
mbed Weather Platform firmware http://mbed.org/users/okini3939/notebook/mbed-weather-platform-firmware/
Dependencies: EthernetNetIf SDHCFileSystem I2CLEDDisp Agentbed NTPClient_NetServices mbed BMP085 HTTPClient ConfigFile I2CLCD
snmp.cpp
00001 /** 00002 * Agentbed SNMP Server 00003 * Modified for mbed, 2011 Suga. 00004 * 00005 * Agentuino SNMP Agent Library Prototyping... 00006 * Copyright 2010 Eric C. Gionet <lavco_eg@hotmail.com> 00007 */ 00008 #include "mbed.h" 00009 #include "Agentbed.h" 00010 #include "EthernetNetIf.h" 00011 #include "ConfigFile.h" 00012 #include "conf.h" 00013 00014 AgentbedClass Agentbed; 00015 00016 // RFC1213-MIB OIDs 00017 // .iso (.1) 00018 // .iso.org (.1.3) 00019 // .iso.org.dod (.1.3.6) 00020 // .iso.org.dod.internet (.1.3.6.1) 00021 // .iso.org.dod.internet.mgmt (.1.3.6.1.2) 00022 // .iso.org.dod.internet.mgmt.mib-2 (.1.3.6.1.2.1) 00023 // .iso.org.dod.internet.mgmt.mib-2.system (.1.3.6.1.2.1.1) 00024 // .iso.org.dod.internet.mgmt.mib-2.system.sysDescr (.1.3.6.1.2.1.1.1) 00025 const char sysDescr[] = "1.3.6.1.2.1.1.1.0"; // read-only (DisplayString) 00026 // .iso.org.dod.internet.mgmt.mib-2.system.sysObjectID (.1.3.6.1.2.1.1.2) 00027 const char sysObjectID[] = "1.3.6.1.2.1.1.2.0"; // read-only (ObjectIdentifier) 00028 // .iso.org.dod.internet.mgmt.mib-2.system.sysUpTime (.1.3.6.1.2.1.1.3) 00029 const char sysUpTime[] = "1.3.6.1.2.1.1.3.0"; // read-only (TimeTicks) 00030 // .iso.org.dod.internet.mgmt.mib-2.system.sysContact (.1.3.6.1.2.1.1.4) 00031 const char sysContact[] = "1.3.6.1.2.1.1.4.0"; // read-write (DisplayString) 00032 // .iso.org.dod.internet.mgmt.mib-2.system.sysName (.1.3.6.1.2.1.1.5) 00033 const char sysName[] = "1.3.6.1.2.1.1.5.0"; // read-write (DisplayString) 00034 // .iso.org.dod.internet.mgmt.mib-2.system.sysLocation (.1.3.6.1.2.1.1.6) 00035 const char sysLocation[] = "1.3.6.1.2.1.1.6.0"; // read-write (DisplayString) 00036 // .iso.org.dod.internet.mgmt.mib-2.system.sysServices (.1.3.6.1.2.1.1.7) 00037 const char sysServices[] = "1.3.6.1.2.1.1.7.0"; // read-only (Integer) 00038 // 00039 // Arduino defined OIDs 00040 // .iso.org.dod.internet.private (.1.3.6.1.4) 00041 // .iso.org.dod.internet.private.enterprises (.1.3.6.1.4.1) 00042 // .iso.org.dod.internet.private.enterprises.arduino (.1.3.6.1.4.1.36582) 00043 const char enterprises[] = "1.3.6.1.4.1.36582."; // read-only (Integer) 00044 // 00045 // 00046 // RFC1213 local values 00047 static char locDescr[] = "mbed Weather Platform"; // read-only (static) 00048 static char locObjectID[] = "1.3.6.1.4.1.36582"; // read-only (static) 00049 uint32_t locUpTime = 0; // read-only (static) 00050 static char locContact[] = "<root@weather>"; // should be stored/read from EEPROM - read/write (not done for simplicity) 00051 static char locName[] = "weather.mbed"; // should be stored/read from EEPROM - read/write (not done for simplicity) 00052 static char locLocation[] = "weather"; // should be stored/read from EEPROM - read/write (not done for simplicity) 00053 static int32_t locServices = 7; // read-only (static) 00054 00055 uint32_t prevMillis = 0; 00056 char oid[SNMP_MAX_OID_LEN]; 00057 SNMP_API_STAT_CODES api_status; 00058 SNMP_ERR_CODES status; 00059 00060 extern DigitalOut led_y; 00061 extern Sensor sensor; 00062 00063 void pduReceived() 00064 { 00065 SNMP_PDU pdu; 00066 // 00067 led_y = 1; 00068 api_status = Agentbed.requestPdu(&pdu); 00069 // 00070 if ( pdu.type == SNMP_PDU_GET || pdu.type == SNMP_PDU_GET_NEXT || pdu.type == SNMP_PDU_SET 00071 && pdu.error == SNMP_ERR_NO_ERROR && api_status == SNMP_API_STAT_SUCCESS ) { 00072 // 00073 pdu.OID.toString(oid); 00074 // 00075 pdu.error = SNMP_ERR_READ_ONLY; 00076 // 00077 if ( strcmp(oid, sysDescr ) == 0 ) { 00078 // handle