This program is porting rosserial_arduino for mbed http://www.ros.org/wiki/rosserial_arduino This program supported the revision of 169 of rosserial.
Dependents: rosserial_mbed robot_S2
TopicInfo.h
00001 #ifndef _ROS_rosserial_msgs_TopicInfo_h 00002 #define _ROS_rosserial_msgs_TopicInfo_h 00003 00004 #include <stdint.h> 00005 #include <string.h> 00006 #include <stdlib.h> 00007 #include "ros/msg.h" 00008 00009 namespace rosserial_msgs 00010 { 00011 00012 class TopicInfo : public ros::Msg 00013 { 00014 public: 00015 uint16_t topic_id; 00016 char * topic_name; 00017 char * message_type; 00018 char * md5sum; 00019 int32_t buffer_size; 00020 enum { ID_PUBLISHER = 0 }; 00021 enum { ID_SUBSCRIBER = 1 }; 00022 enum { ID_SERVICE_SERVER = 2 }; 00023 enum { ID_SERVICE_CLIENT = 4 }; 00024 enum { ID_PARAMETER_REQUEST = 6 }; 00025 enum { ID_LOG = 7 }; 00026 enum { ID_TIME = 10 }; 00027 00028 virtual int serialize(unsigned char *outbuffer) const 00029 { 00030 int offset = 0; 00031 *(outbuffer + offset + 0) = (this->topic_id >> (8 * 0)) & 0xFF; 00032 *(outbuffer + offset + 1) = (this->topic_id >> (8 * 1)) & 0xFF; 00033 offset += sizeof(this->topic_id); 00034 uint32_t * length_topic_name = (uint32_t *)(outbuffer + offset); 00035 *length_topic_name = strlen( (const char*) this->topic_name); 00036 offset += 4; 00037 memcpy(outbuffer + offset, this->topic_name, *length_topic_name); 00038 offset += *length_topic_name; 00039 uint32_t * length_message_type = (uint32_t *)(outbuffer + offset); 00040 *length_message_type = strlen( (const char*) this->message_type); 00041 offset += 4; 00042 memcpy(outbuffer + offset, this->message_type, *length_message_type); 00043 offset += *length_message_type; 00044 uint32_t * length_md5sum = (uint32_t *)(outbuffer + offset); 00045 *length_md5sum = strlen( (const char*) this->md5sum); 00046 offset += 4; 00047 memcpy(outbuffer + offset, this->md5sum, *length_md5sum); 00048 offset += *length_md5sum; 00049 union { 00050 int32_t real; 00051 uint32_t base; 00052 } u_buffer_size; 00053 u_buffer_size.real = this->buffer_size; 00054 *(outbuffer + offset + 0) = (u_buffer_size.base >> (8 * 0)) & 0xFF; 00055 *(outbuffer + offset + 1) = (u_buffer_size.base >> (8 * 1)) & 0xFF; 00056 *(outbuffer + offset + 2) = (u_buffer_size.base >> (8 * 2)) & 0xFF; 00057 *(outbuffer + offset + 3) = (u_buffer_size.base >> (8 * 3)) & 0xFF; 00058 offset += sizeof(this->buffer_size); 00059 return offset; 00060 } 00061 00062 virtual int deserialize(unsigned char *inbuffer) 00063 { 00064 int offset = 0; 00065 this->topic_id |= ((uint16_t) (*(inbuffer + offset + 0))) << (8 * 0); 00066 this->topic_id |= ((uint16_t) (*(inbuffer + offset + 1))) << (8 * 1); 00067 offset += sizeof(this->topic_id); 00068 uint32_t length_topic_name = *(uint32_t *)(inbuffer + offset); 00069 offset += 4; 00070 for(unsigned int k= offset; k< offset+length_topic_name; ++k){ 00071 inbuffer[k-1]=inbuffer[k]; 00072 } 00073 inbuffer[offset+length_topic_name-1]=0; 00074 this->topic_name = (char *)(inbuffer + offset-1); 00075 offset += length_topic_name; 00076 uint32_t length_message_type = *(uint32_t *)(inbuffer + offset); 00077 offset += 4; 00078 for(unsigned int k= offset; k< offset+length_message_type; ++k){ 00079 inbuffer[k-1]=inbuffer[k]; 00080 } 00081 inbuffer[offset+length_message_type-1]=0; 00082 this->message_type = (char *)(inbuffer + offset-1); 00083 offset += length_message_type; 00084 uint32_t length_md5sum = *(uint32_t *)(inbuffer + offset); 00085 offset += 4; 00086 for(unsigned int k= offset; k< offset+length_md5sum; ++k){ 00087 inbuffer[k-1]=inbuffer[k]; 00088 } 00089 inbuffer[offset+length_md5sum-1]=0; 00090 this->md5sum = (char *)(inbuffer + offset-1); 00091 offset += length_md5sum; 00092 union { 00093 int32_t real; 00094 uint32_t base; 00095 } u_buffer_size; 00096 u_buffer_size.base = 0; 00097 u_buffer_size.base |= ((uint32_t) (*(inbuffer + offset + 0))) << (8 * 0); 00098 u_buffer_size.base |= ((uint32_t) (*(inbuffer + offset + 1))) << (8 * 1); 00099 u_buffer_size.base |= ((uint32_t) (*(inbuffer + offset + 2))) << (8 * 2); 00100 u_buffer_size.base |= ((uint32_t) (*(inbuffer + offset + 3))) << (8 * 3); 00101 this->buffer_size = u_buffer_size.real; 00102 offset += sizeof(this->buffer_size); 00103 return offset; 00104 } 00105 00106 virtual const char * getType(){ return "rosserial_msgs/TopicInfo"; }; 00107 virtual const char * getMD5(){ return "63aa5e8f1bdd6f35c69fe1a1b9d28e9f"; }; 00108 00109 }; 00110 00111 } 00112 #endif
Generated on Fri Jul 15 2022 14:04:54 by
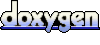