NEC Near Field Communication RF module library for mbed H001-000003-001 (950MHz), H001-000013-001 (920MHz), TY24FM-E2024 (2.4GHz)
Dependents: NECnfc_sample Drone_air Drone_ground
NECnfc_util.cpp
00001 #include "NECnfc.h" 00002 00003 int NECnfc::setRfConfig (enum NECPWR power, int ch, NECBAUD baud) { 00004 char cmd[3]; 00005 00006 switch (_type) { 00007 case TYPE_2400MHz: 00008 if (ch < 0 || ch > 15) return -1; 00009 power = (enum NECPWR)(power >= PWR_HIGH ? 0x0f : 0x00); 00010 case TYPE_950MHz: 00011 if (power == PWR_LOW && (ch < 1 || ch > 33)) return -1; 00012 if (power >= PWR_MID && (ch < 17 || ch > 31)) return -1; 00013 break; 00014 case TYPE_920MHz: 00015 if (power <= PWR_MID && (ch < 24 || ch > 38)) return -1; 00016 if (power >= PWR_HIGH && (ch < 24 || ch > 37 || ch == 32)) return -1; 00017 break; 00018 } 00019 00020 if (_type == TYPE_2400MHz) { 00021 cmd[0] = ch; 00022 if (send(NECMSG_WRITE_CHANNEL, NEC_DUMMYID, cmd, 1)) { 00023 return -1; 00024 } 00025 cmd[0] = power; 00026 if (send(NECMSG_WRITE_RFCONF, NEC_DUMMYID, cmd, 1)) { 00027 return -1; 00028 } 00029 } else { 00030 cmd[0] = power; 00031 cmd[1] = ch; 00032 cmd[2] = baud; 00033 if (send(NECMSG_WRITE_RFCONF, NEC_DUMMYID, cmd, 3)) { 00034 return -1; 00035 } 00036 } 00037 return 0; 00038 } 00039 00040 unsigned int NECnfc::getId () { 00041 00042 if (send(NECMSG_READ_DEFAULT, NEC_DUMMYID, NULL, 0)) { 00043 return 0; 00044 } 00045 if (_type == TYPE_2400MHz) { 00046 _id = (_rxmsg.parameter[23] << 24) | (_rxmsg.parameter[24] << 16) | (_rxmsg.parameter[25] << 8) | _rxmsg.parameter[26]; 00047 } else { 00048 _id = (_rxmsg.parameter[21] << 24) | (_rxmsg.parameter[22] << 16) | (_rxmsg.parameter[23] << 8) | _rxmsg.parameter[24]; 00049 } 00050 return _id; 00051 } 00052 00053 unsigned int NECnfc::getSystemId () { 00054 unsigned int id; 00055 00056 if (send(NECMSG_READ_DEFAULT, NEC_DUMMYID, NULL, 0)) { 00057 return 0; 00058 } 00059 00060 if (_type == TYPE_2400MHz) { 00061 id = (_rxmsg.parameter[19] << 24) | (_rxmsg.parameter[20] << 16) | (_rxmsg.parameter[21] << 8) | _rxmsg.parameter[22]; 00062 } else { 00063 id = (_rxmsg.parameter[17] << 24) | (_rxmsg.parameter[18] << 16) | (_rxmsg.parameter[19] << 8) | _rxmsg.parameter[20]; 00064 } 00065 return id; 00066 } 00067 00068 int NECnfc::setSystemId (unsigned int id) { 00069 int len; 00070 char buf[24]; 00071 00072 if (send(NECMSG_READ_DEFAULT, NEC_DUMMYID, NULL, 0)) { 00073 return 0; 00074 } 00075 00076 if (_type == TYPE_2400MHz) { 00077 len = 23; 00078 memcpy(buf, _rxmsg.parameter, len); 00079 buf[19] = (id >> 24) & 0xff; 00080 buf[20] = (id >> 16) & 0xff; 00081 buf[21] = (id >> 8) & 0xff; 00082 buf[22] = id & 0xff; 00083 } else { 00084 len = 21; 00085 memcpy(buf, _rxmsg.parameter, len); 00086 buf[17] = (id >> 24) & 0xff; 00087 buf[18] = (id >> 16) & 0xff; 00088 buf[19] = (id >> 8) & 0xff; 00089 buf[20] = id & 0xff; 00090 } 00091 if (send(NECMSG_WRITE_DEFAULT, NEC_DUMMYID, buf, len)) { 00092 return -1; 00093 } 00094 return 0; 00095 } 00096 00097 int NECnfc::setSleepMode (int sleep_time, int rev_time) { 00098 int len; 00099 char buf[24]; 00100 00101 if (send(NECMSG_READ_CONFIG, NEC_DUMMYID, NULL, 0)) { 00102 return 0; 00103 } 00104 00105 if (_type == TYPE_2400MHz) { 00106 len = 22; 00107 memcpy(buf, _rxmsg.parameter, len); 00108 buf[12] = (rev_time >> 8) & 0xff; 00109 buf[13] = rev_time & 0xff; 00110 buf[14] = sleep_time; 00111 } else { 00112 len = 21; 00113 memcpy(buf, _rxmsg.parameter, len); 00114 buf[6] = sleep_time; 00115 buf[7] = (rev_time >> 8) & 0xff; 00116 buf[8] = rev_time & 0xff; 00117 } 00118 if (send(NECMSG_WRITE_CONFIG, NEC_DUMMYID, buf, len)) { 00119 return -1; 00120 } 00121 return 0; 00122 } 00123 00124 int NECnfc::getRssi () { 00125 00126 if (send(NECMSG_READ_RSSI, NEC_DUMMYID, NULL, 0)) { 00127 return 0; 00128 } 00129 return _rssi; 00130 } 00131 00132 int NECnfc::search () { 00133 char cmd[1] = {1}; 00134 Timer t; 00135 00136 DBG("search\r\n"); 00137 t.start(); 00138 if (send(NECMSG_SEARCH, NEC_DUMMYID, cmd, 1)) { 00139 return -1; 00140 } 00141 for (;;) { 00142 if (_ack && _rxmsg.msgid == NECMSG_ACK) { 00143 DBG("found %08x", ntohl(_rxmsg.srcid)); 00144 _ack = 0; 00145 } 00146 if (_resend) break; 00147 if (t.read() > NEC_TIMEOUT) { 00148 DBG("timeout\r\n"); 00149 t.stop(); 00150 return -1; 00151 } 00152 } 00153 t.stop(); 00154 DBG(" ack %d, noack %d, resend %d\r\n", _ack, _noack, _resend); 00155 return 0; 00156 }
Generated on Wed Jul 13 2022 14:59:48 by
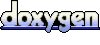