
local fix version of myBlueUSB (http://mbed.org/users/networker/code/myBlueUSB/). - merge deleted files which are required to compile. - enable echo back of received data via RFCOMM.
Dependencies: AvailableMemory FatFileSystem mbed myUSBHost
main.cpp
00001 /* 00002 Copyright (c) 2010 Peter Barrett 00003 00004 Permission is hereby granted, free of charge, to any person obtaining a copy 00005 of this software and associated documentation files (the "Software"), to deal 00006 in the Software without restriction, including without limitation the rights 00007 to use, copy, modify, merge, publish, distribute, sublicense, and/or sell 00008 copies of the Software, and to permit persons to whom the Software is 00009 furnished to do so, subject to the following conditions: 00010 00011 The above copyright notice and this permission notice shall be included in 00012 all copies or substantial portions of the Software. 00013 00014 THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR 00015 IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, 00016 FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE 00017 AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER 00018 LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, 00019 OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN 00020 THE SOFTWARE. 00021 */ 00022 00023 #include "mbed.h" 00024 #include "USBHost.h" 00025 #include "Utils.h" 00026 #include "FATFileSystem.h" 00027 #include "MassStorage.h" 00028 00029 int MassStorage_ReadCapacity(int device, u32* blockCount, u32* blockSize); 00030 int MassStorage_Read(int device, u32 blockAddr, u32 blockCount, u8* dst, u32 blockSize); 00031 int MassStorage_Write(int device, u32 blockAddr, u32 blockCount, u8* dst, u32 blockSize); 00032 00033 class USBFileSystem : public FATFileSystem, public USBSCSI 00034 { 00035 //int _device; 00036 u32 _blockSize; 00037 u32 _blockCount; 00038 00039 public: 00040 USBFileSystem() : FATFileSystem("usb")/*,_device(0)*/,_blockSize(0),_blockCount(0) 00041 { 00042 } 00043 /* 00044 void SetDevice(int device, unsigned char in, unsigned char out) 00045 { 00046 _device = device; 00047 } 00048 */ 00049 virtual int disk_initialize() 00050 { 00051 return SCSIReadCapacity(&_blockCount,&_blockSize); 00052 //return MassStorage_ReadCapacity(_device,&_blockCount,&_blockSize); 00053 } 00054 00055 virtual int disk_write(const char *buffer, int block_number) 00056 { 00057 return SCSITransfer(block_number, 1,(u8*)buffer,_blockSize,HOST_TO_DEVICE); 00058 //return MassStorage_Write(_device,block_number,1,(u8*)buffer,_blockSize); 00059 } 00060 00061 virtual int disk_read(char *buffer, int block_number) 00062 { 00063 return SCSITransfer(block_number, 1, (u8*)buffer, _blockSize, DEVICE_TO_HOST); 00064 //return MassStorage_Read(_device,block_number,1,(u8*)buffer,_blockSize); 00065 } 00066 00067 virtual int disk_sectors() 00068 { 00069 return _blockCount; 00070 } 00071 }; 00072 00073 void DumpFS(int depth, int count) 00074 { 00075 DIR *d = opendir("/usb"); 00076 if (!d) 00077 { 00078 printf("USB file system borked\n"); 00079 return; 00080 } 00081 00082 printf("\nDumping root dir\n"); 00083 struct dirent *p; 00084 for(;;) 00085 { 00086 p = readdir(d); 00087 if (!p) 00088 break; 00089 int len = sizeof( dirent); 00090 printf("%s %d\n", p->d_name, len); 00091 } 00092 closedir(d); 00093 } 00094 00095 int OnDiskInsert(int device, unsigned char in, unsigned char out) 00096 { 00097 USBFileSystem fs; 00098 fs.SetDevice(device, in, out); 00099 DumpFS(0,0); 00100 return 0; 00101 } 00102 00103 /* 00104 Simple test shell to exercise mouse,keyboard,mass storage and hubs. 00105 Add 2 15k pulldown resistors between D+/D- and ground, attach a usb socket and have at it. 00106 */ 00107 00108 Serial pc(USBTX, USBRX); 00109 int GetConsoleChar() 00110 { 00111 if (!pc.readable()) 00112 return -1; 00113 char c = pc.getc(); 00114 pc.putc(c); // echo 00115 return c; 00116 } 00117 00118 void TestShell(); 00119 00120 int main() 00121 { 00122 pc.baud(460800); 00123 printf("BlueUSB\nNow get a bunch of usb or bluetooth things and plug them in\n"); 00124 TestShell(); 00125 }
Generated on Tue Jul 12 2022 18:48:53 by
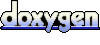