
dynamic load and run users binary file. self write the flash memory.
Embed:
(wiki syntax)
Show/hide line numbers
handler.cpp
00001 #include "mbed.h" 00002 00003 void jump (int vect); 00004 00005 extern "C" { 00006 00007 void SysTick_Handler() { 00008 jump(15); 00009 } 00010 00011 void WDT_IRQHandler () { 00012 jump(16); 00013 } 00014 00015 void TIMER0_IRQHandler() { 00016 jump(17); 00017 } 00018 00019 void TIMER1_IRQHandler() { 00020 jump(18); 00021 } 00022 00023 void TIMER2_IRQHandler() { 00024 jump(19); 00025 } 00026 00027 void TIMER3_IRQHandler() { 00028 jump(20); 00029 } 00030 00031 void UART0_IRQHandler() { 00032 jump(21); 00033 } 00034 00035 void UART1_IRQHandler() { 00036 jump(22); 00037 } 00038 00039 void UART2_IRQHandler() { 00040 jump(23); 00041 } 00042 00043 void UART3_IRQHandler() { 00044 jump(24); 00045 } 00046 00047 void PWM1_IRQHandler() { 00048 jump(25); 00049 } 00050 00051 void I2C0_IRQHandler() { 00052 jump(26); 00053 } 00054 00055 void I2C1_IRQHandler() { 00056 jump(27); 00057 } 00058 00059 void I2C2_IRQHandler() { 00060 jump(28); 00061 } 00062 00063 void SPI_IRQHandler() { 00064 jump(29); 00065 } 00066 00067 void SSP0_IRQHandler() { 00068 jump(30); 00069 } 00070 00071 void SSP1_IRQHandler() { 00072 jump(31); 00073 } 00074 00075 void PLL0_IRQHandler() { 00076 jump(32); 00077 } 00078 00079 void RTC_IRQHandler() { 00080 jump(33); 00081 } 00082 00083 void EINT0_IRQHandler() { 00084 jump(34); 00085 } 00086 00087 void EINT1_IRQHandler() { 00088 jump(35); 00089 } 00090 00091 void EINT2_IRQHandler() { 00092 jump(36); 00093 } 00094 00095 void EINT3_IRQHandler() { 00096 jump(37); 00097 } 00098 00099 void ADC_IRQHandler() { 00100 jump(38); 00101 } 00102 00103 void BOD_IRQHandler() { 00104 jump(39); 00105 } 00106 00107 void USB_IRQHandler() { 00108 jump(40); 00109 } 00110 00111 void CAN_IRQHandler() { 00112 jump(41); 00113 } 00114 00115 void DMA_IRQHandler() { 00116 jump(42); 00117 } 00118 00119 void I2S_IRQHandler() { 00120 jump(43); 00121 } 00122 00123 void ENET_IRQHandler() { 00124 jump(44); 00125 } 00126 00127 void RIT_IRQHandler() { 00128 jump(45); 00129 } 00130 00131 void MCPWM_IRQHandler() { 00132 jump(46); 00133 } 00134 00135 void QEI_IRQHandler() { 00136 jump(47); 00137 } 00138 00139 void PLL1_IRQHandler() { 00140 jump(48); 00141 } 00142 00143 } // extern "C"
Generated on Sat Jul 16 2022 13:37:35 by
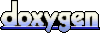