
Playing around with accelerometer and magnetometer on mbed KL46Z
Dependencies: MAG3110 MMA8451Q PinDetect mbed TSI
main.cpp
00001 #include "mbed.h" 00002 #include "PinDetect.h" 00003 #include "MMA8451Q.h" 00004 #include "MAG3110.h" 00005 #include "TSISensor.h" 00006 00007 #define MMA8451_I2C_ADDRESS (0x1d<<1) 00008 00009 // Declare output LEDs 00010 DigitalOut ledgreen(PTD5); 00011 DigitalOut ledred(PTE29); 00012 00013 // Declare USB serial connection 00014 Serial pc(USBTX,USBRX); 00015 00016 // Declare timer interrupt 00017 Ticker timerAcc; 00018 Ticker timerMag; 00019 Ticker timerLight; 00020 Ticker timerTouch; 00021 Ticker timerADC; 00022 00023 // Declare pointer variables 00024 float xAcc; 00025 float yAcc; 00026 float zAcc; 00027 int xMag; 00028 int yMag; 00029 int zMag; 00030 float xLight; 00031 float xTouch; 00032 float xADC; 00033 00034 // Sampling rates 00035 float accRate = 0.1; 00036 float magRate = 0.1; 00037 float lightRate = 0.1; 00038 float touchRate = 0.1; 00039 float adcRate = 0.1; 00040 00041 // Enables 00042 int accEn = 1; 00043 int magEn = 1; 00044 int lightEn = 1; 00045 int touchEn = 1; 00046 int adcEn = 1; 00047 00048 // Receiving Data 00049 const int bufferSize = 255; 00050 char buffer[bufferSize]; 00051 int index = 0; 00052 int received = 0; 00053 int readEn = 0; 00054 float temp; 00055 00056 // Declare Accelerometer pins and I2C address 00057 MMA8451Q acc(PTE25, PTE24, MMA8451_I2C_ADDRESS, 0, 0); 00058 // Declare Magnetometer pins 00059 MAG3110 mag(PTE25, PTE24); 00060 // Declare touch sensor pin 00061 TSISensor touch; 00062 // Declare light sensor pin 00063 AnalogIn light(PTE22); 00064 // Declare analog input pin 00065 AnalogIn adc(PTB0); 00066 00067 // Functions 00068 void init(); 00069 void printData(); 00070 void receiveHandler(); 00071 void processCommand(); 00072 void accTime(); 00073 void magTime(); 00074 void lightTime(); 00075 void touchTime(); 00076 void adcTime(); 00077 00078 void init() 00079 { 00080 // Attach timerAcc 00081 pc.baud(9600); 00082 timerAcc.attach(&accTime, accRate); 00083 timerMag.attach(&magTime, magRate); 00084 timerLight.attach(&lightTime, lightRate); 00085 timerTouch.attach(&touchTime, touchRate); 00086 timerADC.attach(&adcTime, adcRate); 00087 pc.attach(&receiveHandler, Serial::RxIrq); 00088 ledred = 0; 00089 ledgreen = 0; 00090 } 00091 00092 int main() 00093 { 00094 // Initialize 00095 init(); 00096 00097 while(1) 00098 { 00099 __disable_irq(); 00100 printData(); 00101 __enable_irq(); 00102 wait(0.05); 00103 if(received == 1){ 00104 __disable_irq(); 00105 processCommand(); 00106 __enable_irq(); 00107 } 00108 //ledgreen = !ledgreen; 00109 } 00110 } 00111 00112 void printData() 00113 { 00114 pc.printf("/%f/%f/%f/%d/%d/%d/%f/%f/%f/%.3f/%.3f/%.3f/%.3f/%.3f/%d/%d/%d/%d/%d/\r\n", 00115 xAcc, yAcc, zAcc, xMag, yMag, zMag, xLight, xTouch, xADC, 00116 accRate, magRate, lightRate, touchRate, adcRate, 00117 accEn, magEn, lightEn, touchEn, adcEn); 00118 00119 } 00120 00121 void receiveHandler() 00122 { 00123 while (pc.readable()){ 00124 temp = pc.getc(); 00125 //begin reading if char is @ 00126 if (temp == '@') 00127 { 00128 readEn = 1; 00129 } 00130 //stop reading if char is # 00131 else if (temp == '#' && readEn == 1) 00132 { 00133 readEn = 0; 00134 received = 1; 00135 //ledred = 1; 00136 index = 0; 00137 return; 00138 } 00139 // if read enable