This library contains all the functions necesary to perform an application using the SRF02 sensor. Esta librería contiene los métodos necesarios para poder configurar todos los parámetros del sensor SRF02.
SRF02.cpp
00001 #include "SRF02.h" 00002 00003 00004 SRF02::SRF02(PinName sda, PinName scl, int addr) : m_i2c(sda, scl), m_addr(addr) { 00005 } 00006 00007 SRF02::~SRF02() { 00008 00009 } 00010 00011 //Get the data in centimeters 00012 00013 int SRF02::readcm() { 00014 00015 char cmd[2]; 00016 char eco_high[1],eco_low[1]; //this is because the sensor, sends the data in tow bytes, and you have tu read from two different registers 0x02 and 0x03; 00017 00018 00019 // Get range data from SRF02 in centimeters 00020 cmd[0] = 0x00; 00021 cmd[1] = 0x51; 00022 m_i2c.write(m_addr, cmd, 2); 00023 00024 wait(0.07); 00025 00026 00027 cmd[0] = 0x02; 00028 m_i2c.write(m_addr, cmd, 1, 1); 00029 m_i2c.read(m_addr, eco_high, 1); 00030 00031 cmd[0] = 0x03; 00032 m_i2c.write(m_addr,cmd,1,1); 00033 m_i2c.read(m_addr,eco_low,1); 00034 00035 int range = (eco_high[0]<<8)|eco_low[0]; 00036 00037 return range; 00038 } 00039 00040 //Get the data in inches 00041 00042 int SRF02::readinch() { 00043 00044 char cmd[2]; 00045 char eco_high[1],eco_low[1]; //this is because the sensor, sends the data in tow bytes, and you have tu read from two different registers 0x02 and 0x03; 00046 00047 00048 // Get range data from SRF02 in inches 00049 cmd[0] = 0x00; 00050 cmd[1] = 0x50; 00051 m_i2c.write(m_addr, cmd, 2); 00052 00053 wait(0.07); 00054 00055 00056 cmd[0] = 0x02; 00057 m_i2c.write(m_addr, cmd, 1, 1); 00058 m_i2c.read(m_addr, eco_high, 1); 00059 00060 cmd[0] = 0x03; 00061 m_i2c.write(m_addr,cmd,1,1); 00062 m_i2c.read(m_addr,eco_low,1); 00063 00064 int range = (eco_high[0]<<8)|eco_low[0]; 00065 00066 return range; 00067 } 00068 00069 void SRF02::change_addr(char new_addr){ 00070 00071 //Change adress of the device. Remember to have only one sensor conected to execute this method. 00072 00073 char cmd[2]; 00074 00075 cmd[0]=0x00; 00076 cmd[1]=0xA0; 00077 m_i2c.write(m_addr,cmd,2); 00078 cmd[0]=0x00; 00079 cmd[1]=0xAA; 00080 m_i2c.write(m_addr,cmd,2); 00081 cmd[0]=0x00; 00082 cmd[1]=0xA5; 00083 m_i2c.write(m_addr,cmd,2); 00084 cmd[0]=0x00; 00085 cmd[1]=new_addr; 00086 m_i2c.write(m_addr,cmd,2); 00087 00088 } 00089
Generated on Wed Jul 27 2022 04:44:02 by
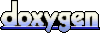