
SPI RAM 23LC1024 (Microchip) with DMA
Fork of SPIRAM_23LC1024 by
Embed:
(wiki syntax)
Show/hide line numbers
iomacros.h
00001 /* 00002 Copyright (c) 2011 Andy Kirkham 00003 00004 Permission is hereby granted, free of charge, to any person obtaining a copy 00005 of this software and associated documentation files (the "Software"), to deal 00006 in the Software without restriction, including without limitation the rights 00007 to use, copy, modify, merge, publish, distribute, sublicense, and/or sell 00008 copies of the Software, and to permit persons to whom the Software is 00009 furnished to do so, subject to the following conditions: 00010 00011 The above copyright notice and this permission notice shall be included in 00012 all copies or substantial portions of the Software. 00013 00014 THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR 00015 IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, 00016 FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE 00017 AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER 00018 LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, 00019 OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN 00020 THE SOFTWARE. 00021 */ 00022 00023 #ifndef IOMACROS_H 00024 #define IOMACROS_H 00025 00026 #ifndef __LPC17xx_H__ 00027 #include "LPC17xx.h" 00028 #endif 00029 00030 #define PIN_PULLUP 0UL 00031 #define PIN_REPEAT 1UL 00032 #define PIN_NONE 2UL 00033 #define PIN_PULLDOWN 3UL 00034 00035 /* p5 is P0.9 */ 00036 #define p5_SEL_MASK ~(3UL << 18) 00037 #define p5_SET_MASK (1UL << 9) 00038 #define p5_CLR_MASK ~(p5_SET_MASK) 00039 #define p5_AS_OUTPUT LPC_PINCON->PINSEL0&=p5_SEL_MASK;LPC_GPIO0->FIODIR|=p5_SET_MASK 00040 #define p5_AS_INPUT LPC_GPIO0->FIOMASK &= p5_CLR_MASK; 00041 #define p5_SET LPC_GPIO0->FIOSET = p5_SET_MASK 00042 #define p5_CLR LPC_GPIO0->FIOCLR = p5_SET_MASK 00043 #define p5_IS_SET (bool)(LPC_GPIO0->FIOPIN & p5_SET_MASK) 00044 #define p5_IS_CLR !(p5_IS_SET) 00045 #define p5_MODE(x) LPC_PINCON->PINMODE0&=p5_SEL_MASK;LPC_PINCON->PINMODE0|=((x&0x3)<<18) 00046 00047 /* p6 is P0.8 */ 00048 #define p6_SEL_MASK ~(3UL << 16) 00049 #define p6_SET_MASK (1UL << 8) 00050 #define p6_CLR_MASK ~(p6_SET_MASK) 00051 #define p6_AS_OUTPUT LPC_PINCON->PINSEL0&=p6_SEL_MASK;LPC_GPIO0->FIODIR|=p6-SET_MASK 00052 #define p6_AS_INPUT LPC_GPIO0->FIOMASK &= p6_CLR_MASK; 00053 #define p6_SET LPC_GPIO0->FIOSET = p6_SET_MASK 00054 #define p6_CLR LPC_GPIO0->FIOCLR = p6_SET_MASK 00055 #define p6_IS_SET (bool)(LPC_GPIO0->FIOPIN & p6_SET_MASK) 00056 #define p6_IS_CLR !