
This is a sample program to use a rocker IS switch from NKK. It reads a file from SD card and send to the switch. Supported file format is the one that is used by a NKK\'s editor for IS series switches. Part of this program is derived from a sample program for PIC micro cotnroller written by NKK.
Dependencies: mbed SDFileSystem
main.cpp
00001 /* 00002 Sample program to use a rocker IS switch from NKK. 00003 Part of this program is derived from a sample program for PIC 00004 micro cotnroller written by NKK. 00005 */ 00006 00007 #include "mbed.h" 00008 #include "SDFileSystem.h" 00009 00010 SDFileSystem sd(p5, p6, p7, p8, "sd"); 00011 SPI spi(p11, p12, p13); // mosi(SDO), miso(SDI), sclk(SCLK) 00012 DigitalOut is_cs(p21); 00013 DigitalOut is_reset(p22); 00014 DigitalOut is_dc(p23); 00015 DigitalOut is_vcc(p24); 00016 DigitalOut led1(LED1); 00017 DigitalIn sw1(p18); 00018 DigitalIn sw2(p19); 00019 DigitalIn sw3(p20); 00020 00021 void OledSendCommand( const unsigned char *com ); 00022 void OledInit( void ); 00023 void OledSendData( const unsigned char *dat, int len ); 00024 00025 const int DAT = 1; 00026 const int CMD = 0; 00027 00028 unsigned char dat[96*64/8]; 00029 const unsigned char cls[96*64/8] = {0}; 00030 00031 int main() { 00032 sw1.mode(PullUp); 00033 sw2.mode(PullUp); 00034 sw3.mode(PullUp); 00035 // Setup the spi for 8 bit data, high steady state clock, 00036 // second edge capture, with a 4MHz clock rate 00037 spi.format(8, 3); 00038 spi.frequency(4000000); 00039 00040 is_reset = 0; 00041 is_vcc = 0; 00042 00043 OledInit(); 00044 00045 for (;;) { 00046 DIR *d; 00047 struct dirent *p; 00048 d = opendir("/sd/bw"); 00049 if (d != NULL) { 00050 while ((p = readdir(d)) != NULL) { 00051 char fname[20]; 00052 sprintf(fname, "/sd/bw/%s", p->d_name); 00053 FILE *fp = fopen(fname, "rb"); 00054 if (fp == NULL) { 00055 // error("Could not open file for read\n"); 00056 } else { 00057 fread(dat, 1, 8, fp); // skip header 00058 fread(dat, 1, sizeof(dat), fp); 00059 fclose(fp); 00060 OledSendData(dat, 96*64/8); 00061 wait_ms(100); 00062 while (sw1 && sw2 && sw3) 00063 ; 00064 } 00065 } 00066 closedir(d); 00067 } 00068 } 00069 } 00070 00071 ////////////////////////////////////////////////////////////////////// 00072 ////////////////////////////////////////////////////////////////////// 00073 // OLED controll commands (Initial settings commands) 00074 const unsigned char SoftwareReset[] = { 0x01, 0x01 }; 00075 const unsigned char DotMatrixDisplay[] = { 0x02, 0x02, 0x00 }; 00076 const unsigned char SetRWOperation[] = { 0x02, 0x07, 0x00 }; 00077 const unsigned char SetDisplayDirection[] = { 0x02, 0x09, 0x00 }; 00078 const unsigned char DotMatrixStandby[] = { 0x02, 0x14, 0x00 }; 00079 const unsigned char GraphicMemoryWrite[] = { 0x02, 0x1d, 0x00 }; 00080 const unsigned char SetupColumnAddress[] = { 0x03, 0x30, 0x00, 0x5f }; 00081 const unsigned char SetupRowAddress[] = { 0x03, 0x32, 0x00, 0x3f }; 00082 const unsigned char XaxisRWStartPoint[] = { 0x02, 0x34, 0x00 }; 00083 const unsigned char XaxisRWEndPoint[] = { 0x02, 0x35, 0x0b }; 00084 const unsigned char YaxisRWStartPoint[] = { 0x02, 0x36, 0x00 }; 00085 const unsigned char YaxisRWEndPoint[] = { 0x02, 0x37, 0x3f }; 00086 const unsigned char XaxisRStartAddress[] = { 0x02, 0x38, 0x00 }; 00087 const unsigned char YaxisRStartAddress[] = { 0x02, 0x39, 0x00 }; 00088 const unsigned char SetScreenSaverTimer1[] = { 0x02, 0xc3, 0x00 }; 00089 const unsigned char SetScreenSaverTimer2[] = { 0x02, 0xc4, 0x00 }; 00090 const unsigned char RepeatScreenSaver[] = { 0x02, 0xcc, 0x00 }; 00091 const unsigned char StartStopScreenSaver[] = { 0x02, 0xcd, 0x00 }; 00092 const unsigned char SetSystemClock[] = { 0x02, 0xd0, 0x80 }; 00093 const unsigned char