This program is porting rosserial_arduino for mbed http://www.ros.org/wiki/rosserial_arduino This program supported the revision of 169 of rosserial.
Dependents: rosserial_mbed robot_S2
Log.h
00001 #ifndef _ROS_rosgraph_msgs_Log_h 00002 #define _ROS_rosgraph_msgs_Log_h 00003 00004 #include <stdint.h> 00005 #include <string.h> 00006 #include <stdlib.h> 00007 #include "ros/msg.h" 00008 #include "std_msgs/Header.h" 00009 #include "rosgraph_msgs/byte.h" 00010 00011 namespace rosgraph_msgs 00012 { 00013 00014 class Log : public ros::Msg 00015 { 00016 public: 00017 std_msgs::Header header; 00018 rosgraph_msgs::byte level; 00019 char * name; 00020 char * msg; 00021 char * file; 00022 char * function; 00023 uint32_t line; 00024 uint8_t topics_length; 00025 char* st_topics; 00026 char* * topics; 00027 enum { DEBUG = 1 }; 00028 enum { INFO = 2 }; 00029 enum { WARN = 4 }; 00030 enum { ERROR = 8 }; 00031 enum { FATAL = 16 }; 00032 00033 virtual int serialize(unsigned char *outbuffer) const 00034 { 00035 int offset = 0; 00036 offset += this->header.serialize(outbuffer + offset); 00037 offset += this->level.serialize(outbuffer + offset); 00038 uint32_t * length_name = (uint32_t *)(outbuffer + offset); 00039 *length_name = strlen( (const char*) this->name); 00040 offset += 4; 00041 memcpy(outbuffer + offset, this->name, *length_name); 00042 offset += *length_name; 00043 uint32_t * length_msg = (uint32_t *)(outbuffer + offset); 00044 *length_msg = strlen( (const char*) this->msg); 00045 offset += 4; 00046 memcpy(outbuffer + offset, this->msg, *length_msg); 00047 offset += *length_msg; 00048 uint32_t * length_file = (uint32_t *)(outbuffer + offset); 00049 *length_file = strlen( (const char*) this->file); 00050 offset += 4; 00051 memcpy(outbuffer + offset, this->file, *length_file); 00052 offset += *length_file; 00053 uint32_t * length_function = (uint32_t *)(outbuffer + offset); 00054 *length_function = strlen( (const char*) this->function); 00055 offset += 4; 00056 memcpy(outbuffer + offset, this->function, *length_function); 00057 offset += *length_function; 00058 *(outbuffer + offset + 0) = (this->line >> (8 * 0)) & 0xFF; 00059 *(outbuffer + offset + 1) = (this->line >> (8 * 1)) & 0xFF; 00060 *(outbuffer + offset + 2) = (this->line >> (8 * 2)) & 0xFF; 00061 *(outbuffer + offset + 3) = (this->line >> (8 * 3)) & 0xFF; 00062 offset += sizeof(this->line); 00063 *(outbuffer + offset++) = topics_length; 00064 *(outbuffer + offset++) = 0; 00065 *(outbuffer + offset++) = 0; 00066 *(outbuffer + offset++) = 0; 00067 for( uint8_t i = 0; i < topics_length; i++){ 00068 uint32_t * length_topicsi = (uint32_t *)(outbuffer + offset); 00069 *length_topicsi = strlen( (const char*) this->topics[i]); 00070 offset += 4; 00071 memcpy(outbuffer + offset, this->topics[i], *length_topicsi); 00072 offset += *length_topicsi; 00073 } 00074 return offset; 00075 } 00076 00077 virtual int deserialize(unsigned char *inbuffer) 00078 { 00079 int offset = 0; 00080 offset += this->header.deserialize(inbuffer + offset); 00081 offset += this->level.deserialize(inbuffer + offset); 00082 uint32_t length_name = *(uint32_t *)(inbuffer + offset); 00083 offset += 4; 00084 for(unsigned int k= offset; k< offset+length_name; ++k){ 00085 inbuffer[k-1]=inbuffer[k]; 00086 } 00087 inbuffer[offset+length_name-1]=0; 00088 this->name = (char *)(inbuffer + offset-1); 00089 offset += length_name; 00090 uint32_t length_msg = *(uint32_t *)(inbuffer + offset); 00091 offset += 4; 00092 for(unsigned int k= offset; k< offset+length_msg; ++k){ 00093 inbuffer[k-1]=inbuffer[k]; 00094 } 00095 inbuffer[offset+length_msg-1]=0; 00096 this->msg = (char *)(inbuffer + offset-1); 00097 offset += length_msg; 00098 uint32_t length_file = *(uint32_t *)(inbuffer + offset); 00099 offset += 4; 00100 for(unsigned int k= offset; k< offset+length_file; ++k){ 00101 inbuffer[k-1]=inbuffer[k]; 00102 } 00103 inbuffer[offset+length_file-1]=0; 00104 this->file = (char *)(inbuffer + offset-1); 00105 offset += length_file; 00106 uint32_t length_function = *(uint32_t *)(inbuffer + offset); 00107 offset += 4; 00108 for(unsigned int k= offset; k< offset+length_function; ++k){ 00109 inbuffer[k-1]=inbuffer[k]; 00110 } 00111 inbuffer[offset+length_function-1]=0; 00112 this->function = (char *)(inbuffer + offset-1); 00113 offset += length_function; 00114 this->line |= ((uint32_t) (*(inbuffer + offset + 0))) << (8 * 0); 00115 this->line |= ((uint32_t) (*(inbuffer + offset + 1))) << (8 * 1); 00116 this->line |= ((uint32_t) (*(inbuffer + offset + 2))) << (8 * 2); 00117 this->line |= ((uint32_t) (*(inbuffer + offset + 3))) << (8 * 3); 00118 offset += sizeof(this->line); 00119 uint8_t topics_lengthT = *(inbuffer + offset++); 00120 if(topics_lengthT > topics_length) 00121 this->topics = (char**)realloc(this->topics, topics_lengthT * sizeof(char*)); 00122 offset += 3; 00123 topics_length = topics_lengthT; 00124 for( uint8_t i = 0; i < topics_length; i++){ 00125 uint32_t length_st_topics = *(uint32_t *)(inbuffer + offset); 00126 offset += 4; 00127 for(unsigned int k= offset; k< offset+length_st_topics; ++k){ 00128 inbuffer[k-1]=inbuffer[k]; 00129 } 00130 inbuffer[offset+length_st_topics-1]=0; 00131 this->st_topics = (char *)(inbuffer + offset-1); 00132 offset += length_st_topics; 00133 memcpy( &(this->topics[i]), &(this->st_topics), sizeof(char*)); 00134 } 00135 return offset; 00136 } 00137 00138 virtual const char * getType(){ return "rosgraph_msgs/Log"; }; 00139 virtual const char * getMD5(){ return "acffd30cd6b6de30f120938c17c593fb"; }; 00140 00141 }; 00142 00143 } 00144 #endif
Generated on Fri Jul 15 2022 14:04:54 by
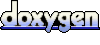