
local fix version of myBlueUSB (http://mbed.org/users/networker/code/myBlueUSB/). - merge deleted files which are required to compile. - enable echo back of received data via RFCOMM.
Dependencies: AvailableMemory FatFileSystem mbed myUSBHost
sdp_data.h
00001 #ifndef SDP_DATA_H 00002 #define SDP_DATA_H 00003 00004 #include <vector> 00005 00006 extern const unsigned char base_uuid[16];// = { 0xfb, 0x34, 0x9b, 0x5f, 0x80, 0, 0x07, 0x70, 0, 0x10, 0, 0}; 00007 00008 class sdp_data { 00009 public: 00010 enum elements { NULL_, UNSIGNED, SIGNED, UUID, STRING, BOOL, SEQUENCE, ALTERNATIVE, URL}; 00011 private: 00012 enum elements type; 00013 char size; 00014 union { 00015 unsigned data; 00016 char *str; 00017 #ifdef LONGUUID 00018 unsigned short uuid[8]; 00019 #endif 00020 }; 00021 vector<sdp_data*> sequence; //not allowed to be in union 00022 static char ret[12]; 00023 char *longstr; 00024 public: 00025 sdp_data(): type(NULL_), size(0), longstr(0) { 00026 //printf("NULL%d ", size); 00027 } 00028 sdp_data(unsigned d, unsigned sz=4): type(UNSIGNED), size(sz), longstr(0) { 00029 data=d; 00030 //printf("UINT%d=%u ", size, data); 00031 } 00032 sdp_data(unsigned short d, unsigned sz=2): type(UNSIGNED), size(sz), longstr(0) { 00033 data=d; 00034 //printf("UINT%d=%u ", size, data); 00035 } 00036 sdp_data(signed d, unsigned sz=4): type(SIGNED), size(sz), longstr(0) { 00037 data=d; 00038 //printf("INT%d=%d ", size, data); 00039 } 00040 sdp_data(bool d, unsigned sz=1): type(BOOL), size(sz), longstr(0) { 00041 data=d; 00042 //printf("BOOL%d=%u ", size, data); 00043 } 00044 sdp_data(char*s, unsigned sz=0): type(STRING), longstr(0) { 00045 if (sz) size = sz+1; 00046 else size = strlen(s)+1; 00047 str = new char[size]; 00048 strncpy(str, s, size); 00049 str[size-1] = '\0'; 00050 //printf("STR%d='%s' ", size, str); 00051 } 00052 sdp_data(enum elements t, unsigned d, unsigned sz=2): type(t), size(sz), longstr(0) { 00053 if (t==UUID) { 00054 #ifdef LONGUUID 00055 memcpy(uuid, base_uuid, 16); 00056 uuid[6] = d; 00057 uuid[7] = d>>16; 00058 // printf("UUID%d=%04X%04X ", size, uuid[7], uuid[6]); 00059 #else 00060 data = d; 00061 #endif 00062 } else printf("Please use other constructor for type %d\n", t); 00063 } 00064 sdp_data(enum elements t, char *d=0, unsigned sz=0): type(t), size(sz), longstr(0) { 00065 switch (t) { 00066 #ifdef LONGUUID 00067 case UUID: 00068 memcpy(uuid, d, size); 00069 // printf("UUID%d=%08X ", size, uuid[6]); 00070 break; 00071 #endif 00072 case URL: 00073 //size = strlen(d)+1; 00074 str = new char[size+1]; 00075 strcpy(str, d); 00076 // printf("URL%d='%u' ", size, str); 00077 break; 00078 case SEQUENCE: 00079 case ALTERNATIVE: 00080 break; 00081 default: 00082 printf("Please use other constructor for type %d\n", t); 00083 } 00084 } 00085 ~sdp_data() { 00086 switch (type) { 00087 case STRING: 00088 case URL: 00089 delete[] str; 00090 break; 00091 case SEQUENCE: 00092 case ALTERNATIVE: 00093 for (int i = 0; i < sequence.size(); i++) 00094 delete sequence.at(i); 00095 break; 00096 } 00097 if (longstr) 00098 delete[] longstr; 00099 } 00100 void add_element(sdp_data *el) { 00101 sequence.push_back(el); 00102 size += el->Size(); 00103 } 00104 unsigned asUnsigned() ; 00105 const char* asString(bool alt=false) ; 00106 unsigned Size() ; 00107 unsigned items() { return sequence.size();} 00108 sdp_data* item(int i) { return sequence[i];} 00109 void remove(int i) { sequence[i] = 0;} 00110 unsigned sizedesc(unsigned char *buf) ; 00111 void revcpy(unsigned char*d, const unsigned char*s, int n) ; 00112 unsigned build(unsigned char *buf, unsigned max) ; 00113 bool findUUID(unsigned uuid); 00114 }; 00115 00116 #endif
Generated on Tue Jul 12 2022 18:48:53 by
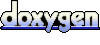