(p6_IS_SET) 00057 #define p6_MODE(x) LPC_PINCON->PINMODE0&=p6_SEL_MASK;LPC_PINCON->PINMODE0|=((x&0x3)<<16) 00058 00059 /* p7 is P0.7 */ 00060 #define p7_SEL_MASK ~(3UL << 14) 00061 #define p7_SET_MASK (1UL << 7) 00062 #define p7_CLR_MASK ~(p7_SET_MASK) 00063 #define p7_AS_OUTPUT LPC_PINCON->PINSEL0&=p7_SEL_MASK;LPC_GPIO0->FIODIR|=p7_SET_MASK 00064 #define p7_AS_INPUT LPC_GPIO0->FIOMASK &= p7_CLR_MASK; 00065 #define p7_SET LPC_GPIO0->FIOSET = p7_SET_MASK 00066 #define p7_CLR LPC_GPIO0->FIOCLR = p7_SET_MASK 00067 #define p7_IS_SET (bool)(LPC_GPIO0->FIOPIN & p7_SET_MASK) 00068 #define p7_IS_CLR !(p7_IS_SET) 00069 #define p7_MODE(x) LPC_PINCON->PINMODE0&=p7_SEL_MASK;LPC_PINCON->PINMODE0|=((x&0x3)<<14) 00070 00071 /* p8 is P0.6 */ 00072 #define p8_SEL_MASK ~(3UL << 12) 00073 #define p8_SET_MASK (1UL << 6) 00074 #define p8_CLR_MASK ~(p8_SET_MASK) 00075 #define p8_AS_OUTPUT LPC_PINCON->PINSEL0&=p8_SEL_MASK;LPC_GPIO0->FIODIR|=p8_SET_MASK 00076 #define p8_AS_INPUT LPC_GPIO0->FIOMASK &= p8_CLR_MASK; 00077 #define p8_SET LPC_GPIO0->FIOSET = p8_SET_MASK 00078 #define p8_CLR LPC_GPIO0->FIOCLR = p8_SET_MASK 00079 #define p8_IS_SET (bool)(LPC_GPIO0->FIOPIN & p8_SET_MASK) 00080 #define p8_IS_CLR !(p8_IS_SET) 00081 #define p8_MODE(x) LPC_PINCON->PINMODE0&=p8_SEL_MASK;LPC_PINCON->PINMODE0|=((x&0x3)<<12) 00082 00083 /* p9 is P0.0 */ 00084 #define p9_SEL_MASK ~(3UL << 0) 00085 #define p9_SET_MASK (1UL << 0) 00086 #define p9_CLR_MASK ~(p9_SET_MASK) 00087 #define p9_AS_OUTPUT LPC_PINCON->PINSEL0&=p9_SEL_MASK;LPC_GPIO0->FIODIR|=p9_SET_MASK 00088 #define p9_AS_INPUT LPC_GPIO0->FIOMASK &= p9_CLR_MASK; 00089 #define p9_SET LPC_GPIO0->FIOSET = p9_SET_MASK 00090 #define p9_CLR LPC_GPIO0->FIOCLR = p9_SET_MASK 00091 #define p9_IS_SET (bool)(LPC_GPIO0->FIOPIN & p9_SET_MASK) 00092 #define p9_IS_CLR !(p9_IS_SET) 00093 #define p9_MODE(x) LPC_PINCON->PINMODE0&=p9_SEL_MASK;LPC_PINCON->PINMODE0|=((x&0x3)<<0) 00094 00095 /* p10 is P0.1 */ 00096 #define p10_SEL_MASK ~(3UL << 2) 00097 #define p10_SET_MASK (1UL << 1) 00098 #define p10_CLR_MASK ~(p10_SET_MASK) 00099 #define p10_AS_OUTPUT LPC_PINCON->PINSEL0&=p10_SEL_MASK;LPC_GPIO0->FIODIR|=p10_SET_MASK 00100 #define p10_AS_INPUT LPC_GPIO0->FIOMASK &= p10_CLR_MASK; 00101 #define p10_SET LPC_GPIO0->FIOSET = p10_SET_MASK 00102 #define p10_CLR LPC_GPIO0->FIOCLR = p10_SET_MASK 00103 #define p10_IS_SET (bool)(LPC_GPIO0->FIOPIN & p10_SET_MASK) 00104 #define p10_IS_CLR !