
Task 5.2.5 Solution
Embed:
(wiki syntax)
Show/hide line numbers
main.cpp
00001 #include "mbed.h" 00002 00003 //Global PWM object 00004 PwmOut pwmRed(D7); 00005 00006 InterruptIn SWOn(D4); //Fade ON 00007 InterruptIn SWOff(D3); //Fade OFF 00008 00009 int T = 100; //100uS 00010 volatile int Tmark = 0; //0us 00011 volatile int delta = 1; //1us 00012 00013 //Timer 00014 Ticker t; 00015 00016 //Function prototype 00017 void doTwinkle(); 00018 void doSwitchON(); 00019 void doSwitchOFF(); 00020 00021 //Flags 00022 volatile int switchONFlag=0; 00023 volatile int switchOFFFlag=0; 00024 volatile int tickerFlag = 0; 00025 00026 int main() { 00027 //Setup switch interrupts 00028 SWOn.rise(doSwitchON); 00029 SWOff.rise(doSwitchOFF); 00030 00031 //Initial PWM state 00032 pwmRed.period_us(T); 00033 pwmRed.pulsewidth_us(Tmark); 00034 00035 00036 printf("Ready\n"); 00037 00038 while(1) { 00039 sleep(); 00040 00041 //Which interrupt occured? 00042 if (switchONFlag == 1) { 00043 switchONFlag = 0; 00044 delta = 5; 00045 t.attach(doTwinkle, 0.1); 00046 printf("ON button pressed\n"); 00047 } 00048 else if (switchOFFFlag == 1) { 00049 switchOFFFlag = 0; 00050 delta = -5; 00051 t.attach(doTwinkle, 0.1); 00052 printf("OFF button pressed\n"); 00053 } 00054 00055 //Has ticker hit the end stop? 00056 if (tickerFlag == 1) { 00057 tickerFlag = 0; 00058 delta = 0; //Pedantic 00059 t.detach(); //Turn off ticker 00060 printf("Timer finished\n"); 00061 } 00062 //Update PWM 00063 pwmRed.pulsewidth_us(Tmark); 00064 00065 printf("Tmark = %d\n", Tmark); //Debug 00066 } 00067 } 00068 00069 void doSwitchON() 00070 { 00071 switchONFlag=1; 00072 } 00073 00074 void doSwitchOFF() 00075 { 00076 switchOFFFlag=1; 00077 } 00078 00079 //ISR for Timer 00080 void doTwinkle() 00081 { 00082 //Add on delta 00083 Tmark += delta; 00084 00085 //Cap at extremes 00086 if (Tmark > 100) { 00087 Tmark = 100; 00088 } 00089 else if (Tmark < 0) { 00090 Tmark = 0; 00091 } 00092 00093 //Check bounds 00094 if ((Tmark == 100) || (Tmark == 0)) { 00095 tickerFlag = 1; 00096 } 00097 }
Generated on Thu Aug 4 2022 07:13:23 by
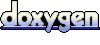