SetSTBYPin[] = { 0x02, 0xd2, 0x00 }; 00094 const unsigned char DACASetting[] = { 0x02, 0xd4, 0x00 }; 00095 const unsigned char DACBSetting[] = { 0x02, 0xd5, 0x00 }; 00096 const unsigned char DACCSetting[] = { 0x02, 0xd6, 0x00 }; 00097 const unsigned char DACDSetting[] = { 0x02, 0xd7, 0x00 }; 00098 const unsigned char DimmerSetting[] = { 0x02, 0xdb, 0x0f }; 00099 00100 const unsigned char Reserved1[] = { 0x02, 0x10, 0x03 }; 00101 const unsigned char Reserved2[] = { 0x02, 0x12, 0x63 }; 00102 const unsigned char Reserved3[] = { 0x02, 0x13, 0x00 }; 00103 const unsigned char Reserved4[] = { 0x02, 0x16, 0x00 }; 00104 const unsigned char Reserved5[] = { 0x02, 0x17, 0x00 }; 00105 const unsigned char Reserved6[] = { 0x02, 0x18, 0x09 }; 00106 const unsigned char Reserved7[] = { 0x02, 0x1a, 0x04 }; 00107 const unsigned char Reserved8[] = { 0x02, 0x1c, 0x00 }; 00108 const unsigned char Reserved9[] = { 0x02, 0x48, 0x03 }; 00109 const unsigned char Reserved10[] = { 0x02, 0xd9, 0x00 }; 00110 const unsigned char Reserved11[] = { 0x02, 0xdd, 0x88 }; 00111 00112 const unsigned char SendImage[] = { 0x01, 0x08 }; 00113 const unsigned char DotMatrixDisplayON[] = { 0x02, 0x02, 0x01 }; 00114 00115 // 00116 // initializing OLED 00117 // 00118 void OledInit( void ) { 00119 // reset OLED 00120 is_vcc = 0; 00121 is_reset = 0; 00122 wait_us(3); 00123 is_vcc = 1; 00124 wait_ms(1); 00125 is_reset = 1; 00126 00127 // initial settings 00128 OledSendCommand( SoftwareReset ); 00129 wait_us(3); 00130 OledSendCommand( DotMatrixDisplayON ); 00131 // OledSendCommand( DotMatrixDisplay ); 00132 OledSendCommand( SetRWOperation ); 00133 OledSendCommand( SetDisplayDirection ); 00134 OledSendCommand( Reserved1 ); 00135 OledSendCommand( Reserved2 ); 00136 OledSendCommand( Reserved3 ); 00137 OledSendCommand( DotMatrixStandby ); 00138 OledSendCommand( Reserved4 ); 00139 OledSendCommand( Reserved5 ); 00140 OledSendCommand( Reserved6 ); 00141 OledSendCommand( Reserved7 ); 00142 OledSendCommand( Reserved8 ); 00143 OledSendCommand( GraphicMemoryWrite ); 00144 OledSendCommand( SetupColumnAddress ); 00145 OledSendCommand( SetupRowAddress ); 00146 OledSendCommand( XaxisRWStartPoint ); 00147 OledSendCommand( XaxisRWEndPoint ); 00148 OledSendCommand( YaxisRWStartPoint ); 00149 OledSendCommand( YaxisRWEndPoint ); 00150 OledSendCommand( XaxisRStartAddress ); 00151 OledSendCommand( YaxisRStartAddress ); 00152 OledSendCommand( Reserved9 ); 00153 OledSendCommand( SetScreenSaverTimer1 ); 00154 OledSendCommand( SetScreenSaverTimer2 ); 00155 OledSendCommand( RepeatScreenSaver ); 00156 OledSendCommand( StartStopScreenSaver ); 00157 OledSendCommand( SetSystemClock ); 00158 OledSendCommand( SetSTBYPin ); 00159 OledSendCommand( DACASetting ); 00160 OledSendCommand( DACBSetting ); 00161 OledSendCommand( DACCSetting ); 00162 OledSendCommand( DACDSetting ); 00163 OledSendCommand( Reserved10 ); 00164 OledSendCommand( DimmerSetting ); 00165 OledSendCommand( Reserved11 ); 00166 } 00167 00168 // 00169 // Send a command / data to OLED via SPI 00170 // 00171 void OledSend( const unsigned char *p, int len ) { 00172 for (; len>0; len --, p ++) { 00173 is_cs = 0; 00174 spi.write(*p); 00175 is_cs = 1; 00176 } 00177 } 00178 00179 // 00180 // Send a command to OLED 00181 // 00182 void OledSendCommand( const unsigned char *com ) { 00183 is_dc = CMD; 00184 OledSend(com+1, 1); 00185 is_dc = DAT; 00186 OledSend(com+2, *com-1); 00187 } 00188 00189 // 00190 // Send data to OLED 00191 // 00192 void OledSendData( const unsigned char *dat, int len ) { 00193 OledSendCommand( SendImage ); 00194 is_dc = DAT; 00195 OledSend(dat, len); 00196 OledSendCommand( DotMatrixDisplayON ); 00197 }
Generated on Sat Jul 16 2022 15:50:07 by
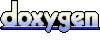