
local fix version of myBlueUSB (http://mbed.org/users/networker/code/myBlueUSB/). - merge deleted files which are required to compile. - enable echo back of received data via RFCOMM.
Dependencies: AvailableMemory FatFileSystem mbed myUSBHost
neighbourhood.cpp
00001 #include "Utils.h" 00002 #include "neighbourhood.h" 00003 00004 neighbourhood *neighbors = 0; 00005 00006 int neighbourhood::get(BD_ADDR *a, unsigned char *key) { 00007 for (list<item>::iterator i = keys.begin(); i != keys.end(); i++) 00008 if (memcmp(a, &(*i).a, sizeof(BD_ADDR)) == 0) { 00009 memcpy(key, (*i).lk, lksize); 00010 #ifdef STRICT_MRU 00011 if (i != keys.begin()) { 00012 keys.push_front(*i); 00013 keys.erase(i); 00014 dirty = true; 00015 } 00016 #endif 00017 return 1; 00018 } 00019 return 0; 00020 } 00021 00022 int neighbourhood::add(BD_ADDR *a, const unsigned char *key, bool init) { 00023 for (list<item>::iterator i = keys.begin(); i != keys.end(); i++) 00024 if (memcmp(a, &(*i).a, sizeof(BD_ADDR)) == 0) { 00025 memcpy((*i).lk, key, lksize); //assume key has changed, update key 00026 (*i).used = true; 00027 return 1; 00028 } 00029 //new key 00030 printf("Neighbourhood: "); printf(a); printf("\n"); 00031 if (keys.size() < cap) { 00032 keys.push_back(item(a, key, !init));//append as long as there is space 00033 } else { 00034 keys.push_front(item(a, key, true));//otherwise prepend 00035 dirty = true; 00036 } 00037 return 0; 00038 } 00039 00040 void neighbourhood::write() { 00041 int n = 0; 00042 static const int maxkey = 11; 00043 unsigned char param[maxkey*(lksize+sizeof(BD_ADDR))+1]; 00044 int k = keys.size()-cap; 00045 list<item>::iterator i = keys.begin(); 00046 while (i != keys.end()) { 00047 if (k>0) { 00048 if (!(*i).used) { 00049 delete_link_key(&(*i).a);//try to make some room 00050 keys.erase(i); 00051 k--; 00052 } else 00053 i++; 00054 } else 00055 break; 00056 } 00057 //hci->delete_link_keys(); 00058 unsigned char *p = ¶m[1]; 00059 for (list<item>::iterator i = keys.begin(); i != keys.end() && n<maxkey; i++, n++) { 00060 memcpy(p, &(*i).a, sizeof(BD_ADDR)); 00061 p += sizeof(BD_ADDR); 00062 memcpy(p, (*i).lk, lksize); 00063 p += lksize; 00064 } 00065 param[0] = n; 00066 if (n > 0) 00067 write_link_keys(param); 00068 } 00069
Generated on Tue Jul 12 2022 18:48:53 by
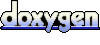