sysDescr (set/get) requests 00079 if ( pdu.type == SNMP_PDU_GET ) { 00080 // response packet from get-request - locDescr 00081 status = pdu.VALUE.encode(SNMP_SYNTAX_OCTETS, locDescr); 00082 pdu.error = status; 00083 } 00084 } else if ( strcmp(oid, sysObjectID ) == 0 ) { 00085 // handle sysName (set/get) requests 00086 if ( pdu.type == SNMP_PDU_GET ) { 00087 // response packet from get-request - locUpTime 00088 status = pdu.VALUE.encode(SNMP_SYNTAX_OCTETS, locObjectID); 00089 pdu.error = status; 00090 } 00091 } else if ( strcmp(oid, sysUpTime ) == 0 ) { 00092 // handle sysName (set/get) requests 00093 if ( pdu.type == SNMP_PDU_GET ) { 00094 // response packet from get-request - locUpTime 00095 status = pdu.VALUE.encode(SNMP_SYNTAX_TIME_TICKS, locUpTime); 00096 pdu.error = status; 00097 } 00098 } else if ( strcmp(oid, sysName ) == 0 ) { 00099 // handle sysName (set/get) requests 00100 if ( pdu.type == SNMP_PDU_GET ) { 00101 // response packet from get-request - locName 00102 status = pdu.VALUE.encode(SNMP_SYNTAX_OCTETS, locName); 00103 pdu.error = status; 00104 } 00105 } else if ( strcmp(oid, sysContact ) == 0 ) { 00106 // handle sysContact (set/get) requests 00107 if ( pdu.type == SNMP_PDU_GET ) { 00108 // response packet from get-request - locContact 00109 status = pdu.VALUE.encode(SNMP_SYNTAX_OCTETS, locContact); 00110 pdu.error = status; 00111 } 00112 } else if ( strcmp(oid, sysLocation ) == 0 ) { 00113 // handle sysLocation (set/get) requests 00114 if ( pdu.type == SNMP_PDU_GET ) { 00115 // response packet from get-request - locLocation 00116 status = pdu.VALUE.encode(SNMP_SYNTAX_OCTETS, locLocation); 00117 pdu.error = status; 00118 } 00119 } else if ( strcmp(oid, sysServices) == 0 ) { 00120 // handle sysServices (set/get) requests 00121 if ( pdu.type == SNMP_PDU_GET ) { 00122 // response packet from get-request - locServices 00123 status = pdu.VALUE.encode(SNMP_SYNTAX_INT, locServices); 00124 pdu.error = status; 00125 } 00126 } else if ( strncmp(oid, enterprises, strlen(enterprises)) == 0 ) { 00127 // handle enterprises (set/get) requests 00128 if ( pdu.type == SNMP_PDU_GET ) { 00129 // response packet from get-request - enterprises 00130 switch (oid[strlen(enterprises)]) { 00131 case '0': 00132 status = pdu.VALUE.encode(SNMP_SYNTAX_OPAQUE_FLOAT, sensor.pres); 00133 break; 00134 case '1': 00135 status = pdu.VALUE.encode(SNMP_SYNTAX_OPAQUE_FLOAT, sensor.temp); 00136 break; 00137 case '2': 00138 status = pdu.VALUE.encode(SNMP_SYNTAX_OPAQUE_FLOAT, sensor.humi); 00139 break; 00140 case '3': 00141 status = pdu.VALUE.encode(SNMP_SYNTAX_OPAQUE_FLOAT, sensor.anemo); 00142 break; 00143 case '4': 00144 status = pdu.VALUE.encode(SNMP_SYNTAX_OPAQUE_FLOAT, sensor.vane); 00145 break; 00146 case '5': 00147 status = pdu.VALUE.encode(SNMP_SYNTAX_OPAQUE_FLOAT, sensor.rain); 00148 break; 00149 case '6': 00150 status = pdu.VALUE.encode(SNMP_SYNTAX_OPAQUE_FLOAT, sensor.light); 00151 break; 00152 case '7': 00153 status = pdu.VALUE.encode(SNMP_SYNTAX_OPAQUE_FLOAT, sensor.uv); 00154 break; 00155 case '8': 00156 status = pdu.VALUE.encode(SNMP_SYNTAX_OPAQUE_FLOAT, sensor.moist); 00157 break; 00158 case '9': 00159 status = pdu.VALUE.encode(SNMP_SYNTAX_OPAQUE_FLOAT, sensor.temp2); 00160 break; 00161 } 00162 pdu.error = status; 00163 } 00164 } else { 00165 // oid does not exist 00166 // 00167 // response packet - object not found 00168 pdu.error = SNMP_ERR_NO_SUCH_NAME; 00169 } 00170 // 00171 pdu.type = SNMP_PDU_RESPONSE; 00172 00173 Agentbed.responsePdu(&pdu); 00174 } 00175 // 00176 Agentbed.freePdu(&pdu); 00177 // 00178 //Serial << "UDP Packet Received End.." << " RAM:" << freeMemory() << endl; 00179 }
Generated on Fri Jul 15 2022 12:55:40 by
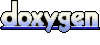