 1 0 0 0 X6 00023 1 0 0 1 X7 00024 1 0 1 0 X8 00025 1 0 1 1 X9 00026 1 1 0 0 X10 00027 1 1 0 1 X11 00028 1 1 1 0 X14 00029 1 1 1 1 X15 00030 */ 00031 #define X0 "X0" 00032 #define X1 "X1" 00033 #define X2 "X2" 00034 #define X3 "X3" 00035 #define X4 "X4" 00036 #define X5 "X5" 00037 #define X6 "X6" 00038 #define X7 "X7" 00039 #define X8 "X8" 00040 #define X9 "X9" 00041 #define X10 "X10" 00042 #define X11 "X11" 00043 #define X12 "X12" 00044 #define X13 "X13" 00045 #define X14 "X14" 00046 #define X15 "X15" 00047 00048 00049 #define X0I 0x00 00050 #define X1I 0x01 00051 #define X2I 0x02 00052 #define X3I 0x03 00053 #define X4I 0x04 00054 #define X5I 0x05 00055 #define X12I 0x06 00056 #define X13I 0x07 00057 #define X6I 0x08 00058 #define X7I 0x09 00059 #define X8I 0x0A 00060 #define X9I 0x0B 00061 #define X10I 0x0C 00062 #define X11I 0x0D 00063 #define X14I 0x0E 00064 #define X15I 0x0F 00065 00066 /* 00067 Y ADDRESS 00068 AY2 AY1 AY0 Y SWITCH 00069 0 0 0 Y0 00070 0 0 1 Y1 00071 0 1 0 Y2 00072 0 1 1 Y3 00073 1 0 0 Y4 00074 1 0 1 Y5 00075 1 1 0 Y6 00076 1 1 1 Y7 00077 */ 00078 00079 #define Y0I 0x00 00080 #define Y1I 0x01 00081 #define Y2I 0x02 00082 #define Y3I 0x03 00083 #define Y4I 0x04 00084 #define Y5I 0x05 00085 #define Y6I 0x06 00086 #define Y7I 0x07 00087 00088 #define Y0 "Y0" 00089 #define Y1 "Y1" 00090 #define Y2 "Y2" 00091 #define Y3 "Y3" 00092 #define Y4 "Y4" 00093 #define Y5 "Y5" 00094 #define Y6 "Y6" 00095 #define Y7 "Y7" 00096 00097 #define MAX_X 0x0F 00098 #define MAX_Y 0x07 00099 00100 struct Association { 00101 string name; 00102 string xp1; 00103 string xp2; 00104 }; 00105 00106 /**A driver for the CD22M3494 crosspoint switch matrix. 00107 */ 00108 class CD22M3494 { 00109 00110 public: 00111 00112 /**Constructs a new CD22M3494 object 00113 * 00114 * @param x0 the pin name for the first bit of the x axis address 00115 * @param x1 the pin name for the second bit of the x axis address 00116 * @param x2 the pin name for the thrid bit of the x axis address 00117 * @param x3 the pin name for the forth bit of the x axis address 00118 * @param y0 the pin name for the first bit of the y axis address 00119 * @param y1 the pin name for the second bit of the y axis address 00120 * @param y2 the pin name for the thrid bit of the y axis address 00121 * @param d the pin name for the data bus 00122 * @param str the pin name for the strobe bus 00123 * @param rs the pin name for reset. 00124 */ 00125 CD22M3494(PinName x0, PinName x1, PinName x2, PinName x3, PinName y0, PinName y1, PinName y2, PinName d, PinName str, PinName rs) { 00126 xbus = new BusOut(x0, x1, x2, x3); 00127 ybus = new BusOut(y0, y1, y2); 00128 data = new DigitalOut(d); 00129 strobe = new DigitalOut(str); 00130 reset = new DigitalOut(rs); 00131 data->write(0); 00132 strobe->write(0); 00133 reset->write(0); 00134 crosspointLookup[X0] = X0I; 00135 crosspointLookup[X1] = X1I; 00136 crosspointLookup[X2] = X2I; 00137 crosspointLookup[X3] = X3I; 00138 crosspointLookup[X4] = X4I; 00139 crosspointLookup[X5] = X5I; 00140 crosspointLookup[X6] = X6I; 00141 crosspointLookup[X7] = X7I; 00142 crosspointLookup[X8] = X8I; 00143 crosspointLookup[X9] = X9I; 00144 crosspointLookup[X10] = X10I; 00145 crosspointLookup[X11] = X11I; 00146 crosspointLookup[X12] = X12I; 00147 crosspointLookup[X13] = X13I; 00148 crosspointLookup[X14] = X14I; 00149 crosspointLookup[X15] = X15I; 00150 crosspointLookup[Y0] = Y0I; 00151 crosspointLookup[Y1] = Y1I; 00152 crosspointLookup[Y2] = Y2I; 00153 crosspointLookup[Y3] = Y3I; 00154 crosspointLookup[Y4] = Y4I; 00155 crosspointLookup[Y5] = Y5I; 00156 crosspointLookup[Y6] = Y6I; 00157 crosspointLookup[Y7] = Y7I; 00158 } 00159 00160 ~CD22M3494() { 00161 delete xbus; 00162 delete ybus; 00163 delete data; 00164 delete strobe; 00165 delete reset; 00166 associationTable.clear(); 00167 delete &associationTable; 00168 } 00169 00170 /**Closes the switch at the given cross point. 