
Simple readout code for MAX110/111 14-bit ADC
Embed:
(wiki syntax)
Show/hide line numbers
main.cpp
00001 #include "mbed.h" 00002 // Use SPI port to control MAX110/111 Analog to digital converter 00003 // MAX110 is split-supply device, MAX111 single supply. 00004 // Devices require 5v for full performance, may be testable at 3.3v 00005 // 00006 // 00007 // Conversion is SLOW. 00008 // 00009 // Data is clocked in on rising edges so SPI 0,0 or SPI 1,1. 00010 00011 Serial pc(USBTX, USBRX); // tx, rx 00012 00013 SPI spi(p5, p6, p7); // mosi, miso, sclk 00014 DigitalOut max_cs(p22); 00015 DigitalIn max_busy(p23); 00016 00017 //module libmax110 00018 // 00019 //const 00020 #define max_12bit 0x9200 00021 #define max_13bit 0x8600 00022 #define max_14bit 0x8c00 00023 #define max_div4 0x0100 00024 #define max_div2 0x0080 00025 #define max_chan1 0x0000 00026 #define max_chan2 0x0010 00027 #define max_full 0x000c 00028 //#define max_gain 0b0000000000001000 00029 #define max_ofs 0x0004 00030 // 00031 // procedure max_init(dim mode as word, dim byref port as //byte, dim cs,bf as byte) 00032 // 00033 // mode must be one of max_12bit, max_13bit, max_14bit 00034 // mode may be modified by "or-ing" with max_div2 or max_div4 to set clock divider 00035 // mode may be modified by "or-ing" with max_chan1 or //max_chan2 to set input channel 00036 // mode may be modified by "or-ing" with a calibration type //max_full,max_ofs 00037 // 00038 // port is the port the busy and cs pins are connected to (doesn't have to be PORTC) 00039 // cs is the bit number for the chip select pin 00040 // bf is the bit number for the busy flag pin 00041 // 00042 // function max_read(dim byref data as integer) as boolean 00043 // 00044 // notes returns true if a value was read, immediately restarts converter 00045 // otherwise returns false 00046 // 00047 // result is integer, valid results are from -16384 through 16383. 00048 // values beyond this are overrange 00049 // 00050 // 00051 // MAX110/111 Control register bits 00052 // 15 !NOOP Set to 1 for action, 0 for pass-through 00053 // 14 NU, set to 0 00054 // 13 NU, set to 0 00055 // CONV 4,3,2,1 00056 // 12 CONV4 1 0 0 1 20ms 00057 // 11 CONV3 0 0 1 1 40ms 00058 // 10 CONV2 0 1 1 0 160ms 00059 // 9 CONV1 0 0 0 0 200ms SPECIAL 00060 // SPECIAL = NO INTERNAL GAIN CALIBRATION, FIX IN SOFTWARE 00061 // 8 DV4 1=DIVIDE XCLK BY 4 00062 // 7 DV2 1=DIVIDE XCLK BY 2 00063 // 00064 // 6 NU, set to 0 00065 // 5 NU, set to 0 00066 00067 // 4 CHS 0:CHANNEL 1 1:CHANNEL2 00068 // 00069 // CAL NUL FUNCTION 00070 // 1 1 INTERNAL ZERO (PERFORM FIRST) 00071 // 3 GAIN CAL 1 0 INTERNAL GAIN (NEXT) 00072 // 2 OFFSET NUL 0 1 INPUT NUL (LAST) 00073 // 0 0 NORMAL CONVERT 00074 // 1 PDX POWER DOWN OSC 00075 // 0 PD POWER DOWN ADC 00076 // 00077 // 00078 unsigned int max_mode; 00079 // 00080 void max_setup(unsigned int mmode) 00081 { 00082 max_mode=mmode; 00083 max_cs=0; 00084 spi.write(0); 00085 spi.write(0); 00086 max_cs=1; 00087 // not sure why the above step helps but it seems to improve restarts after random 00088 // reset 00089 max_cs=0; 00090 spi.write((max_mode & 0xff00) >> 8); 00091 spi.write(max_mode & 0xff); 00092 max_cs=1; 00093 } 00094 00095 00096 int max_read(int *data) 00097 { 00098 if (max_busy) 00099 { 00100 if (max_mode & 0x0c) 00101 { 00102 max_mode = max_mode - 0x04; 00103 00104 max_cs=0; 00105 spi.write((max_mode & 0xff00) >> 8); 00106 spi.write(max_mode & 0xff); 00107 max_cs=1; 00108 wait(0.1); 00109 return(false); 00110 } 00111 else 00112 { 00113 short t; 00114 max_cs=0; 00115 t =spi.write((max_mode & 0xff00) >> 8); 00116 t =spi.write(max_mode & 0xff) | (t<<8); 00117 *data= (int) t; 00118 max_cs=1; 00119 return(true); 00120 } 00121 } 00122 else 00123 { 00124 return(false); 00125 } 00126 } 00127 00128 00129 int main() { 00130 spi.format(8,0); 00131 spi.frequency(100000); 00132 max_cs=1; 00133 // pc.printf("Start converter\r\n"); 00134 max_setup(max_14bit | max_full); 00135 while(1) { 00136 int val; 00137 val=0; 00138 if (max_read(&val)) 00139 { 00140 pc.printf("Readout: %d \r\n",val); 00141 // optional: force a recalibrate 00142 // max_setup(max_14bit | max_full); 00143 } 00144 } 00145 }
Generated on Fri Jul 15 2022 13:55:34 by
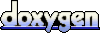