(p10_IS_SET) 00105 #define p10_MODE(x) LPC_PINCON->PINMODE0&=p10_SEL_MASK;LPC_PINCON->PINMODE0|=((x&0x3)<<2) 00106 00107 /* p11 is P0.18 */ 00108 #define p11_SEL_MASK ~(3UL << 4) 00109 #define p11_SET_MASK (1UL << 18) 00110 #define p11_CLR_MASK ~(p11_SET_MASK) 00111 #define p11_AS_OUTPUT LPC_PINCON->PINSEL1&=p11_SEL_MASK;LPC_GPIO0->FIODIR|=p11_SET_MASK 00112 #define p11_AS_INPUT LPC_GPIO0->FIOMASK &= p11_CLR_MASK; 00113 #define p11_SET LPC_GPIO0->FIOSET = p11_SET_MASK 00114 #define p11_CLR LPC_GPIO0->FIOCLR = p11_SET_MASK 00115 #define p11_IS_SET (bool)(LPC_GPIO0->FIOPIN & p11_SET_MASK) 00116 #define p11_IS_CLR !(p11_IS_SET) 00117 #define p11_MODE(x) LPC_PINCON->PINMODE1&=p11_SEL_MASK;LPC_PINCON->PINMODE1|=((x&0x3)<<4) 00118 00119 /* p12 is P0.17 */ 00120 #define p12_SEL_MASK ~(3UL << 2) 00121 #define p12_SET_MASK (1UL << 17) 00122 #define p12_CLR_MASK ~(p12_SET_MASK) 00123 #define p12_AS_OUTPUT LPC_PINCON->PINSEL1&=p12_SEL_MASK;LPC_GPIO0->FIODIR|=p12_SET_MASK 00124 #define p12_AS_INPUT LPC_GPIO0->FIOMASK &= p12_CLR_MASK; 00125 #define p12_SET LPC_GPIO0->FIOSET = p12_SET_MASK 00126 #define p12_CLR LPC_GPIO0->FIOCLR = p12_SET_MASK 00127 #define p12_IS_SET (bool)(LPC_GPIO0->FIOPIN & p12_SET_MASK) 00128 #define p12_IS_CLR !(p12_IS_SET) 00129 #define p12_MODE(x) LPC_PINCON->PINMODE1&=p12_SEL_MASK;LPC_PINCON->PINMODE1|=((x&0x3)<<2) 00130 00131 /* p13 is P0.15 */ 00132 #define p13_SEL_MASK ~(3UL << 30) 00133 #define p13_SET_MASK (1UL << 15) 00134 #define p13_CLR_MASK ~(p13_SET_MASK) 00135 #define p13_AS_OUTPUT LPC_PINCON->PINSEL0&=p13_SEL_MASK;LPC_GPIO0->FIODIR|=p13_SET_MASK 00136 #define p13_AS_INPUT LPC_GPIO0->FIOMASK &= p13_CLR_MASK; 00137 #define p13_SET LPC_GPIO0->FIOSET = p13_SET_MASK 00138 #define p13_CLR LPC_GPIO0->FIOCLR = p13_SET_MASK 00139 #define p13_IS_SET (bool)(LPC_GPIO0->FIOPIN & p13_SET_MASK) 00140 #define p13_IS_CLR !(p13_IS_SET) 00141 #define p13_MODE(x) LPC_PINCON->PINMODE0&=p13_SEL_MASK;LPC_PINCON->PINMODE0|=((x&0x3)<<30) 00142 00143 /* p14 is P0.16 */ 00144 #define p14_SEL_MASK ~(3UL << 0) 00145 #define p14_SET_MASK (1UL << 16) 00146 #define p14_CLR_MASK ~(p14_SET_MASK) 00147 #define p14_AS_OUTPUT LPC_PINCON->PINSEL1&=p14_SEL_MASK;LPC_GPIO0->FIODIR|=p14_SET_MASK 00148 #define p14_AS_INPUT LPC_GPIO0->FIOMASK &= p14_CLR_MASK; 00149 #define p14_SET LPC_GPIO0->FIOSET = p14_SET_MASK 00150 #define p14_CLR LPC_GPIO0->FIOCLR = p14_SET_MASK 00151 #define p14_IS_SET (bool)(LPC_GPIO0->FIOPIN & p14_SET_MASK) 00152 #define p14_IS_CLR !(p14_IS_SET) 00153 #define p14_MODE(x) LPC_PINCON->PINMODE1&=p14_SEL_MASK;LPC_PINCON->PINMODE1|=((x&0x3)<<0) 00154 00155 /* p15 is P0.23 */ 00156 #define p15_SEL_MASK ~(3UL << 14) 00157 #define p15_SET_MASK (1UL << 23) 00158 #define p15_CLR_MASK ~(p15_SET_MASK) 00159 #define p15_AS_OUTPUT LPC_PINCON->PINSEL1&=p15_SEL_MASK;LPC_GPIO0->FIODIR|=p15_SET_MASK 00160 #define p15_AS_INPUT LPC_GPIO0->FIOMASK &= p15_CLR_MASK; 00161 #define p15_SET LPC_GPIO0->FIOSET = p15_SET_MASK 00162 #define p15_CLR LPC_GPIO0->FIOCLR = p15_SET_MASK 00163 #define p15_IS_SET (bool)(LPC_GPIO0->FIOPIN & p15_SET_MASK) 00164 #define p15_IS_CLR !