is on, then read in data 00140 else if (readEn == 1) 00141 { 00142 buffer[index] = temp; 00143 index++; 00144 } 00145 } 00146 return; 00147 } 00148 00149 void processCommand() 00150 { 00151 //pc.printf("%s\r\n", buffer); 00152 char* cmd_index; 00153 char* cmd_val; 00154 char* disStr = "ddd"; 00155 char* enStr = "eee"; 00156 // read in first value (0, 1, 2, or 3) 00157 cmd_index = strtok(buffer, "x"); 00158 //pc.printf("%s\r\n", cmd_index); 00159 //read in second value (integer larger than 050) 00160 cmd_val = strtok(NULL, "x"); 00161 //pc.printf("%s\r\n", cmd_val); 00162 // ddd means disable 00163 if (strcmp(cmd_val, disStr) == 0) 00164 { 00165 ledgreen = 1; 00166 switch(*cmd_index) 00167 { 00168 case '1': 00169 timerAcc.detach(); 00170 accEn = 0; 00171 break; 00172 case '2': 00173 timerMag.detach(); 00174 magEn = 0; 00175 break; 00176 case '3': 00177 timerLight.detach(); 00178 lightEn = 0; 00179 break; 00180 case '4': 00181 timerTouch.detach(); 00182 touchEn = 0; 00183 break; 00184 case '5': 00185 timerADC.detach(); 00186 adcEn = 0; 00187 break; 00188 default: 00189 //pc.printf("incorrect input\r\n"); 00190 break; 00191 } 00192 } 00193 // eee means enable 00194 else if (strcmp(cmd_val, enStr) == 0) 00195 { 00196 switch(*cmd_index) 00197 { 00198 case '1': 00199 timerAcc.attach(&accTime, accRate); 00200 accEn = 1; 00201 break; 00202 case '2': 00203 timerMag.attach(&magTime, magRate); 00204 magEn = 1; 00205 break; 00206 case '3': 00207 timerLight.attach(&lightTime, lightRate); 00208 lightEn = 1; 00209 break; 00210 case '4': 00211 timerTouch.attach(&touchTime, touchRate); 00212 touchEn = 1; 00213 break; 00214 case '5': 00215 timerADC.attach(&adcTime, adcRate); 00216 adcEn = 1; 00217 break; 00218 default: 00219 //pc.printf("incorrect input\r\n"); 00220 break; 00221 } 00222 } 00223 temp = strtod(cmd_val, NULL)/1000; 00224 if (temp > 0.001 && strlen(cmd_val) >= 3) 00225 { 00226 switch(*cmd_index) 00227 { 00228 case '1': 00229 accRate = temp; 00230 timerAcc.detach(); 00231 timerAcc.attach(&accTime, accRate); 00232 break; 00233 case '2': 00234 magRate = temp; 00235 timerMag.detach(); 00236 timerMag.attach(&magTime, magRate); 00237 break; 00238 case '3': 00239 lightRate = temp; 00240 timerLight.detach(); 00241 timerLight.attach(&lightTime, lightRate); 00242 break; 00243 case '4': 00244 touchRate = temp; 00245 timerTouch.detach(); 00246 timerTouch.attach(&touchTime, touchRate); 00247 break; 00248 case '5': 00249 adcRate = temp; 00250 timerADC.detach(); 00251 timerADC.attach(&adcTime, adcRate); 00252 default: 00253 //pc.printf("incorrect input\r\n"); 00254 break; 00255 } 00256 } 00257 00258 received = 0; 00259 memset(buffer, 0, bufferSize); 00260 //pc.printf("%s\r\n", buffer); 00261 } 00262 00263 void accTime() 00264 { 00265 xAcc = abs(acc.getAccX()); 00266 yAcc = abs(acc.getAccY()); 00267 zAcc = abs(acc.getAccZ()); 00268 ledred = !ledred; 00269 } 00270 00271 void magTime() 00272 { 00273 xMag = mag.getXVal(); 00274 yMag = mag.getYVal(); 00275 zMag = mag.getZVal(); 00276 //ledred = !ledred; 00277 } 00278 00279 void lightTime() 00280 { 00281 xLight = 1 - light.read(); 00282 } 00283 00284 void touchTime() 00285 { 00286 xTouch = 1 - touch.readPercentage(); 00287 } 00288 00289 void adcTime() 00290 { 00291 xADC = adc.read(); 00292 }
Generated on Wed Jul 20 2022 22:28:50 by
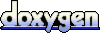