
local fix version of myBlueUSB (http://mbed.org/users/networker/code/myBlueUSB/). - merge deleted files which are required to compile. - enable echo back of received data via RFCOMM.
Dependencies: AvailableMemory FatFileSystem mbed myUSBHost
MassStorage.cpp
00001 00002 /* 00003 Copyright (c) 2010 Peter Barrett 00004 00005 Permission is hereby granted, free of charge, to any person obtaining a copy 00006 of this software and associated documentation files (the "Software"), to deal 00007 in the Software without restriction, including without limitation the rights 00008 to use, copy, modify, merge, publish, distribute, sublicense, and/or sell 00009 copies of the Software, and to permit persons to whom the Software is 00010 furnished to do so, subject to the following conditions: 00011 00012 The above copyright notice and this permission notice shall be included in 00013 all copies or substantial portions of the Software. 00014 00015 THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR 00016 IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, 00017 FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE 00018 AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER 00019 LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, 00020 OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN 00021 THE SOFTWARE. 00022 */ 00023 00024 #include "stdlib.h" 00025 #include "stdio.h" 00026 #include "string.h" 00027 00028 #include "MassStorage.h" 00029 00030 #if 0 00031 int SCSIRequestSense(int device); 00032 00033 int DoSCSI(int device, const u8* cmd, int cmdLen, int flags, u8* data, u32 transferLen) { 00034 CBW cbw; 00035 cbw.Signature = CBW_SIGNATURE; 00036 cbw.Tag = 0; 00037 cbw.TransferLength = transferLen; 00038 cbw.Flags = flags; 00039 cbw.LUN = 0; 00040 cbw.CBLength = cmdLen; 00041 memset(cbw.CB,0,sizeof(cbw.CB)); 00042 memcpy(cbw.CB,cmd,cmdLen); 00043 00044 int r; 00045 //r = USBBulkTransfer(device,0x01,(u8*)&cbw,31); // Send the command 00046 r = USBBulkTransfer(device,BULK_ENDPOINT_OUT,(u8*)&cbw,31); // Send the command 00047 if (r < 0) { 00048 printf("first transfer returns %d\n", r); 00049 return r; 00050 } 00051 if (data) { 00052 //r = USBBulkTransfer(device,flags | 1,data,transferLen); 00053 r = USBBulkTransfer(device,flags ? BULK_ENDPOINT_IN : BULK_ENDPOINT_OUT,data,transferLen); 00054 if (r < 0) { 00055 printf("second transfer returns %d (flags=%02xH)\n", r, flags); 00056 return r; 00057 } 00058 } 00059 00060 CSW csw; 00061 csw.Signature = 0; 00062 //r = USBBulkTransfer(device,0x81,(u8*)&csw,13); 00063 r = USBBulkTransfer(device,BULK_ENDPOINT_IN,(u8*)&csw,13); 00064 if (r < 0) { 00065 printf("third transfer returns %d\n", r); 00066 return r; 00067 } 00068 if (csw.Signature != CSW_SIGNATURE) 00069 return ERR_BAD_CSW_SIGNATURE; 00070 00071 // ModeSense? 00072 if (csw.Status == 1 && cmd[0] != 3) 00073 return SCSIRequestSense(device); 00074 00075 return csw.Status; 00076 } 00077 00078 int SCSITestUnitReady(int device) { 00079 u8 cmd[6]; 00080 memset(cmd,0,6); 00081 return DoSCSI(device,cmd,6,DEVICE_TO_HOST,0,0); 00082 } 00083 00084 int SCSIRequestSense(int device) { 00085 u8 cmd[6] = {0x03,0,0,0,18,0}; 00086 u8 result[18]; 00087 int r = DoSCSI(device,cmd,6,DEVICE_TO_HOST,result,18); 00088 return r; 00089 } 00090 00091 int SCSIInquiry(int device) { 00092 u8 cmd[6] = {0x12,0,0,0,36,0}; 00093 u8 result[36+2]; 00094 result[36] = '\n'; 00095 result[37] = 0; 00096 int r = DoSCSI(device,cmd,6,DEVICE_TO_HOST,result,36); 00097 if (r == 0) 00098 printf((const char*)result + 8); 00099 return r; 00100 } 00101 00102 int SCSIReadCapacity(int device, u32* blockCount, u32* blockSize) { 00103 u8 cmd[10] = {0x25,0,0,0,8,0,0,0,0,0}; 00104 u8 result[8]; 00105 *blockSize = 0; 00106 *blockCount = 0; 00107 int r = DoSCSI(device,cmd,10,DEVICE_TO_HOST,result,8); 00108 if (r == 0) { 00109 *blockCount = BE32(result); 00110 *blockSize = BE32(result+4); 00111 } 00112 return r; 00113 } 00114 00115 int SCSITransfer(int device, u32 blockAddr, u32 blockCount, u8* dst, u32 blockSize, int direction) { 00116 // USB hardware will only do 4k per transfer 00117 while (blockCount*blockSize > 4096) { 00118 int count = 4096/blockSize; 00119 int r = SCSITransfer(device,blockAddr,count,dst,blockSize,direction); 00120 dst += count*blockSize; 00121 blockAddr += count; 00122 blockCount -= count; 00123 } 00124 00125 u8 cmd[10]; 00126 memset(cmd,0,10); 00127 cmd[0] = (direction == DEVICE_TO_HOST) ? 0x28 : 0x2A; 00128 BE32(blockAddr,cmd+2); 00129 BE16(blockCount,cmd+7); 00130 return DoSCSI(device,cmd,10,direction,dst,blockSize*blockCount); 00131 } 00132 00133 int MassStorage_ReadCapacity(int device, u32* blockCount, u32* blockSize) { 00134 int result = SCSIReadCapacity(device,blockCount,blockSize); 00135 printf("MSReadCapacity(%d) = %d, blocks=%u, blksiz=%u\n", device, result, *blockCount, *blockSize); 00136 return result; 00137 } 00138 00139 int MassStorage_Read(int device, u32 blockAddr, u32 blockCount, u8* dst, u32 blockSize = 512) { 00140 int result = SCSITransfer(device,blockAddr,blockCount,dst,blockSize,DEVICE_TO_HOST); 00141 printf("MSRead(%d, %u, %u, %p, %d) = %d\n", device, blockAddr, blockCount, dst, blockSize, result); 00142 return result; 00143 } 00144 00145 int MassStorage_Write(int device, u32 blockAddr, u32 blockCount, u8* dst, u32 blockSize = 512) { 00146 int result = SCSITransfer(device,blockAddr,blockCount,dst,blockSize,HOST_TO_DEVICE); 00147 printf("MSWrite(%d, %u, %u, %p, %d) = %d\n", device, blockAddr, blockCount, dst, blockSize, result); 00148 return result; 00149 } 00150 #endif
Generated on Tue Jul 12 2022 18:48:53 by
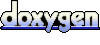