(p15_IS_SET) 00165 #define p15_MODE(x) LPC_PINCON->PINMODE1&=p15_SEL_MASK;LPC_PINCON->PINMODE1|=((x&0x3)<<14) 00166 00167 /* p16 is P0.24 */ 00168 #define p16_SEL_MASK ~(3UL << 16) 00169 #define p16_SET_MASK (1UL << 24) 00170 #define p16_CLR_MASK ~(p16_SET_MASK) 00171 #define p16_AS_OUTPUT LPC_PINCON->PINSEL1&=p16_SEL_MASK;LPC_GPIO0->FIODIR|=p16_SET_MASK 00172 #define p16_AS_INPUT LPC_GPIO0->FIOMASK &= p16_CLR_MASK; 00173 #define p16_SET LPC_GPIO0->FIOSET = p16_SET_MASK 00174 #define p16_CLR LPC_GPIO0->FIOCLR = p16_SET_MASK 00175 #define p16_IS_SET (bool)(LPC_GPIO0->FIOPIN & p16_SET_MASK) 00176 #define p16_IS_CLR !(p16_IS_SET) 00177 #define p16_MODE(x) LPC_PINCON->PINMODE1&=p16_SEL_MASK;LPC_PINCON->PINMODE1|=((x&0x3)<<16) 00178 00179 /* p17 is P0.25 */ 00180 #define p17_SEL_MASK ~(3UL << 18) 00181 #define p17_SET_MASK (1UL << 25) 00182 #define p17_CLR_MASK ~(p17_SET_MASK) 00183 #define p17_AS_OUTPUT LPC_PINCON->PINSEL1&=p17_SEL_MASK;LPC_GPIO0->FIODIR|=p17_SET_MASK 00184 #define p17_AS_INPUT LPC_GPIO0->FIOMASK &= p17_CLR_MASK; 00185 #define p17_SET LPC_GPIO0->FIOSET = p17_SET_MASK 00186 #define p17_CLR LPC_GPIO0->FIOCLR = p17_SET_MASK 00187 #define p17_IS_SET (bool)(LPC_GPIO0->FIOPIN & p17_SET_MASK) 00188 #define p17_IS_CLR !(p17_IS_SET) 00189 #define p17_MODE(x) LPC_PINCON->PINMODE1&=p17_SEL_MASK;LPC_PINCON->PINMODE1|=((x&0x3)<<18) 00190 00191 /* p18 is P0.26 */ 00192 #define p18_SEL_MASK ~(3UL << 20) 00193 #define p18_SET_MASK (1UL << 26) 00194 #define p18_CLR_MASK ~(p18_SET_MASK) 00195 #define p18_AS_OUTPUT LPC_PINCON->PINSEL1&=p18_SEL_MASK;LPC_GPIO0->FIODIR|=p18_SET_MASK 00196 #define p18_AS_INPUT LPC_GPIO0->FIOMASK &= p18_CLR_MASK; 00197 #define p18_SET LPC_GPIO0->FIOSET = p18_SET_MASK 00198 #define p18_CLR LPC_GPIO0->FIOCLR = p18_SET_MASK 00199 #define p18_IS_SET (bool)(LPC_GPIO0->FIOPIN & p18_SET_MASK) 00200 #define p18_IS_CLR !(p18_IS_SET) 00201 #define p18_MODE(x) LPC_PINCON->PINMODE1&=p18_SEL_MASK;LPC_PINCON->PINMODE1|=((x&0x3)<<20) 00202 00203 /* p19 is P1.30 */ 00204 #define p19_SEL_MASK ~(3UL << 28) 00205 #define p19_SET_MASK (1UL << 30) 00206 #define p19_AS_OUTPUT LPC_PINCON->PINSEL3&=p19_SEL_MASK;LPC_GPIO1->FIODIR|=p19_SET_MASK 00207 #define p19_AS_INPUT LPC_GPIO1->FIOMASK &= p19_CLR_MASK; 00208 #define p19_SET LPC_GPIO1->FIOSET = p19_SET_MASK 00209 #define p19_CLR LPC_GPIO1->FIOCLR = p19_SET_MASK 00210 #define p19_IS_SET (bool)(LPC_GPIO1->FIOPIN & p19_SET_MASK) 00211 #define p19_IS_CLR !