DALI send/recv library.
Embed:
(wiki syntax)
Show/hide line numbers
DALI.h
Go to the documentation of this file.
00001 /* 00002 * DALI send/recv library 00003 * Copyright (c) 2020 Hiroshi Suga 00004 * Released under the MIT License: http://mbed.org/license/mit 00005 */ 00006 00007 /** @file 00008 * @brief DALI send/recv 00009 */ 00010 00011 #ifndef _DALI_H_ 00012 #define _DALI_H_ 00013 00014 #include "CBuffer.h" 00015 00016 class DALI { 00017 public: 00018 enum DALI_FRAME { 00019 FORWARD_SHORT_DAP, // DIRECT ARC POWER 00020 FORWARD_SHORT_IAP, // COMMAND 00021 FORWARD_GROUP_DAP, 00022 FORWARD_GROUP_IAP, 00023 BACKWARD, 00024 }; 00025 00026 enum DALI_COMMAND { 00027 OFF = 0x00, 00028 UP = 0x01, 00029 DOWN = 0x02, 00030 STEP_UP = 0x03, 00031 STEP_DOWN = 0x04, 00032 RECALL_MAX_LEVEL = 0x05, 00033 RECALL_MIN_LEVEL = 0x06, 00034 STEP_DOWN_AND_OFF = 0x07, 00035 ON_AND_STEP_UP = 0x08, 00036 RESET = 0x20, 00037 QUERY_STATUS = 0x90, 00038 }; 00039 00040 /** init DALI class 00041 * @param tx TX port 00042 * @param rx RX port (interrupt) 00043 */ 00044 DALI (PinName tx, PinName rx); 00045 00046 /** Recv the data 00047 * @param frame enum DALI_FRAME 00048 * @param addr DALI address (short:0-63, group:0-15,63 broadcast) 00049 * @param value DALI value 00050 */ 00051 int read (enum DALI_FRAME *frame, int *addr, int *value); 00052 00053 int readable (); 00054 00055 /** Send the data 00056 * @param frame enum DALI_FRAME 00057 * @param addr DALI address (short:0-63, group:0-15,63 broadcast) 00058 * @param value DALI value 00059 */ 00060 int write (enum DALI_FRAME frame, int addr, int value); 00061 00062 int writable (); 00063 00064 private: 00065 InterruptIn _rx; 00066 DigitalOut _tx; 00067 Timeout _timer; 00068 Ticker _ticker; 00069 00070 CircBuffer<int> *recv_buf; 00071 CircBuffer<int> *send_buf; 00072 00073 volatile int mode; 00074 int count; 00075 int timeflg; 00076 int recv_bit; 00077 int recv_data; 00078 00079 int send_data; 00080 int send_bit; 00081 int halfbit; 00082 volatile int busy; 00083 00084 void isr_rx (); 00085 void isr_timer (); 00086 void isr_timeout (); 00087 void isr_send (); 00088 00089 }; 00090 00091 #endif
Generated on Wed Jul 20 2022 19:38:24 by
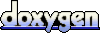