
TextLCD_clock using internal realtime clock function (to check VB functionality)
Embed:
(wiki syntax)
Show/hide line numbers
main.cpp
00001 // This code based on 00002 // http://mbed.org/users/okano/notebook/nxp_pcf2127a-demo-code/ 00003 // http://mbed.org/users/roen/notebook/real-time/ 00004 // 00005 // 2010-07-02 @nxpfan 00006 // Released under the MIT License: http://mbed.org/license/mit 00007 00008 #include "mbed.h" 00009 #include "TextLCD.h" 00010 00011 TextLCD lcd(p24, p25, p26, p27, p28, p29, p30); // rs, rw, e, d0, d1, d2, d3 00012 Ticker updater; 00013 00014 void lcd_update() 00015 { 00016 struct tm dt, *dtp; 00017 time_t t; 00018 char s[ 30 ]; 00019 dtp = &dt; 00020 00021 t = time( NULL ); 00022 dtp = localtime( &t ); 00023 00024 strftime( s, 20, "%H:%M:%S", dtp ); 00025 lcd.locate( 0, 0 ); 00026 lcd.printf( "%s", s ); 00027 00028 strftime( s, 20, "%Y/%b/%d(%a)", dtp ); 00029 lcd.locate( 0, 1 ); 00030 lcd.printf( "%s", s ); 00031 } 00032 00033 int main() { 00034 00035 // get the current time from the terminal 00036 struct tm t; 00037 00038 if ( 0 == time( NULL ) ) { // it should return ((time_t)-1) if it is not initialized but... 00039 lcd.locate( 0, 0 ); 00040 lcd.printf( "please set time from terminal" ); 00041 00042 printf("Enter current date and time:\n"); 00043 printf("YYYY MM DD HH MM SS[enter]\n"); 00044 scanf("%d %d %d %d %d %d", &t.tm_year, &t.tm_mon, &t.tm_mday 00045 , &t.tm_hour, &t.tm_min, &t.tm_sec); 00046 00047 // adjust for tm structure required values 00048 t.tm_year = t.tm_year - 1900; 00049 t.tm_mon = t.tm_mon - 1; 00050 00051 // set the time 00052 set_time(mktime(&t)); 00053 } 00054 00055 updater.attach(&lcd_update, 1.0); 00056 00057 while (1) 00058 ; 00059 }
Generated on Mon Jul 18 2022 10:20:53 by
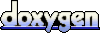