(p19_IS_SET) 00212 #define p19_MODE(x) LPC_PINCON->PINMODE3&=p19_SEL_MASK;LPC_PINCON->PINMODE3|=((x&0x3)<<28) 00213 00214 /* p20 is P1.31 */ 00215 #define p20_SEL_MASK ~(3UL << 30) 00216 #define p20_SET_MASK (1UL << 31) 00217 #define p20_CLR_MASK ~(p20_SET_MASK) 00218 #define p20_AS_OUTPUT LPC_PINCON->PINSEL3&=p20_SEL_MASK;LPC_GPIO1->FIODIR|=p20_SET_MASK 00219 #define p20_AS_INPUT LPC_GPIO1->FIOMASK &= p20_CLR_MASK; 00220 #define p20_SET LPC_GPIO1->FIOSET = p20_SET_MASK 00221 #define p20_CLR LPC_GPIO1->FIOCLR = p20_SET_MASK 00222 #define p20_IS_SET (bool)(LPC_GPIO1->FIOPIN & p20_SET_MASK) 00223 #define p20_IS_CLR !(p20_IS_SET) 00224 #define p20_MODE(x) LPC_PINCON->PINMODE3&=p20_SEL_MASK;LPC_PINCON->PINMODE3|=((x&0x3)<<30) 00225 00226 /* p21 is P2.5 */ 00227 #define p21_SEL_MASK ~(3UL << 10) 00228 #define p21_SET_MASK (1UL << 5) 00229 #define p21_CLR_MASK ~(p21_SET_MASK) 00230 #define p21_AS_OUTPUT LPC_PINCON->PINSEL4&=p21_SEL_MASK;LPC_GPIO2->FIODIR|=p21_SET_MASK 00231 #define p21_AS_INPUT LPC_GPIO2->FIOMASK &= p21_CLR_MASK; 00232 #define p21_SET LPC_GPIO2->FIOSET = p21_SET_MASK 00233 #define p21_CLR LPC_GPIO2->FIOCLR = p21_SET_MASK 00234 #define p21_IS_SET (bool)(LPC_GPIO2->FIOPIN & p21_SET_MASK) 00235 #define p21_IS_CLR !(p21_IS_SET) 00236 #define p21_TOGGLE p21_IS_SET?p21_CLR:p21_SET 00237 #define p21_MODE(x) LPC_PINCON->PINMODE4&=p21_SEL_MASK;LPC_PINCON->PINMODE4|=((x&0x3)<<10) 00238 00239 /* p22 is P2.4 */ 00240 #define p22_SEL_MASK ~(3UL << 8) 00241 #define p22_SET_MASK (1UL << 4) 00242 #define p22_CLR_MASK ~(p22_SET_MASK) 00243 #define p22_AS_OUTPUT LPC_PINCON->PINSEL4&=p22_SEL_MASK;LPC_GPIO2->FIODIR|=p22_SET_MASK 00244 #define p22_AS_INPUT LPC_GPIO2->FIOMASK &= p22_CLR_MASK; 00245 #define p22_SET LPC_GPIO2->FIOSET = p22_SET_MASK 00246 #define p22_CLR LPC_GPIO2->FIOCLR = p22_SET_MASK 00247 #define p22_IS_SET (bool)(LPC_GPIO2->FIOPIN & p22_SET_MASK) 00248 #define p22_IS_CLR !(p22_IS_SET) 00249 #define p22_TOGGLE p22_IS_SET?p22_CLR:p22_SET 00250 #define p22_MODE(x) LPC_PINCON->PINMODE4&=p22_SEL_MASK;LPC_PINCON->PINMODE4|=((x&0x3)<<8) 00251 00252 /* p23 is P2.3 */ 00253 #define p23_SEL_MASK ~(3UL << 6) 00254 #define p23_SET_MASK (1UL << 3) 00255 #define p23_CLR_MASK ~(p23_SET_MASK) 00256 #define p23_AS_OUTPUT LPC_PINCON->PINSEL4&=p23_SEL_MASK;LPC_GPIO2->FIODIR|=p23_SET_MASK 00257 #define p23_AS_INPUT LPC_GPIO2->FIOMASK &= p23_CLR_MASK; 00258 #define p23_SET LPC_GPIO2->FIOSET = p23_SET_MASK 00259 #define p23_CLR LPC_GPIO2->FIOCLR = p23_SET_MASK 00260 #define p23_IS_SET (bool)(LPC_GPIO2->FIOPIN & p23_SET_MASK) 00261 #define p23_IS_CLR !