
mini board PCU9669 (and PCA9665) sample code
Dependencies: mbed PCU9669 utility PCA9665 I2C_slaves parallel_bus
Fork of mini_board_PCU9669_old by
main_PCU9669.c
00001 /** A sample code for "mini board PCU9669/PCA9665" 00002 * 00003 * @author Akifumi (Tedd) OKANO, NXP Semiconductors 00004 * @version 1.0 00005 * @date 26-Mar-2012 00006 * 00007 * Released under the MIT License: http://mbed.org/license/mit 00008 * 00009 * An operation sample of PCU9669/PCA9665 I2C bus controller. 00010 * The mbed accesses the bus controller's parallel port (8/2 bit address and 8 bit data) by bit-banging. 00011 * The bit-banging is poerformed by PortInOut function of mbed library. 00012 * 00013 * To make the code porting easier, all codes are partitioned into layers to abstract other parts. 00014 * The mbed specific parts are concentrated in lowest layer: "hardware_abs.*". 00015 * This module may need to be modified for the porting. 00016 * 00017 * All other upper layers are writen in standard-C. 00018 * 00019 * base code is written from 05-Sep-2011 to 09-Sep-2011. 00020 * And demo code has been build on 11-Sep-2011. 00021 * Debug and code adjustment has been done on 08-Sep-2011. 00022 * Small sanitization for main.cpp. All mbed related codes are moved in to "hardware_abs.*". 13-Oct-2011 00023 * hardware_abs are moved into parallel_bus library folder, 3 LED driver operation sample 13-Feb.-2012 00024 * PCU9669 and PCA9665 codes are packed in a project 14-Feb-2012. 00025 * 00026 * Before builidng the code, please edit the file mini_board_PCU9669/config.h 00027 * Un-comment the target name what you want to target. 00028 */ 00029 00030 /** @note Application layer module of PCU9669 00031 * 00032 * This code is made to explain basic PCU9669 operation. 00033 * And also, it demonstrates 3 channels of I2C operation with several slaves on those buses. 00034 * 00035 * The ch0 is an Fm+ bus that has a PCA9955 as slave device 00036 * The ch1 and ch2 are UFm buses. Each bus has a PCU9955 as slave devices. 00037 */ 00038 00039 #include "config.h" 00040 #include "hardware_abs.h" 00041 #include "transfer_manager.h" // abstracting the access of PCU9669 internal buffer 00042 #include "PCU9669_access.h" // PCU9669 chip access interface 00043 #include "PCx9955_reg.h" // slave specific definitions 00044 #include "PCA9629_reg.h" 00045 #include "utility.h" // 00046 //#include "mbed.h" // this header is required only when printf() is used. 00047 00048 #if defined( CODE_FOR_PCU9669 ) || defined( CODE_FOR_PCA9663 ) 00049 00050 #ifdef CODE_FOR_PCU9669 00051 #define TARGET_CHIP_ID PCU9669_ID 00052 #endif 00053 00054 #ifdef CODE_FOR_PCA9663 00055 #define TARGET_CHIP_ID PCA9663_ID 00056 #endif 00057 00058 #define RESET_PULSE_WIDTH_US 10 // Minimum pulse width is 4us for PCU9669 00059 #define RESET_RECOVERY_US 1000 00060 00061 /** 00062 * register settings for PCx9955 00063 */ 00064 00065 char PCx9955_reg_data[] = { 00066 0x80, // Strat register address with AutoIncrement bit 00067 0x00, 0x05, // MODE1, MODE2 00068 0xAA, 0xAA, 0xAA, 0xAA, // LEDOUT[3:0] 00069 0x80, 0x00, // GRPPWM, GRPFREQ 00070 PWM_INIT, PWM_INIT, PWM_INIT, PWM_INIT, // PWM[3:0] 00071 PWM_INIT, PWM_INIT, PWM_INIT, PWM_INIT, // PWM[7:4] 00072 PWM_INIT, PWM_INIT, PWM_INIT, PWM_INIT, // PWM[11:8] 00073 PWM_INIT, PWM_INIT, PWM_INIT, PWM_INIT, // PWM[15:12] 00074 IREF_INIT, IREF_INIT, IREF_INIT, IREF_INIT, // IREF[3:0] 00075 IREF_INIT, IREF_INIT, IREF_INIT, IREF_INIT, // IREF[7:4] 00076 