00171 * 00172 * @param x the x axis 00173 * @param y the y axis 00174 */ 00175 bool crossPointConnect(unsigned short x, unsigned short y) { 00176 INFO("Got x point connect for x %d, y %d", x, y); 00177 if (x <= MAX_X && y <= MAX_Y) { 00178 INFO("setting data high..."); 00179 data->write(1); 00180 INFO("writing x..."); 00181 xbus->write(x); 00182 INFO("writing y..."); 00183 ybus->write(y); 00184 // let the data busses settle before setting obe high 00185 wait_us(2); 00186 strobe->write(1); 00187 // obe must be high for at least 20 ns 00188 wait_us(1); 00189 strobe->write(0); 00190 INFO("Done"); 00191 return true; 00192 } 00193 INFO("Error"); 00194 return false; 00195 } 00196 00197 /**Opens the switch at the given cross point. 00198 * 00199 * @param x the x axis 00200 * @param y the y axis 00201 */ 00202 bool crossPointDisconnect(unsigned short x, unsigned short y) { 00203 INFO("Got x point disconnect for x %d, y %d", x, y); 00204 if (x <= MAX_X && y <= MAX_Y) { 00205 INFO("setting data high..."); 00206 data->write(0); 00207 INFO("writing x..."); 00208 xbus->write(x); 00209 INFO("writing y..."); 00210 ybus->write(y); 00211 // let the data busses settle before setting obe high 00212 wait_us(2); 00213 strobe->write(1); 00214 // obe must be high for at least 20 ns 00215 wait_us(1); 00216 strobe->write(0); 00217 INFO("Done"); 00218 return true; 00219 } 00220 INFO("Error"); 00221 return false; 00222 } 00223 00224 /**Associated the two given crosspoint with the given name, note, both crosspoint MUST be on the same axis. 00225 * 00226 * @param name the name of the association 00227 * @param xp1 the first crosspoint to associate with the given name 00228 * @param xp2 the second crosspoint to associate with the given name 00229 */ 00230 bool associate(string name, string xp1, string xp2) { 00231 if (crosspointLookup.count(xp1) && crosspointLookup.count(xp2)) { 00232 Association *assoc = new Association(); 00233 assoc->xp1 = xp1; 00234 assoc->xp2 = xp2; 00235 associationTable[name] = assoc; 00236 return true; 00237 } 00238 return false; 00239 } 00240 00241 /**Connects all the crosspoints for the given associations. 00242 * 00243 * @param src the source association 00244 * @param dst the destination to connect the source to. 00245 */ 00246 bool routeAssociations(string src, string dst) { 00247 if (associationTable.count(src) && associationTable.count(dst)) { 00248 crossPointConnect(crosspointLookup[associationTable[src]->xp1], crosspointLookup[associationTable[dst]->xp1]); 00249 crossPointConnect(crosspointLookup[associationTable[src]->xp2], crosspointLookup[associationTable[dst]->xp2]); 00250 return true; 00251 } 00252 return false; 00253 } 00254 00255 /**Disconnects all the crosspoints for the given associations. 00256 * 00257 * @param src the source association 00258 * @param dst the destination to disconnect from the source. 00259 */ 00260 bool unRouteAssociations(string src, string dst) { 00261 if (associationTable.count(src) && associationTable.count(dst)) { 00262 crossPointDisconnect(crosspointLookup[associationTable[src]->xp1], crosspointLookup[associationTable[dst]->xp1]); 00263 crossPointDisconnect(crosspointLookup[associationTable[src]->xp2], crosspointLookup[associationTable[dst]->xp2]); 00264 return true; 00265 } 00266 return false; 00267 } 00268 00269 Association* getAssociation(string name) { 00270 return associationTable[name]; 00271 } 00272 00273 private: 00274 BusOut *xbus; 00275 BusOut *ybus; 00276 DigitalOut *data; 00277 DigitalOut *strobe; 00278 DigitalOut *reset; 00279 map<string, Association*> associationTable; 00280 map<string, unsigned short> crosspointLookup; 00281 }; 00282 00283 00284 #endif
Generated on Tue Jul 19 2022 04:55:40 by
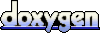