(p23_IS_SET) 00262 #define p23_TOGGLE p23_IS_SET?p23_CLR:p23_SET 00263 #define p23_MODE(x) LPC_PINCON->PINMODE4&=p23_SEL_MASK;LPC_PINCON->PINMODE4|=((x&0x3)<<6) 00264 00265 /* p24 is P2.2 */ 00266 #define p24_SEL_MASK ~(3UL << 4) 00267 #define p24_SET_MASK (1UL << 2) 00268 #define p24_CLR_MASK ~(p24_SET_MASK) 00269 #define p24_AS_OUTPUT LPC_PINCON->PINSEL4&=p24_SEL_MASK;LPC_GPIO2->FIODIR|=p24_SET_MASK 00270 #define p24_AS_INPUT LPC_GPIO2->FIOMASK &= p24_CLR_MASK; 00271 #define p24_SET LPC_GPIO2->FIOSET = p24_SET_MASK 00272 #define p24_CLR LPC_GPIO2->FIOCLR = p24_SET_MASK 00273 #define p24_IS_SET (bool)(LPC_GPIO2->FIOPIN & p24_SET_MASK) 00274 #define p24_IS_CLR !(p24_IS_SET) 00275 #define p24_TOGGLE p24_IS_SET?p24_CLR:p24_SET 00276 #define p24_MODE(x) LPC_PINCON->PINMODE4&=p24_SEL_MASK;LPC_PINCON->PINMODE4|=((x&0x3)<<4) 00277 00278 /* p25 is P2.1 */ 00279 #define p25_SEL_MASK ~(3UL << 2) 00280 #define p25_SET_MASK (1UL << 1) 00281 #define p25_CLR_MASK ~(p25_SET_MASK) 00282 #define p25_AS_OUTPUT LPC_PINCON->PINSEL4&=p25_SEL_MASK;LPC_GPIO2->FIODIR|=p25_SET_MASK 00283 #define p25_AS_INPUT LPC_GPIO2->FIOMASK &= p25_CLR_MASK; 00284 #define p25_SET LPC_GPIO2->FIOSET = p25_SET_MASK 00285 #define p25_CLR LPC_GPIO2->FIOCLR = p25_SET_MASK 00286 #define p25_IS_SET (bool)(LPC_GPIO2->FIOPIN & p25_SET_MASK) 00287 #define p25_IS_CLR !(p25_IS_SET) 00288 #define p25_MODE(x) LPC_PINCON->PINMODE4&=p25_SEL_MASK;LPC_PINCON->PINMODE4|=((x&0x3)<<2) 00289 00290 /* p26 is P2.0 */ 00291 #define p26_SEL_MASK ~(3UL << 0) 00292 #define p26_SET_MASK (1UL << 0) 00293 #define p26_CLR_MASK ~(p26_SET_MASK) 00294 #define p26_AS_OUTPUT LPC_PINCON->PINSEL4&=p26_SEL_MASK;LPC_GPIO2->FIODIR|=p26_SET_MASK 00295 #define p26_AS_INPUT LPC_GPIO2->FIOMASK &= p26_CLR_MASK; 00296 #define p26_SET LPC_GPIO2->FIOSET = p26_SET_MASK 00297 #define p26_CLR LPC_GPIO2->FIOCLR = p26_SET_MASK 00298 #define p26_IS_SET (bool)(LPC_GPIO2->FIOPIN & p26_SET_MASK) 00299 #define p26_IS_CLR !(p26_IS_SET) 00300 #define p26_MODE(x) LPC_PINCON->PINMODE4&=p26_SEL_MASK;LPC_PINCON->PINMODE4|=((x&0x3)<<0) 00301 00302 /* p27 is P0.11 */ 00303 #define p27_SEL_MASK ~(3UL << 22) 00304 #define p27_SET_MASK (1UL << 11) 00305 #define p27_CLR_MASK ~(p27_SET_MASK) 00306 #define p27_AS_OUTPUT LPC_PINCON->PINSEL0&=p27_SEL_MASK;LPC_GPIO0->FIODIR|=p27_SET_MASK 00307 #define p27_AS_INPUT LPC_GPIO0->FIOMASK &= p27_CLR_MASK; 00308 #define p27_SET LPC_GPIO0->FIOSET = p27_SET_MASK 00309 #define p27_CLR LPC_GPIO0->FIOCLR = p27_SET_MASK 00310 #define p27_IS_SET (bool)(LPC_GPIO0->FIOPIN & p27_SET_MASK) 00311 #define p27_IS_CLR !