
Tiny HTTP Client http://mbed.org/users/okini3939/notebook/tinyhttp
Dependencies: EthernetNetIf mbed
main.cpp
00001 #include "mbed.h" 00002 #include "EthernetNetIf.h" 00003 #include "TCPSocket.h" 00004 #include "TinyHTTP.h" 00005 00006 DigitalOut myled(LED1); 00007 Serial pc(USBTX, USBRX); 00008 EthernetNetIf eth; 00009 00010 00011 int pachube (int feedid, char *apikey, char *buf) { 00012 Host host; 00013 char uri[40], head[160]; 00014 00015 // header 00016 snprintf(head, sizeof(head), "Content-type: text/csv\r\nX-PachubeApiKey: %s\r\n", apikey); 00017 00018 // uri 00019 snprintf(uri, sizeof(uri), "/v1/feeds/%d.csv?_method=put", feedid); 00020 00021 host.setName("api.pachube.com"); 00022 host.setPort(HTTP_PORT); 00023 return httpRequest(METHOD_POST, &host, uri, head, buf) == 200 ? 0 : -1; 00024 } 00025 00026 int twitter (char *msg, char *user, char *pwd) { 00027 Host host; 00028 char buf[300], head[160]; 00029 00030 // header 00031 createauth(user, pwd, head, sizeof(head)); 00032 strncat(head, "Content-type: application/x-www-form-urlencoded\r\n", sizeof(head) - strlen(head)); 00033 00034 // post data 00035 strcpy(buf, "status="); 00036 urlencode(msg, &buf[strlen(buf)], sizeof(buf) - strlen(buf)); 00037 00038 host.setName("api.supertweet.net"); 00039 host.setPort(HTTP_PORT); 00040 return httpRequest(METHOD_POST, &host, "/1/statuses/update.xml", head, buf) == 200 ? 0 : -1; 00041 } 00042 00043 int main () { 00044 EthernetErr ethErr; 00045 Host host; 00046 int r; 00047 00048 myled = 1; 00049 // pc.baud(115200); 00050 00051 ethErr = eth.setup(); 00052 if(ethErr) { 00053 return -1; 00054 } 00055 00056 host.setName("mbed.org"); 00057 r = httpRequest(METHOD_GET, &host, "/", NULL, NULL); 00058 00059 // r = twitter("test from #mbed TinyHTTP", "username", "password"); 00060 00061 // r = pachube(99999, "api key", "1000,30,70"); 00062 00063 /* 00064 host.setName("www.domain.name"); 00065 r = httpRequest(METHOD_POST, &host, "/xxx.cgi", "Content-Type: application/x-www-form-urlencoded\r\n", "key=value&key2=value2"); 00066 */ 00067 printf("status %d\r\n", r); 00068 00069 myled = 0; 00070 return 0; 00071 }
Generated on Wed Jul 13 2022 22:19:11 by
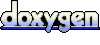