STMicroelectronics LPS331AP, LPS25H SPI Library. This library is base on https://developer.mbed.org/users/nyamfg/code/LPS331_I2C/
Dependents: LPS331_SPI_Test main_SPC
Fork of LPS331_I2C by
LPS331_SPI.h
00001 /* 00002 * I2C/SPI digital pressure sensor "LPS331AP" "LPS25H" library for SPI mode. 00003 * 00004 * http://www.st.com/web/en/resource/technical/document/datasheet/DM00036196.pdf 00005 * 00006 * Copyright(c) -2016 Toru OHTSUKA, 00007 * Copyright(c) -2013 unos@NYAMFG, 00008 * Released under the MIT License: http://mbed.org/license/mit 00009 * 00010 * revision 1.2 07-Jul-2016 SPI version. 00011 * revision 1.1 22-Oct-2013 Add multibyte read, Change temperature and pressure reading method. 00012 * revision 1.0 20-Oct-2013 1st release, Does not support interrupts. 00013 */ 00014 00015 #ifndef LPS331_SPI_H 00016 #define LPS331_SPI_H 00017 00018 #include "mbed.h" 00019 00020 // SA0 status configuration values. 00021 #define LPS331_SPI_SA0_HIGH true 00022 #define LPS331_SPI_SA0_LOW false 00023 00024 // Pressure configuration values. 00025 #define LPS331_SPI_PRESSURE_AVG_1 0x00 00026 #define LPS331_SPI_PRESSURE_AVG_2 0x01 00027 #define LPS331_SPI_PRESSURE_AVG_4 0x02 00028 #define LPS331_SPI_PRESSURE_AVG_8 0x03 00029 #define LPS331_SPI_PRESSURE_AVG_16 0x04 00030 #define LPS331_SPI_PRESSURE_AVG_32 0x05 00031 #define LPS331_SPI_PRESSURE_AVG_64 0x06 00032 #define LPS331_SPI_PRESSURE_AVG_128 0x07 00033 #define LPS331_SPI_PRESSURE_AVG_256 0x08 00034 #define LPS331_SPI_PRESSURE_AVG_384 0x09 00035 #define LPS331_SPI_PRESSURE_AVG_512 0x0a 00036 00037 // Temperature configuration values. 00038 #define LPS331_SPI_TEMP_AVG_1 0x00 00039 #define LPS331_SPI_TEMP_AVG_2 0x01 00040 #define LPS331_SPI_TEMP_AVG_4 0x02 00041 #define LPS331_SPI_TEMP_AVG_8 0x03 00042 #define LPS331_SPI_TEMP_AVG_16 0x04 00043 #define LPS331_SPI_TEMP_AVG_32 0x05 00044 #define LPS331_SPI_TEMP_AVG_64 0x06 00045 #define LPS331_SPI_TEMP_AVG_128 0x07 00046 00047 // Data Rate Pressure / Temperature 00048 #define LPS331_SPI_DATARATE_ONESHOT 0x00 // OneShot OneShot 00049 #define LPS331_SPI_DATARATE_1HZ 0x01 // 1Hz 1Hz 00050 #define LPS331_SPI_DATARATE_7HZ 0x02 // 7Hz 1Hz 00051 #define LPS331_SPI_DATARATE_12_5HZ 0x03 // 12.5Hz 1Hz 00052 #define LPS331_SPI_DATARATE_25HZ 0x04 // 25Hz 1Hz 00053 #define LPS331_SPI_DATARATE_7HZ_T 0x05 // 7Hz 7Hz 00054 #define LPS331_SPI_DATARATE_12_5HZ_T 0x06 // 12.5Hz 12.5Hz 00055 #define LPS331_SPI_DATARATE_25HZ_T 0x07 // 25Hz 25Hz (*) 00056 // (*) Not allowed with PRESSURE_AVG_512 & TEMP_AVG_128. 00057 // More information , see datasheet. 00058 00059 // I2C Address. 00060 #define LPS331_SPI_ADDRESS_SA0_HIGH 0xba 00061 #define LPS331_SPI_ADDRESS_SA0_LOW 0xb8 00062 00063 class LPS331_SPI 00064 { 00065 public: 00066 LPS331_SPI(PinName mosi, PinName miso, PinName sclk, PinName cs); 00067 ~LPS331_SPI(); 00068 00069 char whoami(); 00070 bool isLPS331(); 00071 bool isLPS25H(); 00072 00073 void setResolution(char pressure_avg, char temp_avg); 00074 void setActive(bool is_active); 00075 void setDataRate(char datarate); 00076 00077 void quickStart(); 00078 00079 float getPressure(); 00080 float getTemperature(); 00081 00082 void _write(char subaddress, char data); 00083 char _read(char subaddress); 00084 void _read_multibyte(char startsubaddress, char* data, char count); 00085 00086 private: 00087 SPI _spi; 00088 DigitalOut _cs; 00089 char _address; 00090 char _ctrlreg1; 00091 }; 00092 00093 00094 #endif /* LPC331_SPI_H */ 00095
Generated on Fri Jul 22 2022 21:26:34 by
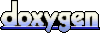