
I2CSlave test program. I2CSlave library has some improper behavior, so this program is able to watch that. The workaround for this behavior, only for NXP device, is wrote in 'I2CSlave_mod_NXP.cpp'.
main.cpp
00001 #include "mbed.h" 00002 00003 //#define I2C_SLAVE_MOD 00004 00005 #ifdef I2C_SLAVE_MOD 00006 #include "I2CSlave_mod_NXP.h" 00007 I2CSlave_mod slave(dp5, dp27); // sda, scl 00008 #else 00009 I2CSlave slave(dp5, dp27); // sda, scl 00010 #endif 00011 00012 Serial serial(dp16, dp15); // tx, rx 00013 DigitalOut led(LED1); // indicator 00014 00015 int main() { 00016 led = 0; 00017 serial.baud(115200); 00018 00019 char buf[10]; 00020 slave.address(0x80); 00021 00022 while(1) { 00023 int i = slave.receive(); 00024 switch (i) { 00025 case I2CSlave::ReadAddressed: 00026 //slave.write(msg, strlen(msg) + 1); // Includes null char 00027 led = 0; 00028 break; 00029 case I2CSlave::WriteGeneral: 00030 //slave.read(buf, 10); 00031 //printf("Read G: %s\n", buf); 00032 led = 0; 00033 break; 00034 case I2CSlave::WriteAddressed: 00035 if (slave.read(buf, 10) == 0) { 00036 // success 00037 serial.printf("Read success. DATA: %s\n", buf); 00038 led = 1; 00039 } else { 00040 // failure 00041 serial.printf("Read failure. DATA: %s\n", buf); 00042 led = 0; 00043 } 00044 break; 00045 } 00046 00047 for(int i = 0; i < 10; i++) buf[i] = 0; // Clear buffer 00048 } 00049 }
Generated on Tue Aug 16 2022 07:10:45 by
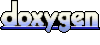