
Comparing a wait with yield vs a polling-busy-wait
Fork of Task621-mbedos54 by
main.cpp
00001 #include "mbed.h" 00002 #include "rtos.h" 00003 #include "string.h" 00004 #include <stdio.h> 00005 #include <ctype.h> 00006 00007 #define DELAY 200 00008 00009 //Digital outputs 00010 DigitalOut onBoardLED(LED1); 00011 DigitalOut redLED(D7); 00012 DigitalOut yellowLED(D6); 00013 DigitalOut greenLED(D5); 00014 00015 //Serial Interface 00016 Serial pc(USBTX, USBRX); 00017 00018 //Digital inputs 00019 DigitalIn onBoardSwitch(USER_BUTTON); 00020 DigitalIn SW1(D4); 00021 DigitalIn SW2(D3); 00022 00023 //Thread ID for the Main function (CMSIS API) 00024 osThreadId tidMain; 00025 00026 void thread1() 00027 { 00028 pc.printf("Entering thread 1\n"); 00029 while (true) { 00030 yellowLED = 1; 00031 Thread::wait(DELAY); 00032 yellowLED = 0; 00033 Thread::wait(DELAY); 00034 } 00035 } 00036 00037 //This thread has higher priority 00038 void thread2() 00039 { 00040 pc.printf("Entering thread 2\n"); 00041 while (true) { 00042 redLED = 1; 00043 if (SW1 == 1) { 00044 00045 // 1) Select the 'type' of wait 00046 00047 //wait_ms(osWaitForever); 00048 Thread::wait(osWaitForever); 00049 } else { 00050 Thread::wait(DELAY); 00051 } 00052 redLED = 0; 00053 Thread::wait(DELAY); 00054 } 00055 } 00056 00057 00058 //Main thread 00059 int main() { 00060 redLED = 0; 00061 yellowLED = 0; 00062 greenLED = 0; 00063 00064 //Main thread ID 00065 tidMain = Thread::gettid(); 00066 00067 Thread t1(osPriorityNormal); 00068 t1.start(thread1); 00069 00070 // 2) Select the Thread Priority 00071 00072 //Thread t2(thread2, NULL, osPriorityNormal); 00073 Thread t2(osPriorityAboveNormal); 00074 t2.start(thread2); 00075 00076 pc.printf("Main Thread\n"); 00077 while (true) { 00078 Thread::wait(osWaitForever); 00079 } 00080 00081 } 00082 00083
Generated on Mon Jul 25 2022 05:44:40 by
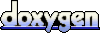