(p27_IS_SET) 00312 #define p27_MODE(x) LPC_PINCON->PINMODE0&=p27_SEL_MASK;LPC_PINCON->PINMODE0|=((x&0x3)<<22) 00313 00314 /* p28 is P0.10 */ 00315 #define p28_SEL_MASK ~(3UL << 20) 00316 #define p28_SET_MASK (1UL << 10) 00317 #define p28_CLR_MASK ~(p28_SET_MASK) 00318 #define p28_AS_OUTPUT LPC_PINCON->PINSEL0&=p28_SEL_MASK;LPC_GPIO0->FIODIR|=p28_SET_MASK 00319 #define p28_AS_INPUT LPC_GPIO0->FIOMASK &= p28_CLR_MASK; 00320 #define p28_SET LPC_GPIO0->FIOSET = p28_SET_MASK 00321 #define p28_CLR LPC_GPIO0->FIOCLR = p28_SET_MASK 00322 #define p28_IS_SET (bool)(LPC_GPIO0->FIOPIN & p28_SET_MASK) 00323 #define p28_IS_CLR !(p28_IS_SET) 00324 #define p28_MODE(x) LPC_PINCON->PINMODE0&=p28_SEL_MASK;LPC_PINCON->PINMODE0|=((x&0x3)<<20) 00325 00326 /* p29 is P0.5 */ 00327 #define p29_SEL_MASK ~(3UL << 10) 00328 #define p29_SET_MASK (1UL << 5) 00329 #define p29_CLR_MASK ~(p29_SET_MASK) 00330 #define p29_AS_OUTPUT LPC_PINCON->PINSEL0&=p29_SEL_MASK;LPC_GPIO0->FIODIR|=p29_SET_MASK 00331 #define p29_AS_INPUT LPC_GPIO0->FIOMASK &= p29_CLR_MASK; 00332 #define p29_SET LPC_GPIO0->FIOSET = p29_SET_MASK 00333 #define p29_CLR LPC_GPIO0->FIOCLR = p29_SET_MASK 00334 #define p29_IS_SET (bool)(LPC_GPIO0->FIOPIN & p29_SET_MASK) 00335 #define p29_IS_CLR !(p29_IS_SET) 00336 #define p29_TOGGLE p29_IS_SET?p29_CLR:p29_SET 00337 #define p29_MODE(x) LPC_PINCON->PINMODE0&=p29_SEL_MASK;LPC_PINCON->PINMODE0|=((x&0x3)<<10) 00338 00339 /* p30 is P0.4 */ 00340 #define p30_SEL_MASK ~(3UL << 8) 00341 #define p30_SET_MASK (1UL << 4) 00342 #define p30_CLR_MASK ~(p30_SET_MASK) 00343 #define p30_AS_OUTPUT LPC_PINCON->PINSEL0&=p30_SEL_MASK;LPC_GPIO0->FIODIR|=p30_SET_MASK 00344 #define p30_AS_INPUT LPC_GPIO0->FIOMASK &= p30_CLR_MASK; 00345 #define p30_SET LPC_GPIO0->FIOSET = p30_SET_MASK 00346 #define p30_CLR LPC_GPIO0->FIOCLR = p30_SET_MASK 00347 #define p30_IS_SET (bool)(LPC_GPIO0->FIOPIN & p30_SET_MASK) 00348 #define p30_IS_CLR !(p30_IS_SET) 00349 #define p30_MODE(x) LPC_PINCON->PINMODE0&=p30_SEL_MASK;LPC_PINCON->PINMODE0|=((x&0x3)<<8) 00350 00351 /* The following definitions are for the four Mbed LEDs. 00352 LED1 = P1.18 00353 LED2 = P1.20 00354 LED3 = P1.21 00355 LED4 = P1.23 */ 00356 00357 #define P1_18_SEL_MASK ~(3UL << 4) 00358 #define P1_18_SET_MASK (1UL << 18) 00359 #define P1_18_CLR_MASK ~(P1_18_SET_MASK) 00360 #define P1_18_AS_OUTPUT LPC_PINCON->PINSEL3&=P1_18_SEL_MASK;LPC_GPIO1->FIODIR|=P1_18_SET_MASK 00361 #define P1_18_AS_INPUT LPC_GPIO1->FIOMASK &= P1_18_CLR_MASK; 00362 #define P1_18_SET LPC_GPIO1->FIOSET = P1_18_SET_MASK 00363 #define P1_18_CLR LPC_GPIO1->FIOCLR = P1_18_SET_MASK 00364 #define P1_18_IS_SET (bool)(LPC_GPIO1->FIOPIN & P1_18_SET_MASK) 00365 #define P1_18_IS_CLR !