
SNMP Agent http://mbed.org/users/okini3939/notebook/agentbed-library/
Dependencies: EthernetNetIf mbed
main.cpp
00001 /** 00002 * Agentbed library (SNMP) 00003 * Modified for mbed, 2010 Suga. 00004 * 00005 * Agentuino SNMP Agent Library Prototyping... 00006 * 00007 * Copyright 2010 Eric C. Gionet <lavco_eg@hotmail.com> 00008 * 00009 */ 00010 #include "mbed.h" 00011 #include "Agentbed.h" 00012 #include "EthernetNetIf.h" 00013 00014 EthernetNetIf eth; 00015 AgentbedClass Agentbed; 00016 00017 // RFC1213-MIB OIDs 00018 // .iso (.1) 00019 // .iso.org (.1.3) 00020 // .iso.org.dod (.1.3.6) 00021 // .iso.org.dod.internet (.1.3.6.1) 00022 // .iso.org.dod.internet.mgmt (.1.3.6.1.2) 00023 // .iso.org.dod.internet.mgmt.mib-2 (.1.3.6.1.2.1) 00024 // .iso.org.dod.internet.mgmt.mib-2.system (.1.3.6.1.2.1.1) 00025 // .iso.org.dod.internet.mgmt.mib-2.system.sysDescr (.1.3.6.1.2.1.1.1) 00026 const char sysDescr[] = "1.3.6.1.2.1.1.1.0"; // read-only (DisplayString) 00027 // .iso.org.dod.internet.mgmt.mib-2.system.sysObjectID (.1.3.6.1.2.1.1.2) 00028 const char sysObjectID[] = "1.3.6.1.2.1.1.2.0"; // read-only (ObjectIdentifier) 00029 // .iso.org.dod.internet.mgmt.mib-2.system.sysUpTime (.1.3.6.1.2.1.1.3) 00030 const char sysUpTime[] = "1.3.6.1.2.1.1.3.0"; // read-only (TimeTicks) 00031 // .iso.org.dod.internet.mgmt.mib-2.system.sysContact (.1.3.6.1.2.1.1.4) 00032 const char sysContact[] = "1.3.6.1.2.1.1.4.0"; // read-write (DisplayString) 00033 // .iso.org.dod.internet.mgmt.mib-2.system.sysName (.1.3.6.1.2.1.1.5) 00034 const char sysName[] = "1.3.6.1.2.1.1.5.0"; // read-write (DisplayString) 00035 // .iso.org.dod.internet.mgmt.mib-2.system.sysLocation (.1.3.6.1.2.1.1.6) 00036 const char sysLocation[] = "1.3.6.1.2.1.1.6.0"; // read-write (DisplayString) 00037 // .iso.org.dod.internet.mgmt.mib-2.system.sysServices (.1.3.6.1.2.1.1.7) 00038 const char sysServices[] = "1.3.6.1.2.1.1.7.0"; // read-only (Integer) 00039 // 00040 // Arduino defined OIDs 00041 // .iso.org.dod.internet.private (.1.3.6.1.4) 00042 // .iso.org.dod.internet.private.enterprises (.1.3.6.1.4.1) 00043 // .iso.org.dod.internet.private.enterprises.arduino (.1.3.6.1.4.1.36582) 00044 // 00045 // 00046 // RFC1213 local values 00047 static char locDescr[] = "Agentbed, a light-weight SNMP Agent."; // read-only (static) 00048 static char locObjectID[] = "1.3.6.1.4.1.36582"; // read-only (static) 00049 static uint32_t locUpTime = 0; // read-only (static) 00050 static char locContact[] = "<root@weather>"; // should be stored/read from EEPROM - read/write (not done for simplicity) 00051 static char locName[] = "weather.mbed"; // should be stored/read from EEPROM - read/write (not done for simplicity) 00052 static char locLocation[] = "weather"; // should be stored/read from EEPROM - read/write (not done for simplicity) 00053 static int32_t locServices = 7; // read-only (static) 00054 00055 uint32_t prevMillis = 0; 00056 char oid[SNMP_MAX_OID_LEN]; 00057 SNMP_API_STAT_CODES api_status; 00058 SNMP_ERR_CODES status; 00059 00060 00061 void pduReceived() 00062 { 00063 SNMP_PDU pdu; 00064 // 00065 api_status = Agentbed.requestPdu(&pdu); 00066 // 00067 if ( pdu.type == SNMP_PDU_GET || pdu.type == SNMP_PDU_GET_NEXT || pdu.type == SNMP_PDU_SET 00068 && pdu.error == SNMP_ERR_NO_ERROR && api_status == SNMP_API_STAT_SUCCESS ) { 00069 // 00070 pdu.OID.toString(oid); 00071 // 00072 pdu.error = SNMP_ERR_READ_ONLY; 00073 // 00074 if ( strcmp(oid, sysDescr ) == 0 ) { 00075 // handle sysDescr (set/get) requests 00076 if ( pdu.type == SNMP_PDU_GET ) { 00077 // response packet from get-request - locDescr 00078 status = pdu.VALUE.encode(SNMP_SYNTAX_OCTETS, locDescr); 00079 pdu.error = status; 00080 } 00081 } else if ( strcmp(oid, sysObjectID ) == 0 ) { 00082 // handle sysName (set/get) requests 00083 if ( pdu.type == SNMP_PDU_GET ) { 00084 // response packet from get-request - locUpTime 00085 status = pdu.VALUE.encode(SNMP_SYNTAX_OCTETS, locObjectID); 00086 pdu.error = status; 00087 } 00088 } else if ( strcmp(oid, sysUpTime ) == 0 ) { 00089 // handle sysName (set/get) requests 00090 if ( pdu.type == SNMP_PDU_GET ) { 00091 // response packet from get-request - locUpTime 00092 status = pdu.VALUE.encode(SNMP_SYNTAX_TIME_TICKS, locUpTime); 00093 pdu.error = status; 00094 } 00095 } else if ( strcmp(oid, sysName ) == 0 ) { 00096 // handle sysName (set/get) requests 00097 if ( pdu.type == SNMP_PDU_GET ) { 00098 // response packet from get-request - locName 00099 status = pdu.VALUE.encode(SNMP_SYNTAX_OCTETS, locName); 00100 pdu.error = status; 00101 } 00102 } else if ( strcmp(oid, sysContact ) == 0 ) { 00103 // handle sysContact (set/get) requests 00104 if ( pdu.type == SNMP_PDU_GET ) { 00105 // response packet from get-request - locContact 00106 status = pdu.VALUE.encode(SNMP_SYNTAX_OCTETS, locContact); 00107 pdu.error = status; 00108 } 00109 } else if ( strcmp(oid, sysLocation ) == 0 ) { 00110 // handle sysLocation (set/get) requests 00111 if ( pdu.type == SNMP_PDU_GET ) { 00112 // response packet from get-request - locLocation 00113 status = pdu.VALUE.encode(SNMP_SYNTAX_OCTETS, locLocation); 00114 pdu.error = status; 00115 } 00116 } else if ( strcmp(oid, sysServices) == 0 ) { 00117 // handle sysServices (set/get) requests 00118 if ( pdu.type == SNMP_PDU_GET ) { 00119 // response packet from get-request - locServices 00120 status = pdu.VALUE.encode(SNMP_SYNTAX_INT, locServices); 00121 pdu.error = status; 00122 } 00123 } else { 00124 // oid does not exist 00125 // 00126 // response packet - object not found 00127 pdu.error = SNMP_ERR_NO_SUCH_NAME; 00128 } 00129 // 00130 pdu.type = SNMP_PDU_RESPONSE; 00131 00132 Agentbed.responsePdu(&pdu); 00133 } 00134 // 00135 Agentbed.freePdu(&pdu); 00136 // 00137 //Serial << "UDP Packet Received End.." << " RAM:" << freeMemory() << endl; 00138 } 00139 00140 00141 00142 00143 int main () 00144 { 00145 EthernetErr r; 00146 00147 r = eth.setup(); 00148 if (r) { 00149 printf("Error %d in setup.\n", r); 00150 return -1; 00151 } 00152 00153 api_status = Agentbed.begin(ð); 00154 if (api_status != SNMP_API_STAT_SUCCESS) { 00155 return -1; 00156 } 00157 00158 Agentbed.onPduReceive(pduReceived); 00159 printf("Agentbed\r\n"); 00160 00161 while (1) { 00162 wait(1); 00163 locUpTime += 100; 00164 Net::poll(); 00165 } 00166 }
Generated on Wed Jul 13 2022 19:03:32 by
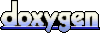