
Task 1.3.2 Solution
Embed:
(wiki syntax)
Show/hide line numbers
main.cpp
00001 //This is known as a “header file” 00002 //In short, this copies and pastes the text file 00003 //mbed.h into this code 00004 #include "mbed.h" 00005 00006 //Create a DigitalOut “object” called myled 00007 //Pass constant D7 as a “parameter” 00008 DigitalOut redLED(D7); 00009 DigitalOut yellowLED(D6); 00010 DigitalOut greenLED(D5); 00011 00012 //The main function - all executable C / C++ 00013 //applications have a main function. This is 00014 //out entry point in the software 00015 int main() { 00016 00017 redLED = 0; 00018 yellowLED = 0; 00019 greenLED = 0; 00020 00021 // ALL the code is contained in a 00022 // “while loop" 00023 00024 00025 while(1) 00026 { 00027 //The code between the { curly braces } 00028 //is the code that is repeated 00029 00030 //STATE 1 (R) 00031 redLED = 1; 00032 yellowLED = 0; 00033 greenLED = 0; 00034 wait(1.0); 00035 00036 //STATE 2 (RA) 00037 yellowLED = 1; 00038 wait(1.0); 00039 00040 //STATE 3 (G) 00041 redLED = 0; 00042 yellowLED = 0; 00043 greenLED = 1; 00044 wait(1.0); 00045 00046 //STATE 4 (A) 00047 yellowLED = 1; 00048 greenLED = 0; 00049 wait(1.0); 00050 } 00051 }
Generated on Sat Jul 23 2022 08:45:38 by
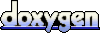