(P1_18_IS_SET) 00366 #define LED1_USE P1_18_AS_OUTPUT;P1_18_AS_INPUT 00367 #define LED1_ON P1_18_SET 00368 #define LED1_OFF P1_18_CLR 00369 #define LED1_IS_ON P1_18_IS_SET 00370 #define LED1_TOGGLE P1_18_IS_SET?LED1_OFF:LED1_ON 00371 00372 #define P1_20_SEL_MASK ~(3UL << 8) 00373 #define P1_20_SET_MASK (1UL << 20) 00374 #define P1_20_CLR_MASK ~(P1_20_SET_MASK) 00375 #define P1_20_AS_OUTPUT LPC_PINCON->PINSEL3&=P1_20_SEL_MASK;LPC_GPIO1->FIODIR|=P1_20_SET_MASK 00376 #define P1_20_AS_INPUT LPC_GPIO1->FIOMASK &= P1_20_CLR_MASK; 00377 #define P1_20_SET LPC_GPIO1->FIOSET = P1_20_SET_MASK 00378 #define P1_20_CLR LPC_GPIO1->FIOCLR = P1_20_SET_MASK 00379 #define P1_20_IS_SET (bool)(LPC_GPIO1->FIOPIN & P1_20_SET_MASK) 00380 #define P1_20_IS_CLR !(P1_20_IS_SET) 00381 #define LED2_USE P1_20_AS_OUTPUT;P1_20_AS_INPUT 00382 #define LED2_ON P1_20_SET 00383 #define LED2_OFF P1_20_CLR 00384 #define LED2_IS_ON P1_20_IS_SET 00385 #define LED2_TOGGLE P1_20_IS_SET?LED2_OFF:LED2_ON 00386 00387 #define P1_21_SEL_MASK ~(3UL << 10) 00388 #define P1_21_SET_MASK (1UL << 21) 00389 #define P1_21_CLR_MASK ~(P1_21_SET_MASK) 00390 #define P1_21_AS_OUTPUT LPC_PINCON->PINSEL3&=P1_21_SEL_MASK;LPC_GPIO1->FIODIR|=P1_21_SET_MASK 00391 #define P1_21_AS_INPUT LPC_GPIO1->FIOMASK &= P1_21_CLR_MASK; 00392 #define P1_21_SET LPC_GPIO1->FIOSET = P1_21_SET_MASK 00393 #define P1_21_CLR LPC_GPIO1->FIOCLR = P1_21_SET_MASK 00394 #define P1_21_IS_SET (bool)(LPC_GPIO1->FIOPIN & P1_21_SET_MASK) 00395 #define P1_21_IS_CLR !(P1_21_IS_SET) 00396 #define LED3_USE P1_21_AS_OUTPUT;P1_21_AS_INPUT 00397 #define LED3_ON P1_21_SET 00398 #define LED3_OFF P1_21_CLR 00399 #define LED3_IS_ON P1_21_IS_SET 00400 #define LED3_TOGGLE P1_21_IS_SET?LED3_OFF:LED3_ON 00401 00402 #define P1_23_SEL_MASK ~(3UL << 14) 00403 #define P1_23_SET_MASK (1UL << 23) 00404 #define P1_23_CLR_MASK ~(P1_23_SET_MASK) 00405 #define P1_23_AS_OUTPUT LPC_PINCON->PINSEL3&=P1_23_SEL_MASK;LPC_GPIO1->FIODIR|=P1_23_SET_MASK 00406 #define P1_23_AS_INPUT LPC_GPIO1->FIOMASK &= P1_23_CLR_MASK; 00407 #define P1_23_SET LPC_GPIO1->FIOSET = P1_23_SET_MASK 00408 #define P1_23_CLR LPC_GPIO1->FIOCLR = P1_23_SET_MASK 00409 #define P1_23_IS_SET (bool)(LPC_GPIO1->FIOPIN & P1_23_SET_MASK) 00410 #define P1_23_IS_CLR !(P1_23_IS_SET) 00411 #define LED4_USE P1_23_AS_OUTPUT;P1_23_AS_INPUT 00412 #define LED4_ON P1_23_SET 00413 #define LED4_OFF P1_23_CLR 00414 #define LED4_IS_ON P1_23_IS_SET 00415 #define LED4_TOGGLE P1_23_IS_SET?LED4_OFF:LED4_ON 00416 00417 #endif 00418
Generated on Wed Jul 13 2022 17:00:38 by
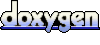