
http://mbed.org/users/okini3939/notebook/gainspan-wifi
Embed:
(wiki syntax)
Show/hide line numbers
main.cpp
00001 #include "mbed.h" 00002 #include "GSwifi.h" 00003 00004 //#define USE_SLEEP 00005 #define USE_STANDBY 00006 00007 #if defined(TARGET_LPC11U24) 00008 Serial pc(USBTX, USBRX); 00009 #endif 00010 DigitalOut led1(LED1), led2(LED2), led3(LED3), led4(LED4); 00011 //InterruptIn sw1(p23), sw2(p24), sw3(p25), sw4(p26); 00012 DigitalIn sw1(p23), sw2(p24), sw3(p25), sw4(p26); 00013 00014 #if defined(TARGET_LPC1768) || defined(TARGET_LPC2368) 00015 GSwifi gs(p13, p14, p12, P0_22); // TX, RX, CTS, RTS 00016 DigitalOut gs_reset(p9), gs_wakeup(p10); 00017 #elif defined(TARGET_LPC11U24) 00018 GSwifi gs(p9, p10, p21, p22); // TX, RX, CTS, RTS 00019 DigitalOut gs_reset(p27), gs_wakeup(p28); 00020 #endif 00021 00022 Host server; 00023 volatile int gsconnect = 0, button = 0; 00024 00025 void isr_sw1 () { 00026 if (gsconnect == 0) gsconnect = 1; 00027 } 00028 00029 void isr_sw2 () { 00030 button = 1; 00031 } 00032 00033 void isr_sw3 () { 00034 button = 2; 00035 } 00036 00037 void isr_sw4 () { 00038 button = 3; 00039 } 00040 00041 void isr_udp (int cid, int acid, int len) { 00042 int i; 00043 char buf[20]; 00044 Host host; 00045 00046 i = gs.recv(cid, buf, sizeof(buf), host); 00047 buf[i] = 0; 00048 if (i > 0 && strncmp("Welcome", buf, 7) == 0) { 00049 server.setIp(host.getIp()); 00050 } 00051 } 00052 00053 void find () { 00054 int i; 00055 int cid; 00056 Host bloadcast; 00057 00058 bloadcast.setPort(10123); 00059 bloadcast.setIp(IpAddr(255,255,255,255)); 00060 00061 cid = gs.open(bloadcast, GSPROT_UDP, &isr_udp); 00062 if (cid >= 0) { 00063 gs.send(cid, "Hello", 5); 00064 for (i = 0; i < 15; i ++) { 00065 gs.poll(); 00066 wait_ms(100); 00067 } 00068 gs.close(cid); 00069 } 00070 } 00071 00072 void key () { 00073 if (sw1 == 0 && gsconnect == 0) gsconnect = 1; 00074 if (sw2 == 0 && button == 0) button = 1; 00075 if (sw3 == 0 && button == 0) button = 2; 00076 if (sw4 == 0 && button == 0) button = 3; 00077 } 00078 00079 int main() { 00080 int i; 00081 00082 #if defined(TARGET_LPC11U24) 00083 pc.baud(115200); 00084 #endif 00085 gs_reset = 0; 00086 gs_wakeup = 1; 00087 sw1.mode(PullUp); 00088 sw2.mode(PullUp); 00089 sw3.mode(PullUp); 00090 sw4.mode(PullUp); 00091 wait_ms(100); 00092 /* 00093 sw1.fall(&isr_sw1); 00094 sw2.fall(&isr_sw2); 00095 sw3.fall(&isr_sw3); 00096 sw4.fall(&isr_sw4); 00097 */ 00098 server.setPort(10123); 00099 server.setIp(IpAddr(192,168,111,20)); 00100 gs_reset = 1; 00101 wait_ms(500); 00102 led1 = 1; 00103 00104 for (;;) { 00105 key(); 00106 gs.poll(); 00107 /* 00108 switch (gs.getStatus()) { 00109 case GSSTAT_READY: 00110 sleep(); 00111 break; 00112 case GSSTAT_WAKEUP: 00113 gs.standby(600000); 00114 break; 00115 } 00116 */ 00117 if (gs.getStatus() == GSSTAT_WAKEUP) { 00118 gs.standby(600000); 00119 } 00120 00121 if (gsconnect == 1) { 00122 // WPS 00123 led1 = 0; 00124 led3 = 1; 00125 if (gs.connect(GSSEC_WPS_BUTTON, NULL, NULL, 1)) { 00126 // if (gs.connect(GSSEC_WPA2_PSK, "GSTEST", "testpass12345", 1)) { 00127 led1 = 1; 00128 led2 = 0; 00129 gsconnect = 0; 00130 } else { 00131 led1 = 0; 00132 led2 = 1; 00133 gsconnect = 2; 00134 00135 find(); 00136 #ifdef USE_SLEEP 00137 gs.deepSleep(); 00138 #endif 00139 #ifdef USE_STANDBY 00140 gs.standby(600000); 00141 #endif 00142 } 00143 led3 = 0; 00144 } 00145 00146 if (button) { 00147 led4 = 1; 00148 if (gsconnect == 2) { 00149 int cid; 00150 char buf[10]; 00151 00152 #ifdef USE_SLEEP 00153 gs.wakeup(); 00154 #endif 00155 #ifdef USE_STANDBY 00156 gs_wakeup = 0; 00157 while (gs.getStatus() != GSSTAT_WAKEUP) { 00158 gs.poll(); 00159 } 00160 gs_wakeup = 1; 00161 gs.wakeup(); 00162 #endif 00163 led3 = 1; 00164 cid = gs.open(server, GSPROT_UDP); 00165 if (cid >= 0) { 00166 buf[0] = '0' + button; 00167 gs.send(cid, buf, 1); 00168 gs.close(cid); 00169 } 00170 led3 = 0; 00171 #ifdef USE_SLEEP 00172 gs.deepSleep(); 00173 #endif 00174 #ifdef USE_STANDBY 00175 gs.standby(600000); 00176 #endif 00177 } 00178 button = 0; 00179 led4 = 0; 00180 } 00181 00182 } 00183 }
Generated on Tue Jul 12 2022 21:46:35 by
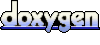