SMPTE timedode (LTC) decode library for mbed
Embed:
(wiki syntax)
Show/hide line numbers
LTC_SMPTE.h
Go to the documentation of this file.
00001 /** 00002 * SMPTE timedode (LTC) decode library for mbed 00003 * Copyright (c) 2015 Suga 00004 * Released under the MIT License: http://mbed.org/license/mit 00005 */ 00006 /** @file 00007 * @brief SMPTE timedode (LTC) decode library for mbed 00008 */ 00009 00010 #ifndef _LTC_SMPTE_H_ 00011 #define _LTC_SMPTE_H_ 00012 00013 #include "mbed.h" 00014 00015 class LTC_SMPTE { 00016 public: 00017 enum LTC_TYPE { 00018 LTC_INPUT, 00019 LTC_OUTPUT 00020 }; 00021 00022 LTC_SMPTE (PinName pin, enum LTC_TYPE type = LTC_INPUT); 00023 00024 void read (int *hour, int *min, int *sec, int *frame, int *dir = NULL); 00025 int isReceived (); 00026 00027 void write (int hour, int min, int sec, int frame, int dir = 0); 00028 00029 static LTC_SMPTE *_inst; 00030 void isr_ticker (); 00031 00032 protected: 00033 InterruptIn *_input; 00034 Timer _timer; 00035 DigitalOut *_output; 00036 00037 int mode; 00038 int oneflg; 00039 int count; 00040 int bit; 00041 char code[10]; 00042 int hour, min, sec, frame; 00043 int drop, direction, fps; 00044 int received; 00045 00046 enum LTC_TYPE type; 00047 int phase, zeroflg; 00048 00049 void isr_change (); 00050 void parse_code (); 00051 00052 }; 00053 00054 #endif
Generated on Wed Jul 27 2022 05:49:11 by
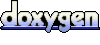