
633 solution updated for mbed os 54
Fork of Task633Solution-mbedos54 by
main.cpp
00001 #include "mbed.h" 00002 #include "string.h" 00003 #include <stdio.h> 00004 #include <ctype.h> 00005 00006 #define SWITCH1_RELEASE 1 00007 00008 void thread1(); 00009 void thread2(); 00010 void switchISR(); 00011 00012 //Analogue inputs 00013 AnalogIn adcIn(A0); 00014 00015 //Digital outputs 00016 DigitalOut onBoardLED(LED1); 00017 DigitalOut redLED(D7); 00018 DigitalOut yellowLED(D6); 00019 DigitalOut greenLED(D5); 00020 00021 //Digital inputs 00022 DigitalIn onBoardSwitch(USER_BUTTON); 00023 DigitalIn sw1(D4); //CONSIDER CHANGING THIS TO AN INTERRUPT 00024 DigitalIn sw2(D3); 00025 00026 //Threads 00027 Thread *t1; 00028 00029 //Class type 00030 class message_t { 00031 public: 00032 float adcValue; 00033 int sw1State; 00034 int sw2State; 00035 00036 //Constructor 00037 message_t(float f, int s1, int s2) { 00038 adcValue = f; 00039 sw1State = s1; 00040 sw2State = s2; 00041 } 00042 }; 00043 00044 //Memory Pool - with capacity for 16 message_t types 00045 //MemoryPool<message_t, 16> mpool; 00046 00047 //Message queue - matched to the memory pool 00048 //Queue<message_t, 16> queue; 00049 00050 //Mail queue 00051 Mail<message_t, 16> mail_box; 00052 00053 00054 // Call this on precise intervals 00055 void adcISR() { 00056 00057 00058 //Read sample - make a copy 00059 float sample = adcIn; 00060 //Grab switch state 00061 uint32_t switch1State = sw1; 00062 uint32_t switch2State = sw2; 00063 00064 //Allocate a block from the memory pool 00065 message_t *message = mail_box.alloc(); 00066 if (message == NULL) { 00067 //Out of memory 00068 printf("Out of memory\n\r"); 00069 redLED = 1; 00070 return; 00071 } 00072 00073 //Fill in the data 00074 message->adcValue = sample; 00075 message->sw1State = switch1State; 00076 message->sw2State = switch2State; 00077 00078 //Write to queue 00079 osStatus stat = mail_box.put(message); //Note we are sending the "pointer" 00080 00081 //Check if succesful 00082 if (stat == osErrorResource) { 00083 redLED = 1; 00084 printf("queue->put() Error code: %4Xh, Resource not available\r\n", stat); 00085 mail_box.free(message); 00086 return; 00087 } 00088 00089 00090 } 00091 00092 //Normal priority thread (consumer) 00093 void thread1() 00094 { 00095 static int count = 0; 00096 00097 while (true) { 00098 //Block on the queue 00099 osEvent evt = mail_box.get(); 00100 00101 //Check status 00102 if (evt.status == osEventMail) { 00103 message_t *pMessage = (message_t*)evt.value.p; //This is the pointer (address) 00104 //Make a copy 00105 message_t msg(pMessage->adcValue, pMessage->sw1State, pMessage->sw2State); 00106 //We are done with this, so give back the memory to the pool 00107 mail_box.free(pMessage); 00108 00109 //Echo to the terminal 00110 printf("ADC Value: %.2f\t", msg.adcValue); 00111 printf("SW1: %u\t", msg.sw1State); 00112 printf("SW2: %u\n\r", msg.sw2State); 00113 00114 //Update state 00115 if ((msg.sw1State == 1) && (msg.sw2State == 1)) { 00116 count++; 00117 } else { 00118 count = 0; 00119 } 00120 if (count == 10) { 00121 greenLED = !greenLED; 00122 count = 0; 00123 } 00124 } else { 00125 printf("ERROR: %x\n\r", evt.status); 00126 } 00127 00128 } //end while 00129 } 00130 00131 00132 // Main thread 00133 int main() { 00134 redLED = 0; 00135 yellowLED = 0; 00136 greenLED = 0; 00137 00138 //Start message 00139 printf("Welcome\n"); 00140 00141 //Hook up timer interrupt 00142 Ticker timer; 00143 timer.attach(&adcISR, 0.1); 00144 00145 //Threads 00146 t1 = new Thread(); 00147 t1->start(thread1); 00148 00149 printf("Main Thread\n"); 00150 while (true) { 00151 Thread::wait(5000); 00152 puts("Main Thread Alive"); 00153 } 00154 } 00155 00156
Generated on Sun Jul 17 2022 02:35:07 by
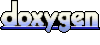