IREF_INIT, IREF_INIT, IREF_INIT, IREF_INIT, // IREF[11:8] 00077 IREF_INIT, IREF_INIT, IREF_INIT, IREF_INIT, // IREF[15:12] 00078 0x08 // OFFSET: 1uS offsets 00079 }; 00080 00081 00082 char PCx9955_reg_read_start_address = 0x80; 00083 00084 char read_buffer[ 41 ]; 00085 00086 transaction ufm_transactions[] = { 00087 { PCx9955_ADDR0, PCx9955_reg_data, sizeof( PCx9955_reg_data ) } 00088 }; 00089 00090 transaction fm_plus_transactions[] = { 00091 { PCx9955_ADDR0, PCx9955_reg_data, sizeof( PCx9955_reg_data ) }, 00092 { PCx9955_ADDR0, &PCx9955_reg_read_start_address, 1 }, 00093 { PCx9955_ADDR0 | 0x01, read_buffer, sizeof( read_buffer ) } 00094 }; 00095 00096 char read_done = 0; 00097 00098 void interrupt_handler( void ); 00099 00100 int main() { 00101 char clear[ 16 ] = { 0 }; 00102 int count = 0; 00103 int i; 00104 int j; 00105 00106 // printf( "\r\nPCU9669 simple demo program on mbed\r\n build : %s (UTC), %s \r\n\r\n", __TIME__, __DATE__ ); 00107 00108 hardware_initialize(); // initializing bit-banging parallel port 00109 reset( RESET_PULSE_WIDTH_US, RESET_RECOVERY_US ); // assert hardware /RESET sgnal 00110 00111 if ( start_bus_controller( TARGET_CHIP_ID ) ) // wait the bus controller ready and check chip ID 00112 return 1; 00113 00114 write_ch_register( CH_FM_PLUS, INTMSK, 0x30 ); // set bus controller to ignore NAK return from Fm+ slaves 00115 install_ISR( &interrupt_handler ); // interrupt service routine install 00116 00117 setup_transfer( CH_FM_PLUS, fm_plus_transactions, sizeof( fm_plus_transactions ) / sizeof( transaction ) ); 00118 setup_transfer( CH_UFM1, ufm_transactions, sizeof( ufm_transactions ) / sizeof( transaction ) ); 00119 setup_transfer( CH_UFM2, ufm_transactions, sizeof( ufm_transactions ) / sizeof( transaction ) ); 00120 00121 while ( 1 ) { 00122 for ( i = 0; i < 16; i++ ) { 00123 for ( j = 0; j < 256; j += 4 ) { 00124 buffer_overwrite( CH_FM_PLUS, 0, 9 + i, (char *)&j, 1 ); 00125 buffer_overwrite( CH_UFM1, 0, 9 + i, (char *)&j, 1 ); 00126 buffer_overwrite( CH_UFM2, 0, 9 + i, (char *)&j, 1 ); 00127 00128 read_done = 0; 00129 00130 set_n_of_transaction( CH_FM_PLUS, (sizeof( fm_plus_transactions ) / sizeof( transaction )) - ((count % 256) ? 1 : 0) ); 00131 00132 start( CH_FM_PLUS ); 00133 start( CH_UFM1 ); 00134 start( CH_UFM2 ); 00135 00136 wait_sec( 0.01 ); 00137 count++; 00138 #if 0 00139 if ( read_done ) { 00140 buffer_read( CH_FM_PLUS, 2, 0, read_buffer, sizeof( read_buffer ) ); 00141 dump_read_data( read_buffer, sizeof( read_buffer ) ); 00142 read_done = 0; 00143 } 00144 #endif 00145 } 00146 } 00147 buffer_overwrite( CH_FM_PLUS, 0, 9, clear, 16 ); 00148 buffer_overwrite( CH_UFM1, 0, 9, clear, 16 ); 00149 buffer_overwrite( CH_UFM2, 0, 9, clear, 16 ); 00150 } 00151 } 00152 00153 void interrupt_handler( void ) { 00154 char global_status; 00155 char channel_status; 00156 00157 global_status = read_data( CTRLSTATUS ); 00158 00159 if ( global_status & 0x01 ) { // ch0 00160 channel_status = read_ch_register( 0, CHSTATUS ); 00161 00162 if ( channel_status & 0x80 ) 00163 read_done = 1; 00164 } 00165 if ( global_status & 0x02 ) { 00166 channel_status = read_ch_register( 1, CHSTATUS ); 00167 } 00168 if ( global_status & 0x04 ) { 00169 channel_status = read_ch_register( 2, CHSTATUS ); 00170 } 00171 // printf( "ISR channel_status 0x%02X\r\n", channel_status ); 00172 } 00173 00174 #endif // CODE_FOR_PCU9669 00175 00176 00177 00178 00179 00180
Generated on Sat Jul 16 2022 23:21:06 by
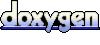