DALI send/recv library.
Embed:
(wiki syntax)
Show/hide line numbers
CBuffer.h
00001 /* Copyright (C) 2012 mbed.org, MIT License 00002 * 00003 * Permission is hereby granted, free of charge, to any person obtaining a copy of this software 00004 * and associated documentation files (the "Software"), to deal in the Software without restriction, 00005 * including without limitation the rights to use, copy, modify, merge, publish, distribute, 00006 * sublicense, and/or sell copies of the Software, and to permit persons to whom the Software is 00007 * furnished to do so, subject to the following conditions: 00008 * 00009 * The above copyright notice and this permission notice shall be included in all copies or 00010 * substantial portions of the Software. 00011 * 00012 * THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR IMPLIED, INCLUDING 00013 * BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND 00014 * NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, 00015 * DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, 00016 * OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE. 00017 */ 00018 00019 #ifndef CIRCBUFFER_H_ 00020 #define CIRCBUFFER_H_ 00021 00022 template <class T> 00023 class CircBuffer { 00024 public: 00025 CircBuffer(int length, void *addr = NULL) { 00026 write = 0; 00027 read = 0; 00028 size = length + 1; 00029 if (addr) { 00030 buf = (T *)addr; 00031 } else { 00032 buf = (T *)malloc(size * sizeof(T)); 00033 } 00034 if (buf == NULL) 00035 error("Can't allocate memory"); 00036 }; 00037 00038 bool isFull() { 00039 return (((write + 1) % size) == read); 00040 }; 00041 00042 bool isEmpty() { 00043 return (read == write); 00044 }; 00045 00046 bool queue(T k) { 00047 if (isFull()) { 00048 return false; 00049 } 00050 buf[write++] = k; 00051 write %= size; 00052 return true; 00053 } 00054 int queue(T *k, int size) { 00055 int i; 00056 for (i = 0; i < size; i ++) { 00057 if (queue(k[i]) == false) break; 00058 } 00059 return i; 00060 } 00061 00062 void flush() { 00063 read = 0; 00064 write = 0; 00065 } 00066 00067 00068 uint32_t available() { 00069 return (write >= read) ? write - read : size - read + write; 00070 }; 00071 uint32_t free() { 00072 return size - available() - 1; 00073 }; 00074 00075 bool dequeue(T * c) { 00076 bool empty = isEmpty(); 00077 if (!empty) { 00078 *c = buf[read++]; 00079 read %= size; 00080 } 00081 return(!empty); 00082 }; 00083 int dequeue(T * c, int size) { 00084 int i; 00085 for (i = 0; i < size; i ++) { 00086 if (dequeue(&c[i]) == false) break; 00087 } 00088 return i; 00089 } 00090 00091 private: 00092 volatile uint32_t write; 00093 volatile uint32_t read; 00094 uint32_t size; 00095 T * buf; 00096 }; 00097 00098 #endif
Generated on Wed Jul 20 2022 19:38:24 by
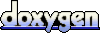