
DJI NAZA-M controller (remote controller side) see: https://developer.mbed.org/users/okini3939/notebook/drone/
Dependencies: NECnfc SpiOLED USBHost mbed
rf920.cpp
00001 #include "mbed.h" 00002 #include "drone.h" 00003 #include "NECnfc.h" 00004 00005 #define RF_CHANNEL 34 00006 #define RF_CHANNEL2 30 00007 00008 NECnfc rf(p13, p14, p11, p12, NC, 38400, NECnfc::TYPE_920MHz); // tx, rx, reset, wakeup, mode 00009 //cts 12, rts 13 00010 NECnfc rf2(p9, p10, NC, 38400, NECnfc::TYPE_920MHz); // tx, rx, reset, wakeup, mode 00011 00012 //static int air = NEC_DUMMYID; 00013 const static int air = 0x4b800063; 00014 static int seq = 1; 00015 00016 extern int rf_dual; 00017 00018 void isrRecv () { 00019 char buf[NEC_MAXLENGTH + 1]; 00020 int i, len, dest, src; 00021 uint8_t sum = 0; 00022 00023 len = rf.readData(&dest, &src, buf, sizeof(buf)); 00024 if (len <= 0) return; 00025 00026 for (i = 0; i < len; i ++) { 00027 sum += (uint8_t)buf[i]; 00028 } 00029 if (sum) return; // sum error 00030 if (strncmp(buf, "Suge", 4) != NULL) return; 00031 rf_dual &= ~2; 00032 00033 len --; 00034 buf[len] = 0; 00035 if (air != src) { 00036 // air = src; 00037 printf("new air %08x\r\n", air); 00038 } 00039 // recvRf((struct GroundData *)buf, rf.getRssi()); 00040 recvRf((struct GroundData *)buf, 0); 00041 } 00042 00043 void isrRecv2 () { 00044 char buf[NEC_MAXLENGTH + 1]; 00045 int i, len, dest, src; 00046 uint8_t sum = 0; 00047 00048 len = rf2.readData(&dest, &src, buf, sizeof(buf)); 00049 if (len <= 0) return; 00050 00051 if (strncmp(buf, "Suge", 4) != NULL) return; 00052 rf_dual |= 2; 00053 00054 for (i = 0; i < len; i ++) { 00055 sum += (uint8_t)buf[i]; 00056 } 00057 if (sum) return; // sum error 00058 00059 len --; 00060 buf[len] = 0; 00061 // recvRf((struct GroundData *)buf, rf2.getRssi()); 00062 recvRf((struct GroundData *)buf, 0); 00063 } 00064 00065 int sendRf (struct AirData *send_data) { 00066 int i; 00067 uint8_t sum = 0; 00068 char *buf = (char*)send_data; 00069 00070 memcpy(send_data->magic, "Suge", 4); 00071 send_data->type = DATA_TYPE_AIR; 00072 send_data->seq = seq; 00073 seq ++; 00074 if (seq >= 0x10000) seq = 1; 00075 send_data->flags = rf_dual; 00076 00077 for (i = 0; i < sizeof(struct AirData) - 1; i ++) { 00078 sum += (uint8_t)buf[i]; 00079 } 00080 send_data->sum = ~sum + 1; // two's complement 00081 00082 return rf.sendData(air, buf, sizeof(struct AirData)); 00083 } 00084 00085 void pollRf () { 00086 rf.poll(); 00087 rf2.poll(); 00088 } 00089 00090 int initRf () { 00091 if (rf.setRfConfig(NECnfc::PWR_MAX, RF_CHANNEL, NECnfc::BAUD_50k)) return -1; 00092 rf.attach(&isrRecv); 00093 printf("RF %08x\r\n", rf.getId()); 00094 00095 if (rf2.setRfConfig(NECnfc::PWR_MAX, RF_CHANNEL2, NECnfc::BAUD_50k)) {; 00096 printf("RF2 error\r\n"); 00097 } else { 00098 rf2.attach(&isrRecv2); 00099 printf("RF2 %08x\r\n", rf2.getId()); 00100 rf_dual |= 1; 00101 } 00102 return 0; 00103 }
Generated on Mon Jul 18 2022 16:23:01 by
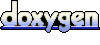