
Embed:
(wiki syntax)
Show/hide line numbers
main.cpp
00001 /** 00002 * digitalInOut.pde 00003 * RTno is RT-middleware and arduino. 00004 * 00005 * Using RTno, arduino device can communicate any RT-components 00006 * through the RTno-proxy component which is launched in PC. 00007 * Connect arduino with USB, and program with RTno library. 00008 * You do not have to define any protocols to establish communication 00009 * between arduino and PC. 00010 * 00011 * Using RTno, you must not define the function "setup" and "loop". 00012 * Those functions are automatically defined in the RTno libarary. 00013 * You, developers, must define following functions: 00014 * int onInitialize(void); 00015 * int onActivated(void); 00016 * int onDeactivated(void); 00017 * int onExecute(void); 00018 * int onError(void); 00019 * int onReset(void); 00020 * These functions are spontaneously called by the RTno-proxy 00021 * RT-component which is launched in the PC. 00022 */ 00023 00024 #include "mbed.h" 00025 #include "RTno.h" 00026 #include "Serial.h" 00027 DigitalIn inPorts[] = {(p5), (p6),(p7),(p8),(p9),(p10)}; 00028 DigitalOut outPorts[] = {(p15),(p16),(p17),(p18),(p19),(p20)}; 00029 DigitalOut leds[] = {(LED1),(LED2),(LED3),(LED4)}; 00030 00031 /** 00032 * This function is called at first. 00033 * conf._default.baudrate: baudrate of serial communication 00034 * exec_cxt.periodic.type: reserved but not used. 00035 */ 00036 void rtcconf(void) { 00037 conf._default.baudrate = 115200; 00038 exec_cxt.periodic.type = ProxySynchronousExecutionContext; 00039 } 00040 00041 /** 00042 * Declaration Division: 00043 * 00044 * DataPort and Data Buffer should be placed here. 00045 * 00046 * Currently, following 6 types are available. 00047 * TimedLong: 00048 * TimedDouble: 00049 * TimedFloat: 00050 * TimedLongSeq: 00051 * TimedDoubleSeq: 00052 * TimedFloatSeq: 00053 * 00054 * Please refer following comments. If you need to use some ports, 00055 * uncomment the line you want to declare. 00056 **/ 00057 00058 TimedLongSeq led; 00059 InPort ledIn("led", led); 00060 00061 TimedLongSeq in0; 00062 InPort in0In("in0", in0); 00063 00064 TimedLongSeq out0; 00065 OutPort out0Out("out0", out0); 00066 00067 00068 ////////////////////////////////////////// 00069 // on_initialize 00070 // 00071 // This function is called in the initialization 00072 // sequence. The sequence is triggered by the 00073 // PC. When the RTnoRTC is launched in the PC, 00074 // then, this function is remotely called 00075 // through the USB cable. 00076 // In on_initialize, usually DataPorts are added. 00077 // 00078 ////////////////////////////////////////// 00079 int RTno::onInitialize() { 00080 /* Data Ports are added in this section. 00081 */ 00082 addInPort(ledIn); 00083 addInPort(in0In); 00084 addOutPort(out0Out); 00085 00086 // Some initialization (like port direction setting) 00087 00088 return RTC_OK; 00089 } 00090 00091 //////////////////////////////////////////// 00092 // on_activated 00093 // This function is called when the RTnoRTC 00094 // is activated. When the activation, the RTnoRTC 00095 // sends message to call this function remotely. 00096 // If this function is failed (return value 00097 // is RTC_ERROR), RTno will enter ERROR condition. 00098 //////////////////////////////////////////// 00099 int RTno::onActivated() { 00100 // Write here initialization code. 00101 00102 return RTC_OK; 00103 } 00104 00105 ///////////////////////////////////////////// 00106 // on_deactivated 00107 // This function is called when the RTnoRTC 00108 // is deactivated. 00109 ///////////////////////////////////////////// 00110 int RTno::onDeactivated() { 00111 // Write here finalization code. 00112 00113 return RTC_OK; 00114 } 00115 00116 ////////////////////////////////////////////// 00117 // This function is repeatedly called when the 00118 // RTno is in the ACTIVE condition. 00119 // If this function is failed (return value is 00120 // RTC_ERROR), RTno immediately enter into the 00121 // ERROR condition.r 00122 ////////////////////////////////////////////// 00123 int RTno::onExecute() { 00124 00125 /* 00126 * Input digital data 00127 */ 00128 00129 if (ledIn.isNew()) { 00130 ledIn.read(); 00131 for (int i = 0; i < led.data.length() && i < 4; i++) { 00132 leds[i] =led.data[i]; 00133 } 00134 } 00135 00136 00137 if (in0In.isNew()) { 00138 in0In.read(); 00139 for (int i = 0; i < in0.data.length() && i < 6; i++) { 00140 outPorts[i] =in0.data[i]; 00141 } 00142 } 00143 00144 00145 /* 00146 * Output digital data in Voltage unit. 00147 */ 00148 out0.data.length(6); 00149 for (int i = 0; i < 6; i++) { 00150 out0.data[i] = inPorts[i]; 00151 } 00152 out0Out.write(); 00153 00154 return RTC_OK; 00155 } 00156 00157 00158 ////////////////////////////////////// 00159 // on_error 00160 // This function is repeatedly called when 00161 // the RTno is in the ERROR condition. 00162 // The ERROR condition can be recovered, 00163 // when the RTno is reset. 00164 /////////////////////////////////////// 00165 int RTno::onError() { 00166 return RTC_OK; 00167 } 00168 00169 //////////////////////////////////////// 00170 // This function is called when 00171 // the RTno is reset. If on_reset is 00172 // succeeded, the RTno will enter into 00173 // the INACTIVE condition. If failed 00174 // (return value is RTC_ERROR), RTno 00175 // will stay in ERROR condition.ec 00176 /////////////////////////////////////// 00177 int RTno::onReset() { 00178 return RTC_OK; 00179 }
Generated on Wed Jul 13 2022 13:00:00 by
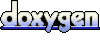