ADAFRUIT GP9002 VFD Driver, requires GFX-Library
Embed:
(wiki syntax)
Show/hide line numbers
Adafruit_GP9002.h
00001 #ifndef _ADAFRUIT_GP9002_H 00002 #define _ADAFRUIT_GP9002_H 00003 00004 #include "mbed.h" 00005 00006 #include "Adafruit_GFX.h" 00007 00008 #define BLACK 0 00009 #define WHITE 1 00010 00011 #define GP9002_DISPLAYSOFF 0x00 00012 #define GP9002_DISPLAY1ON 0x01 00013 #define GP9002_DISPLAY2ON 0x02 00014 #define GP9002_ADDRINCR 0x04 00015 #define GP9002_ADDRHELD 0x05 00016 #define GP9002_CLEARSCREEN 0x06 00017 #define GP9002_CONTROLPOWER 0x07 00018 #define GP9002_DATAWRITE 0x08 00019 #define GP9002_DATAREAD 0x09 00020 #define GP9002_LOWERADDR1 0x0A 00021 #define GP9002_HIGHERADDR1 0x0B 00022 #define GP9002_LOWERADDR2 0x0C 00023 #define GP9002_HIGHERADDR2 0x0D 00024 #define GP9002_ADDRL 0x0E 00025 #define GP9002_ADDRH 0x0F 00026 #define GP9002_OR 0x10 00027 #define GP9002_XOR 0x11 00028 #define GP9002_AND 0x12 00029 #define GP9002_BRIGHT 0x13 00030 #define GP9002_DISPLAY 0x14 00031 #define GP9002_DISPLAY_MONOCHROME 0x10 00032 #define GP9002_DISPLAY_GRAYSCALE 0x14 00033 #define GP9002_INTMODE 0x15 00034 #define GP9002_DRAWCHAR 0x20 00035 #define GP9002_CHARRAM 0x21 00036 #define GP9002_CHARSIZE 0x22 00037 #define GP9002_CHARBRIGHT 0x24 00038 00039 00040 class Adafruit_GP9002 : public Adafruit_GFX { 00041 public: 00042 /* Adafruit_GP9002(int8_t SCLK, int8_t MISO, int8_t MOSI, 00043 int8_t CS, int8_t DC); 00044 */ 00045 Adafruit_GP9002(SPI &SPIport, PinName CS, PinName DC); 00046 00047 // particular to this display 00048 void begin(void); 00049 // uint8_t slowSPIread(); 00050 // uint8_t fastSPIread(); 00051 // void slowSPIwrite(uint8_t); 00052 // void fastSPIwrite(uint8_t); 00053 00054 void command(uint8_t c); 00055 void dataWrite(uint8_t c); 00056 uint8_t dataRead(void); 00057 void setBrightness(uint8_t val); 00058 void invert(bool i); 00059 00060 void displayOn(); 00061 void displayOff(); 00062 void clearDisplay(void); 00063 00064 virtual void drawPixel(int16_t x, int16_t y, uint16_t color); 00065 virtual void drawFastVLine(int16_t x, int16_t y, int16_t h, uint16_t color); 00066 00067 private: 00068 // int8_t _miso, _mosi, _sclk, _dc, _cs; 00069 SPI _spi; 00070 DigitalOut _dc, _cs; 00071 00072 // volatile uint8_t *mosiport, *misopin, *clkport, *csport, *dcport; 00073 // uint8_t mosipinmask, misopinmask, clkpinmask, cspinmask, dcpinmask; 00074 00075 // bool hwSPI; 00076 00077 void spiwrite(uint8_t c); 00078 }; 00079 00080 #endif
Generated on Wed Jul 13 2022 18:58:28 by
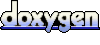